In this Tkinter tutorial we will explore how to create a “Context Menu”. A context menu refers to the popup window that shows whenever you do a right click on a window.
If you are reading this on a desktop browser (regardless of which browser it is), do a right click with your mouse or touch pad. The menu that you see in front of you is called a Context Menu, which typically presents you with a list of options or commands.
Creating a Context Menu in Tkinter
To create a context menu in Tkinter, we will be using the tkinter.Menu
class. This class provides a convenient way to create and manage a context menu in your Tkinter application (as well as creating regular menus for your window)
Here is an example of how you could create a context menu with the Menu
class (triggered by right clicking the window).
import tkinter as tk
# Create the main window
root = tk.Tk()
# Create a context menu
context_menu = tk.Menu(root, tearoff=0)
def handleClick(event):
print("Hello World")
# Add some options to the context menu
context_menu.add_command(label="Option 1", command=handleClick)
context_menu.add_command(label="Option 2", command=handleClick)
context_menu.add_separator()
context_menu.add_command(label="Quit", command=root.quit)
# Bind the context menu to the root window
root.bind("<Button-3>", lambda event: context_menu.post(event.x_root, event.y_root))
# Start the main event loop
root.mainloop()
Our output (after performing a right click)
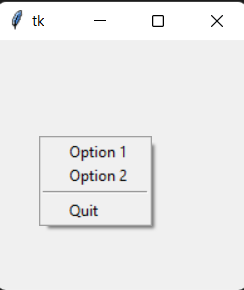
Most of the code here is fairly simple and easy to understand. Let’s take a closer look at some of the important lines which made the above output possible.
Here we created the Menu itself, and added a few “commands” or “options” to it. Each command can be linked back to the custom function that we define.
context_menu = tk.Menu(root, tearoff=0)
context_menu.add_command(label="Option 1", command=handleClick)
context_menu.add_command(label="Option 2", command=handleClick)
context_menu.add_separator()
context_menu.add_command(label="Quit", command=root.quit)
Normally each option would have its own function, but this is just an example.
This line here is responsible for two things. First, it binds the Right Click button to a function that we define in the second parameter. This line is also responsible for actually defining the function that will be called (by using a lambda function).
# Bind the context menu to the root window
root.bind("<Button-3>", lambda event: context_menu.post(event.x_root, event.y_root))
We can also break this down a bit to make it easier to explain.
def handleRightClick(event):
context_menu.post(event.x_root, event.y_root)
root.bind("<Button-3>", handleRightClick)
This code snippet actually does the same thing as the one from earlier. This is the non-lambda approach. We get an event object, which contains information about the x and y locations of where the “click” was made. We then call the post()
method that places our menu in that location.
There is an alternative function that can be used, which actually does the exact same thing, called tk_popup()
.
def handleRightClick(event):
context_menu.tk_popup(event.x_root, event.y_root)
root.bind("<Button-3>", handleRightClick)
Binding Context Menu’s to specific widgets
You might not have realized this, but the bind()
method can be used on any widget, not just “root”. What this also means, is that we can bind the Context Menu we created to even a button or label widget.
An interesting way of using this, would be if you have multiple frames in your window. Binding different Context Menu’s to each frame, would result in a different one being shown depending on which part of the window you clicked on.
This marks the end of the Tkinter Context Menu Tutorial. Any suggestions or contributions for CodersLegacy are more than welcome. Questions regarding the tutorial content can be asked in the comments section below.