In this PyQt6 tutorial we will explore how to create a “Context Menu”. A context menu refers to the popup window that shows whenever you do a right click on a window.
If you are reading this on a desktop browser (regardless of which browser it is), do a right click with your mouse or touch pad. The menu that you see in front of you is called a Context Menu, which typically presents you with a list of options or commands.
Creating a Context Menu in PyQt6
Here is a code example where we create a simple Context Menu in PyQt6.
from PyQt6.QtWidgets import QWidget, QMenu, QApplication
import sys
class MyWindow(QWidget):
def __init__(self):
super().__init__()
# Create the context menu and add some actions
self.context_menu = QMenu(self)
action1 = self.context_menu.addAction("Action 1")
action2 = self.context_menu.addAction("Action 2")
action3 = self.context_menu.addAction("Action 3")
# Connect the actions to methods
action1.triggered.connect(self.action1_triggered)
action2.triggered.connect(self.action2_triggered)
action3.triggered.connect(self.action3_triggered)
self.show()
def contextMenuEvent(self, event):
# Show the context menu
self.context_menu.exec(event.globalPos())
def action1_triggered(self):
# Handle the "Action 1" action
pass
def action2_triggered(self):
# Handle the "Action 2" action
pass
def action3_triggered(self):
# Handle the "Action 3" action
pass
app = QApplication(sys.argv)
window = MyWindow()
sys.exit(app.exec())
Here is the output.
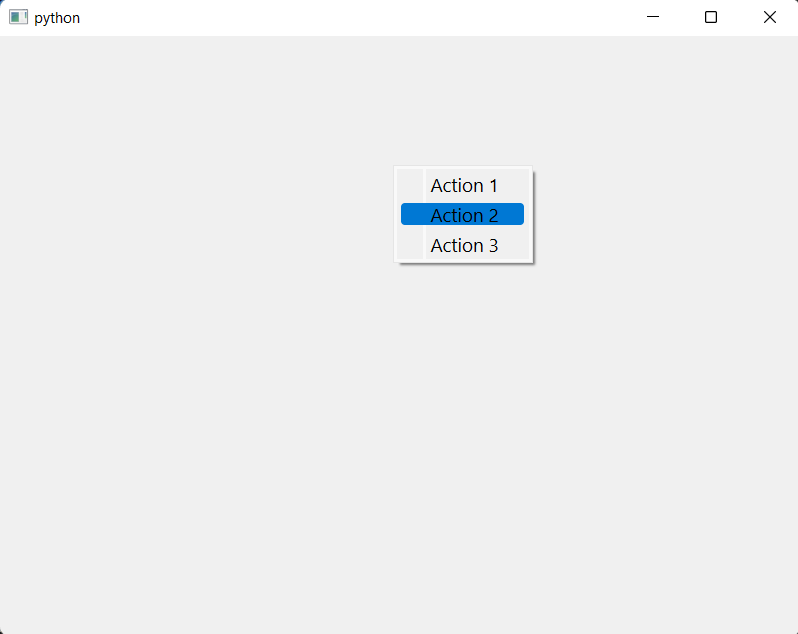
Now lets discuss some of the important code snippets from our earlier code in greater detail.
First we created a basic Menu using the QMenu Class. This follows the same process that you would do for creating a regular Menu for your Window.
# Create the context menu and add some actions
self.context_menu = QMenu(self)
action1 = self.context_menu.addAction("Action 1")
action2 = self.context_menu.addAction("Action 2")
action3 = self.context_menu.addAction("Action 3")
We can add various options/items in our Menu using the .addAction()
method as shown above. These options that we have added are just for show however. They will not actually do anything when you click them.
To trigger a function whenever one of these “Actions” are pressed, we will use the Signals and Slots system in PyQt.
# Connect the actions to methods
action1.triggered.connect(self.action1_triggered)
action2.triggered.connect(self.action2_triggered)
action3.triggered.connect(self.action3_triggered)
Basically we use the connect()
method, which takes a function name as a parameter. Whenever the appropriate event occurs (i.e. The action item is clicked), the function will be called.
This last step is very crucial. So far we have just been creating a regular Menu. The last step is what makes our Regular menu into a Context menu.
We will define a function called contextMenuEvent
. This exact name is important, because this is actually a function that we are overriding (from a parent class). Changing the name in any way will cause our code to stop working.
def contextMenuEvent(self, event):
# Show the context menu
self.context_menu.exec(event.globalPos())
What we are doing here, is calling the exec()
method, which takes the coordinates of where the event occurred (the event is the mouse click). It will automatically spawn our Menu at that location.
(PyQt is responsible for calling the contextMenuEvent function, not us, which is why it’s critical not to change the name).
This marks the end of the PyQt6 Context Menu Tutorial. Any suggestions or contributions for CodersLegacy are more than welcome. Questions regarding the tutorial content can be asked in the comments section below.
thanks.
it was helpful for me.
and, I am going to make the action of context menu action.
in other words, when click the cell in tablewidget, how can I make the clipboard action?
would you help me?
I will be appreciated, if you reply to below email.
Best regards.
thanks.
it was helpful for me.
and, I am going to make the action of context menu action.
in other words, when click the cell in tablewidget, how can I make the clipboard action?
would you help me?
I will be appreciated, if you reply to below email.
Best regards.
[email protected]