In this short JavaFX tutorial, we will explore how to create Nested Layouts. But first, what exactly is a nested layout?
We all know what nested loops are, which is when we place a loop within another loop. Similarly, a nested layout is when we plan a layout within another layout. This allows us to create very intricate and complex layout patterns for our JavaFX GUI elements.
We can nest layouts of the same type (StackPane inside a StackPane) and layouts of different types as well (StackPane inside a FlowPane). Let’s explore an example to help you get a better idea.
Placing HBox Layouts into VBox Layout
Lets imagine a scenario where we want to create a 2×2 grid of 4 Buttons, using VBox and/or HBox. VBox has the ability to display two buttons in a column, whereas HBox has the ability to display two buttons in a row. But neither has the ability to create a 2×2 grid.
With nested layouts, we can combine the functionality of these two Layouts and create a Grid-like pattern in our Window. Let’s try it out!
First we will setup our basic application, with a Scene, a Stage and a “main” layout. The “main” layout here can be either VBox or HBox. Doesn’t make much of a difference either way.
We will go with VBox as the main layout, that we create our scene with. All other layouts will be nested within this layout.
import javafx.application.Application;
import javafx.stage.Stage;
import javafx.scene.Scene;
import javafx.scene.layout.VBox;
public class Main extends Application {
public static void main(String args[]){
launch(args);
}
@Override
public void start(Stage primaryStage) throws Exception {
VBox vlayout = new VBox();
Scene scene = new Scene(vlayout);
primaryStage.setTitle("CodersLegacy");
primaryStage.setScene(scene);
primaryStage.show();
}
}
Now we will begin creating a few buttons.
Our goal is to create a 2×2 grid, where we have two rows and two buttons in each row. This can now easily be achieved, by adding two HBox layouts into our VBox layout. These two HBox layouts will automatically be stacked vertically because we are adding them into a VBox layout.
By placing buttons within the HBox layout, we can get them to be stacked horizontally. Did you understand this theory?
If not, let’s take a look at the code, along with the output. We will also color code each layout in our window. Yellow is for the VBox layout, Blue and Red are for the HBox layouts. We will also add in some spacing between the buttons, and margins between the layouts and the edge of the window.
import javafx.application.Application;
import javafx.geometry.Insets;
import javafx.stage.Stage;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.scene.layout.HBox;
import javafx.scene.layout.VBox;
public class Main extends Application {
public static void main(String args[]){
launch(args);
}
@Override
public void start(Stage primaryStage) throws Exception {
Button Button1 = new Button("Button 1");
Button Button2 = new Button("Button 2");
Button Button3 = new Button("Button 3");
Button Button4 = new Button("Button 4");
HBox row1 = new HBox(Button1, Button2);
row1.setStyle("-fx-background-color: blue");
row1.setSpacing(20);
HBox row2 = new HBox(Button3, Button4);
row2.setStyle("-fx-background-color: red");
row2.setSpacing(20);
VBox vlayout = new VBox(row1, row2);
vlayout.setStyle("-fx-background-color: yellow");
VBox.setMargin(row1, new Insets(20, 20, 20, 20));
VBox.setMargin(row2, new Insets(20, 20, 20, 20));
Scene scene = new Scene(vlayout);
primaryStage.setTitle("CodersLegacy");
primaryStage.setScene(scene);
primaryStage.show();
}
}
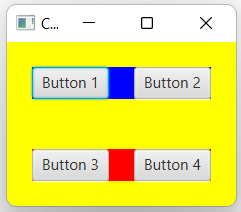
The same effect could be achieved by nested two VBox layouts into an HBox.
Hope you found this JavaFX tutorial on nested layouts helpful!
This marks the end of the Nested Layouts in JavaFX Tutorial. Any suggestions or contributions for CodersLegacy are more than welcome. Questions regarding the article content can be asked in the comments section below.