In this Tutorial, we will discuss the Taylor Series, what it is, why we need to use it, and most importantly how to code it. We will be using Python, and the SymPy library to achieve to create an algorithm capable of producing a Taylor series for any given function.
What is the Taylor Series?
In mathematics, the Taylor series of a function is an infinite sum of terms that are expressed in terms of the function’s derivatives at a single point.
The goal of the Taylor series, is to create an estimate (guess) of what a function looks like by expanding it into an infinite set of terms. In other words, it allows you to create approximations for functions.

This enables us to achieve other things, such as expanding the domain of a function e,g. from R (set of all real numbers) to C. As well as finding the integration of a function for which there is no anti-derivative.
Let’s take a look at some graphs to strengthen our understanding of how the Taylor Series works, and what effect does adding more terms have to the graph representation of the Taylor Series.
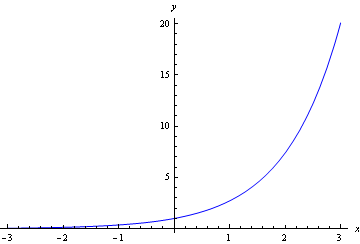
This graph here is the graph for the function ex
. We are going to try and make the Taylor series for this function in our tutorial. Before that though, let’s see what the graph of the Taylor series looks like as we add points to it.
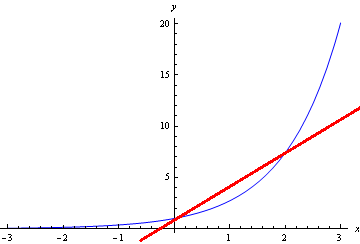
This here is what the Taylor series graph looks like when there are only two points (terms). Obviously, this is a terrible approximation, and no where near accurate. Let’s try adding another point (term).
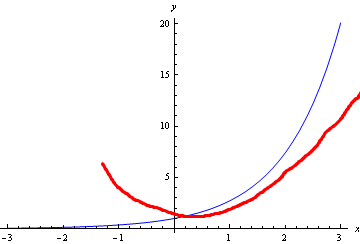
The graph has now begun to resemble a curve. This is because the third point turns it from a straight line to a curve (by adding x2). But still, this is very inaccurate.
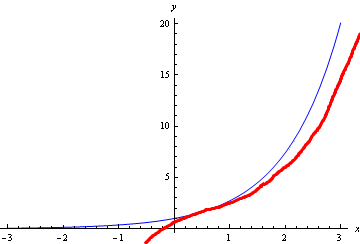
We now add a 4th point, and we can see that the graphs are beginning to look similar.
Like this, we keep adding more and more points until we end up with a Taylor series that almost perfectly resembles the graph of the original function.
Can you guess what happens when you try to create a Taylor series for a polynomial? We’ll have the answer at the end of the tutorial!
Implementing the Taylor Series in Python
It’s time to implement the Taylor Series into our Python program. Just to be clear, our goal is to create a Taylor Series representation of the function ex. Of course, our code will be able to accept any other function too, so you can try it as much as you like.

Let’s use the above formula as reference. The function “f” refers to the function we are approximating, which in this case is ex. a
is simply an unknown, into which we can pass any value, just like we would to any regular function to evaluate it’s value at that point.
As mentioned earlier, we will be using SymPy, and various math related functions that it has, like diff()
to differentiate expressions, symbols()
to create unknowns that we can use, factorial()
to calculate factorial of any number, and exp()
to represent the “e”.
from sympy import symbols, diff, pretty_print, factorial, exp
x, y = symbols("x y")
n = 10 # Number of iterations
x0 = 0 # The value of "a" or the point
func = exp(x) # The function we are approximating
result = func.subs(x, x0) # Initializing result with the first term
for i in range(1, n):
result += diff(func, x, i).subs(x, x0) * ((x - x0)**i)/(factorial(i))
# diff(func, x, i) -> This differentiates "func" w.r.t x, "i-th" times
# subs(x, x0) -> This is a method used on expressions,
# to substitute an unknown (x) with a value (x0).
pretty_print(result)
9 8 7 6 5 4 3 2
x x x x x x x x
────── + ───── + ──── + ─── + ─── + ── + ── + ── + x + 1
362880 40320 5040 720 120 24 6 2
If you are having trouble understanding any of the above code, check out our SymPy Tutorial Series, where we have covered all these functions and more in detail.
But how do we know how accurate our Taylor Series is? For this, we need to calculate the “error”, which we will do in the next section.
Evaluating Relative Error in the Taylor Series
Now that we have successfully generated a Taylor Series for our function, we need to determine the error. The error indicates show by how much our answer differs from the original. The lower the error, the more accurate our series is.
from sympy import symbols, diff, pretty_print, factorial, exp
x, y = symbols("x y")
n: int = 20
x0 = 0
func = exp(x)
result = func.subs(x, x0)
for i in range(1, n):
result += diff(func, x, i).subs(x, x0) * ((x - x0)**i)/(factorial(i))
rel_error = (func.subs(x, 3) - result.subs(x, 3))/func.subs(x, 3)
print("Error: ", float(rel_error))
pretty_print(result)
All we have done here, is add an extra line which calculates the error, and then prints it out. In the above example, we are evaluating the error at the point “3
“.
The formula for error can differ a bit, but the logic and trend of error values as iterations increase, remains the same. The formula we are using is: (func(x) - taylor_func(x)) / func(x)
.
Error: 0.8008517265285442
Error: 0.5768099188731565
Error: 0.35276811121776874
Error: 0.18473675547622792
Error: 0.08391794203130346
Error: 0.03350853530884121
Error: 0.011904503856357388
Error: 0.003802992061675957
Error: 0.0011024881301154798
9 8 7 6 5 4 3 2
x x x x x x x x
────── + ───── + ──── + ─── + ─── + ── + ── + ── + x + 1
362880 40320 5040 720 120 24 6 2
As you can see from the output, as the number of iterations increase, the error drops further and further. Hypothetically speaking, if we increased the number of iterations of “n” to infinity, the error would drop to 0.
Interested in other similar tutorials in Python? Check out our tutorial on Newton’s method!
This marks the end of the Taylor Series in Python Tutorial. Any suggestions or contributions for CodersLegacy are more than welcome. Questions regarding the tutorial content can be asked in the comments section below.