In this Python Matplotlib Tutorial, we will discuss various methods on how to read an Image File in our application.
Matplotlib imread() function
This function takes an image filepath, or image filename as a parameter. It returns the image data in the form of an array, which is compatible with matplotlib and it’s functions.
Let’s take a look at a small example, where we load an image called “quiver plot”.
import matplotlib.pyplot as plt
import numpy as np
img = plt.imread("quiverplot.png")
print(img.shape) # Prints out the image dimensions and channels
Now that you have loaded the image into the matplotlib application, you may use it in further processing, or maybe even have it displayed on the matplotlib window.
Pillow Imaging Library
There’s no real reason to use this library over the native imread()
function, unless the images you have are all stored as Pillow objects. In which case you will need to use this method to convert them to matplotlib compatible objects first.
In the below example we loaded an object using Pillow, then we converted it to a numpy array. Remember, that matplotlib also uses numpy arrays for holding image data, so they are compatbile.
import matplotlib.pyplot as plt
from PIL import Image
import numpy as np
img = Image.open("quiverplot.png")
img = np.array(img)
fig, ax = plt.subplots()
ax.imshow(img)
plt.show()
We also went ahead and displayed the image. Here is the output:
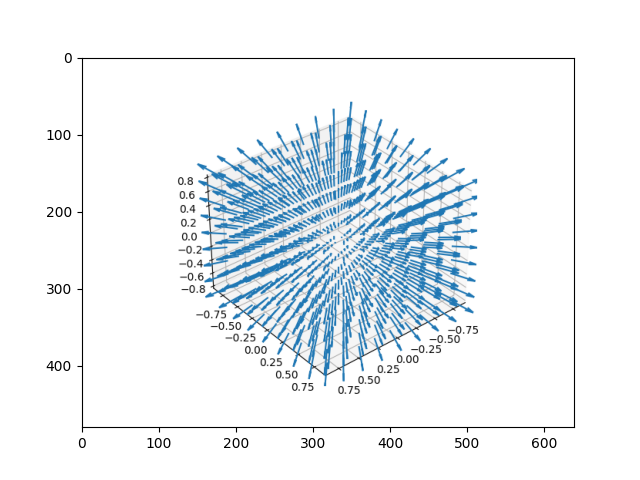
This marks the end of the How to Read an Image in Python Matplotlib Tutorial. Any suggestions or contributions for CodersLegacy are more than welcome. Questions regarding the tutorial content can be asked in the comments section below.