In this wxPython Tutorial, we will demonstrate how to use the RadioButton Widget, alongside it’s various styles, features and functions. A complete list of options will be included here, alongside several code examples for your convenience.
wxPython RadioButton Syntax
The syntax for the creation of a RadioButton Widget.
rb = RadioButton(parent, id, label, pos, size, style, validator, name)
parent
: This is the Widget to which it is parented, such as a Panel.id
: Widget ID. Default value is ID_ANY, which gives it the next available ID.label:
The Text which you want to display on the Widget.pos:
A tuple containing the coordinates of where the topleft corner of the RadioButton Widget should begin from.size:
A tuple which defines the dimensions of the area occupied by the RadioButton.style:
Used for styling the RadioButton.
wxPython RadioButton Styles and Methods
A list of available styles for the wxPython RadioButton.
Style | Description |
---|---|
wx.RB_GROUP | Explicitly creates a new group of RadioButtons. |
wx.RB_SINGLE | Creates a radio button which is not part of any radio button group |
A list of useful methods for the wxPython RadioButton.
Method | Description |
---|---|
SetLabel(string) | Takes a string as parameter as the new label of the RadioButton. |
GetLabel() | Returns a string of the current label of the RadioButton. |
SetValue(bool) | Takes a boolean as parameter as the new value of the RadioButton. |
GetValue() | Returns a string of the current value of the RadioButton. |
GetFirstInGroup() | Returns the First RadioButton of the group to which this RadioButton belongs to. |
GetLastInGroup() | Returns the Last RadioButton of the group to which this RadioButton belongs to. |
GetNextInGroup() | Returns the Next RadioButton of the group to which this RadioButton belongs to. |
GetPreviousInGroup() | Returns the Previous RadioButton of the group to which this RadioButton belongs to. |
Example Code
The first thing you need to do is create a few RadioButton Widgets, with unique labels. The first RadioButton you create should have the wx.RB_GROUP
style. All RadioButtons created after this shall be in included in this group (wxPython will implicitly create a group if you do not).
Next we bind the panel in which the RadioButtons are contained, to the EVT_RADIOBUTTON
event and RadioButtons()
function. This means that any time a RadioButton in the panel is clicked, the RadioButtons()
function will be called.
Finally we create a Label on which the currently selected RadioButton’s label will be displayed. The RadioButtons()
functions is in charge of changing text on the StaticText Widget using SetLabel().
(e.GetEventObject()
returns the object that triggered the event, in this case, the RadioButton)
import wx
class Window(wx.Frame):
def __init__(self, title):
super().__init__(parent = None, title = title, size = (400, 300))
self.panel = wx.Panel(self)
self.rb1 = wx.RadioButton(self.panel, label= "Cat", pos= (100,100), style = wx.RB_GROUP)
self.rb2 = wx.RadioButton(self.panel, label= "Dog", pos=(100, 130))
self.rb3 = wx.RadioButton(self.panel, label= "Tiger", pos=(100, 160))
self.panel.Bind(wx.EVT_RADIOBUTTON, self.RadioButtons)
self.label = wx.StaticText(self.panel, label = "You have selected: Null", pos = (100,20))
self.Centre()
self.Show()
def RadioButtons(self, e):
rb = e.GetEventObject()
self.label.SetLabelText("You have selected: " + rb.GetLabel())
app = wx.App()
window = Window("WxPython Tutorial")
app.MainLoop()
The output after running the code and selecting the “Dog” option.
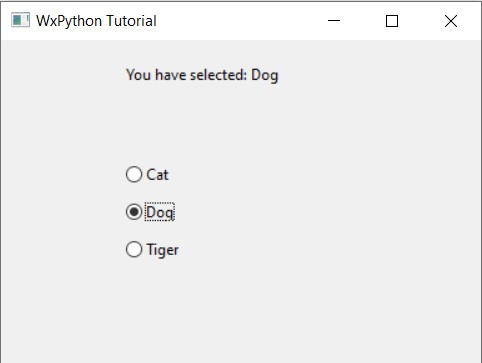
Alternatively, you can use the RadioBox Widget, which is a faster way of creating multiple RadioButtons and managing them.
This marks the end of the wxPython RadioButton Widget Tutorial. Any suggestions or contributions for CodersLegacy are more than welcome. Questions regarding the tutorial content can be asked in the comments section below.