JavaFX is a popular graphical user interface (GUI) toolkit for building desktop and mobile applications in Java. One of the most common UI elements in modern applications is the context menu, which is a pop-up menu that appears when the user right-clicks on a UI element. In this article, we’ll explore how to use the JavaFX ContextMenu Class to create and customize context menus in your JavaFX application.
Creating a Basic Context Menu
Here is some basic code for our JavaFX application. We have a nice little TextArea widget, which is serving as a text editor in our application. We also included a screenshot of the current output.
package application;
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.control.TextArea;
import javafx.scene.layout.StackPane;
import javafx.stage.Stage;
public class Tutorial extends Application {
@Override
public void start(Stage primaryStage) {
TextArea textArea = new TextArea();
textArea.setPrefSize(400, 300);
Scene scene = new Scene(new StackPane(textArea), 400, 300);
primaryStage.setScene(scene);
primaryStage.show();
}
public static void main(String[] args) {
launch(args);
}
}
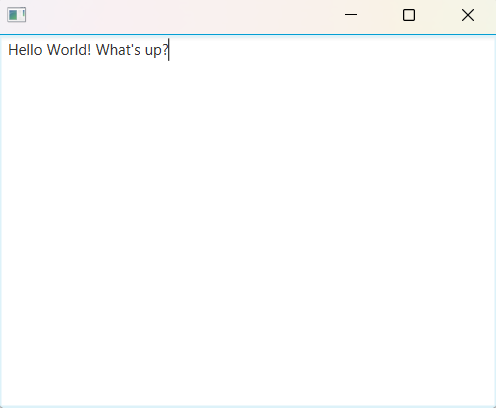
As you can see, we currently haven’t added any code for the ContextMenu. We picked a text editor as an example, because every Text editor has a ContextMenu. That’s what we will do today. Add a context menu into our application for basic features like “copy” and “paste”.
Let’s begin.
Here is the code for implementing a basic context menu (GUI only). We have made two new imports, one for the ContextMenu Class, and one for the MenuItem Class.
import javafx.scene.control.ContextMenu; // new
import javafx.scene.control.MenuItem; // new
We then created the ContextMenu.
ContextMenu contextMenu = new ContextMenu();
Then we created some Menu Items for our Context Menu.
MenuItem menuItem1 = new MenuItem("Copy");
MenuItem menuItem2 = new MenuItem("Paste");
We then added these menu items into our context menu.
contextMenu.getItems().add(menuItem1);
contextMenu.getItems().add(menuItem2);
Finally, using the setContextMenu()
method, we added the context menu we created to the TextArea widget.
textArea.setContextMenu(contextMenu);
We can now activate the context menu by right clicking anywhere on the screen.
Here is the complete code, with a screenshot of the context menu.
package application;
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.control.ContextMenu; // new
import javafx.scene.control.MenuItem; // new
import javafx.scene.control.TextArea;
import javafx.scene.layout.StackPane;
import javafx.stage.Stage;
public class Tutorial extends Application {
@Override
public void start(Stage primaryStage) {
TextArea textArea = new TextArea();
textArea.setPrefSize(400, 300);
// Create a context menu
ContextMenu contextMenu = new ContextMenu();
// Create some menu items
MenuItem menuItem1 = new MenuItem("Copy");
MenuItem menuItem2 = new MenuItem("Paste");
// Add the Menu Items to the Context Menu
contextMenu.getItems().add(menuItem1);
contextMenu.getItems().add(menuItem2);
// Set the context menu on the text area
textArea.setContextMenu(contextMenu);
Scene scene = new Scene(new StackPane(textArea), 400, 300);
primaryStage.setScene(scene);
primaryStage.show();
}
public static void main(String[] args) {
launch(args);
}
}
Here is the current output.
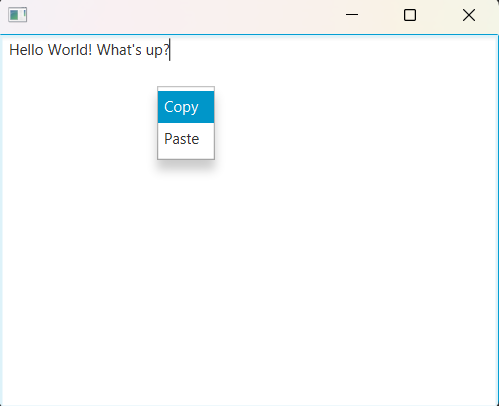
It is worth mentioning, that currently none of these menu items (copy and paste) actually do anything. We have only created the GUI so far. We will address this further in the next section.
Connecting the ContextMenu items to Functions
Now that we have created our basic ContextMenu with Menu Items, we need to connect them to their respective functions. In our case, we will connect the “Copy” and “Paste” functions to their respective menu items.
Here’s how we do it. First, we need to add an EventHandler to each of our Menu Items. This is done using the setOnAction()
method.
menuItem1.setOnAction(e -> {
textArea.copy();
});
menuItem2.setOnAction(e -> {
textArea.paste();
});
In this case, we are using lambda expressions to define the actions that need to be taken when the menu items are clicked. Lambda expressions are a shorthand notation for defining anonymous inner classes that implement a single method. They are particularly useful when defining simple event handlers like these.
Now when the “Copy” or “Paste” option is clicked in the context menu, it will perform the appropriate function on the text in the TextArea.
Customizing the JavaFX ContextMenu
The JavaFX ContextMenu can be customized in a variety of ways to meet your specific requirements. For instance, you can add images to your menu items, change the font size and style, and even change the color of the menu items.
To add an image to a menu item, you can create a new ImageView object and set it as the graphic for the menu item. Here’s an example.
import javafx.scene.image.Image;
import javafx.scene.image.ImageView;
// Place code inside the Class
MenuItem menuItem3 = new MenuItem("Cut");
Image cutImage = new Image("cut.png");
ImageView cutImageView = new ImageView(cutImage);
menuItem3.setGraphic(cutImageView);
This code creates a new MenuItem called “Cut” and sets an image called “cut.png” as its graphic. You can use any image file that you like. Just make sure that it is in a format that is supported by JavaFX (e.g. PNG, JPEG, BMP, etc.).
Remember to make those two imports at the top for the Image Classes.
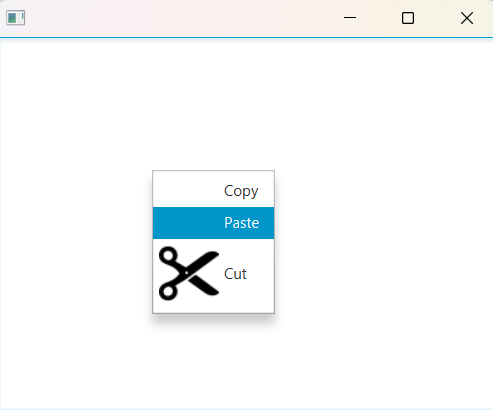
Looks good right? It could be a bit smaller, but it definitely improves the look of the application.
To change the font size and style of the menu items, you can use CSS styles. Here’s an example:
ContextMenu contextMenu = new ContextMenu();
contextMenu.setStyle("-fx-font-size: 14px; -fx-font-family: Arial;");
Output:
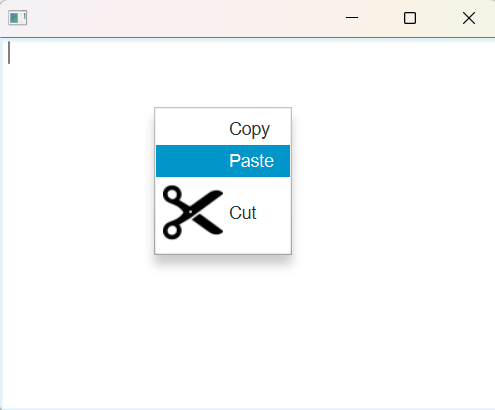
This marks the end of the Javafx ContextMenu Tutorial. Any suggestions or contributions for CodersLegacy are more than welcome. Questions about the tutorial content can be asked in the comments section below.