Creating a single Menu in Tkinter is not a big deal. But eventually we begin to wonder, how can we create a Menu within a Menu (a Submenu) in Tkinter? The answer is actually quite simple, and all we need is a single example to show you how its done.
Create a Nested Menu in Tkinter
First thing we need to do is setup a Basic Tkinter Window with a regular Menu. You should be fairly familiar with the syntax required to create a basic Menu (follow to the link to our Menu tutorial if you are new to this).
import tkinter as tk
class Window:
def __init__(self, master):
mainframe = tk.Frame(master, width=200, height=200)
mainframe.pack()
mainmenu = tk.Menu(mainframe)
master.config(menu = mainmenu)
filemenu = tk.Menu(mainframe, tearoff = 0)
filemenu.add_command(label = "New File", command=self.func)
filemenu.add_command(label = "Save", command=self.func)
filemenu.add_command(label = "Load", command=self.func)
mainmenu.add_cascade(label = "File", menu = filemenu)
def func(self):
print("This is an empty function")
root = tk.Tk()
window = Window(root)
root.mainloop()
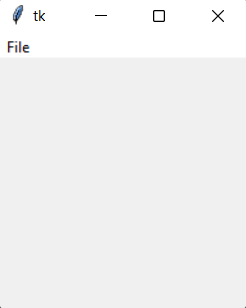
The key take-away from this code is that we created two Menus, a “Main Menu” and a “File Menu”. The main menu was inserted into the window (using config
function), and the file menu was added into the main menu (using add_cascade
function).
Now its time to add a Nested Menu, which in other words is a Menu within a Menu.
The answer to this problem is actually quite obvious. In the previous example, we already demonstrated how to add a Menu to another Menu. All we need to do is create a new Menu, which we will then add to our File Menu (using the same add_cascade
function).
In the below example, we create a new menu called “Export Menu” which then add into the File Menu.
import tkinter as tk
class Window:
def __init__(self, master):
mainframe = tk.Frame(master, width=200, height=200)
mainframe.pack()
mainmenu = tk.Menu(mainframe)
master.config(menu = mainmenu)
filemenu = tk.Menu(mainframe, tearoff = 0)
filemenu.add_command(label = "New File", command=self.func)
filemenu.add_command(label = "Save", command=self.func)
filemenu.add_command(label = "Load", command=self.func)
exportmenu = tk.Menu(mainframe, tearoff=0)
exportmenu.add_command(label="Export as .PDF", command=self.func)
exportmenu.add_command(label="Export as .JPG", command=self.func)
exportmenu.add_command(label="Export as .MP4", command=self.func)
mainmenu.add_cascade(label = "File", menu = filemenu)
filemenu.add_cascade(label = "Export", menu = exportmenu)
def func(self):
print("This is an empty function")
root = tk.Tk()
window = Window(root)
root.mainloop()
Here is our output.
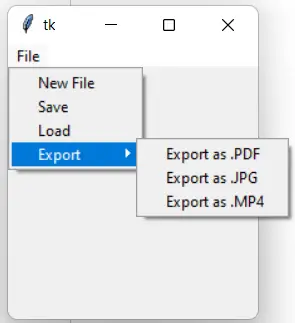
This marks the end of the How to create a Submenu in Tkinter? Tutorial. Any suggestions or contributions for CodersLegacy are more than welcome. Questions regarding the Tutorial content can be asked in the comments section below.