Tkinter is a popular Python library for GUI development, and the ttk module provides advanced widgets for Tkinter. One such widget is the ttk Notebook widget, which can be used to create tabbed interfaces in Tkinter applications. In some cases, you might want to hide the tab(s) in the Tkinter Notebook widget to provide a simpler and more streamlined look to your application.
There are two ways that we can do this, each with its own benefits.
Method 1: Hiding all Notebook tabs using Styles
The first method involves disabling the ttk styles for the Notebook tabs, thus making them disappear from the window.
Here is the code (explanation given below).
import tkinter as tk
from tkinter import ttk
class Window:
def __init__(self):
self.notebook = ttk.Notebook(root, width = 400, height = 200)
frame = tk.Frame(root)
frame.pack(fill=tk.X)
self.first = ttk.Frame(root)
self.second = ttk.Frame(root)
self.third = ttk.Frame(root)
self.fourth = ttk.Frame(root)
self.fifth = ttk.Frame(root)
self.notebook.add(self.first, text="Notebook Tab 1")
self.notebook.add(self.second, text="Notebook Tab 2")
self.notebook.add(self.third, text="Notebook Tab 3")
self.notebook.add(self.fourth, text="Notebook Tab 4")
self.notebook.add(self.fifth, text="Notebook Tab 5")
self.notebook.pack()
root = tk.Tk()
style = ttk.Style()
style.layout("TNotebook.Tab", [])
window = Window()
root.mainloop()
In the code provided above, the Window class creates a Notebook widget with 5 tabs. The tabs are created as ttk Frame widgets and added to the Notebook widget using the add()
method.
To hide the tabs, we create an instance of the ttk Style class and use the layout()
method to define a custom style layout for the TNotebook.Tab element. In this case, we pass an empty list to the layout()
method, which means that no elements will be displayed for this element.
Here is the output of the above code. If you look closely, you can see the outline of the notebook around the edges of the window, but the tabs are completely gone.
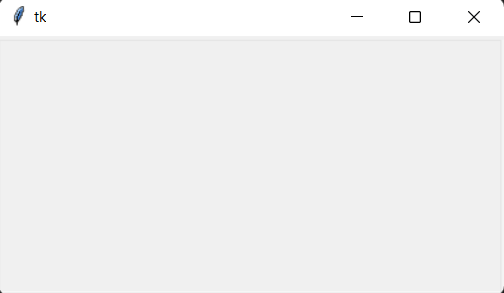
It is worth mentioning that disabling the ttk styles for the Notebook will be a global change. If you want to only effect a certain notebook, then do the following:
style.layout("Tabless.TNotebook.Tab", [])
Instead of the modifying the default styles for the Notebook tabs, we created a new one called “Tabless.TNotebook.Tab”. Now all we have to do is assign this new style to the Notebook whose tabs we want to hide.
Like this:
self.notebook = ttk.Notebook(root, width = 400, height = 200, style="Tabless.TNotebook")
You might be wondering how to change tabs if the tabs are now invisible. You can use the notebook.select()
method, which takes a frame object or an index number as a parameter. It will then switch to the tab which corresponds to that frame or index number.
Method 2: Hiding some or all Notebook tabs using hide()
The second method is a little more complicated, but gives us more control over how we hide tabs. We have developed a useful little application here, where we have limited the number of visible tabs to 3. We have also created “back” and “forward” buttons which allow us to cycle through the tabs.
The main trick here, is the use of the hide()
method. By passing in either a frame object, or index number to this method, we can hide the Notebook tab corresponding to that object/index. Likewise, using the select()
method will unhide the tab.
import tkinter as tk
from tkinter import ttk
class Window:
def __init__(self):
self.notebook = ttk.Notebook(root, width = 400, height = 200)
frame = tk.Frame(root)
frame.pack(fill=tk.X)
tk.Button(frame, text = "Back", command=self.back).pack(side=tk.LEFT)
tk.Button(frame, text = "Forward", command=self.forward).pack(side=tk.RIGHT)
self.first = ttk.Frame(root)
self.second = ttk.Frame(root)
self.third = ttk.Frame(root)
self.fourth = ttk.Frame(root)
self.fifth = ttk.Frame(root)
self.notebook.add(self.first, text="Notebook Tab 1")
self.notebook.add(self.second, text="Notebook Tab 2")
self.notebook.add(self.third, text="Notebook Tab 3")
self.notebook.add(self.fourth, text="Notebook Tab 4")
self.notebook.add(self.fifth, text="Notebook Tab 5")
self.notebook.hide(self.fourth)
self.notebook.hide(self.fifth)
self.notebook.pack()
def back(self):
index = self.notebook.index(self.notebook.select())
if index >= 3 and index <= 4:
self.notebook.select(index - 1)
elif index < 3 and index > 0:
self.notebook.hide(index + 2)
self.notebook.select(index - 1)
def forward(self):
index = self.notebook.index(self.notebook.select())
if index < 4:
self.notebook.select(index + 1)
if index >= 2 and index < 4:
self.notebook.hide(index - 2)
root = tk.Tk()
window = Window()
root.mainloop()
A snapshot of our application using the above code.
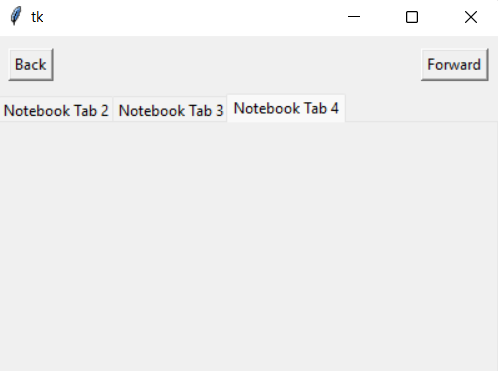
This marks the end of the How to hide Tkinter (ttk) Notebook Tab(s) Tutorial. Any suggestions or contributions for CodersLegacy are more than welcome. Questions regarding the tutorial content can be asked in the comments section below.