In any software application, user experience (UX) is critical. One key aspect of UX is providing users with clear and concise information about various elements within the application. JavaFX ToolTips are an effective way to enhance UX by providing text popups with additional information about a specific element when the user hovers over it with their mouse. In this article, we’ll explore JavaFX ToolTips and how they can improve UX in your JavaFX application.
What are JavaFX ToolTips?
JavaFX ToolTips are a type of pop-up window that displays additional information when the user hovers over a specific element, such as a button or text field. ToolTips are a way to provide users with more information about an element without cluttering the main user interface (UI) with additional text or graphics. ToolTips can also be used to provide users with helpful hints, such as keyboard shortcuts or information about what a particular feature does.
Creating a Basic ToolTip
Creating a basic ToolTip in JavaFX is straightforward. First, you need to create the UI element that you want the ToolTip to appear on, such as a button or text field. Once you have created the UI element, you can add a ToolTip by calling the setTooltip() method on the element and passing in a new Tooltip object.
Here’s an example of how to create a basic ToolTip for a button. First we are going to create the basic application structure, and the button widget.
package application;
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.scene.control.Tooltip;
import javafx.scene.layout.StackPane;
import javafx.stage.Stage;
public class Tutorial extends Application {
@Override
public void start(Stage primaryStage) throws Exception {
Button button = new Button("Click me");
StackPane root = new StackPane();
root.getChildren().add(button);
Scene scene = new Scene(root, 300, 250);
primaryStage.setTitle("Basic Tooltip Example");
primaryStage.setScene(scene);
primaryStage.show();
}
public static void main(String[] args) {
launch(args);
}
}
Next, we will add in the code for the tooltip. You might have already noticed, that in the above code we made an import for the Tooltip class. Now it’s time to use it.
All you have to do is create a Tooltip object, then pass it into the setToolTip
method of the widget you want that tooltip to appear on. Here is an example:
Tooltip tooltip = new Tooltip("This is a button");
button.setTooltip(tooltip);
Here is the output:
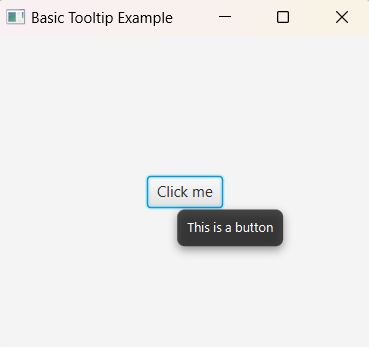
Customizing ToolTips
While creating a basic ToolTip is easy, there are several ways to customize ToolTips to better suit your application’s needs. One way to customize ToolTips is to change the style of the ToolTip itself. JavaFX provides a CSS-based API that allows you to customize the appearance of various UI elements, including ToolTips.
Here’s an example of how to customize the style of a ToolTip (using FX styles).
.tooltip {
-fx-background-color: #FFFFFF;
-fx-border-color: #000000;
-fx-font-family: "Arial";
-fx-font-size: 14px;
-fx-padding: 5px;
-fx-text-fill: #000000; /* set the font color to black */
}
Normally we place these in a separate file. If you already know how to do this, go ahead and do so. We will take a shortcut, and add it directly into our code.
Tooltip tooltip = new Tooltip("This is a button");
tooltip.setStyle("-fx-background-color: #FFFFFF; " +
"-fx-text-fill: #000000; " +
"-fx-border-color: #000000; " +
"-fx-font-family: 'Arial'; " +
"-fx-font-size: 14px; " +
"-fx-padding: 5px;");
button.setTooltip(tooltip);
Here is the output (we flipped the colors).
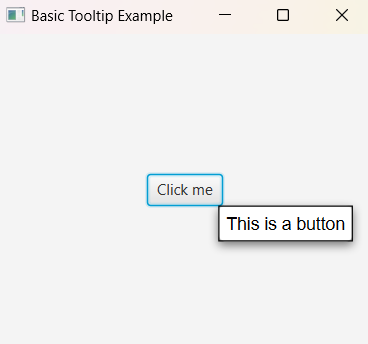
Cool right?
Anyways, there is one more important customization, that you can’t do using the FX styles. Lets talk about that real quick.
Customizing Delay in JavaFX Tooltips
Another way to customize ToolTips is to add a delay before the ToolTip appears. This can be useful if you have a UI element that the user might accidentally hover over and you don’t want the ToolTip to appear immediately.
Here’s an example of how to add a delay to a ToolTip.
import java.time.Duration;
Tooltip tooltip = new Tooltip("This is a button");
tooltip.setShowDelay(Duration.seconds(3));
button.setTooltip(tooltip);
Make sure you to make that extra import for “Duration”.
You can also customize the duration that a ToolTip remains visible after the user moves their mouse away from the UI element. The method for doing so is actually pretty similar to the one we just discussed.
Here it is.
Tooltip tooltip = new Tooltip("This is a button");
// set the duration to 5 seconds
tooltip.setShowDuration(Duration.seconds(5));
button.setTooltip(tooltip);
The above code sets the tooltip to only show for 5 seconds, after which it will go away.
This marks the end of the JavaFX Tooltips tutorial. Any suggestions or contributions for CodersLegacy are more than welcome. Questions regarding the tutorial content can be asked in the comments section below.