In this Python Tutorial, we will explain how to perform the Union operation between multiple Sets. Union is one of many various Set operations available to us, which we can use on Python sets.
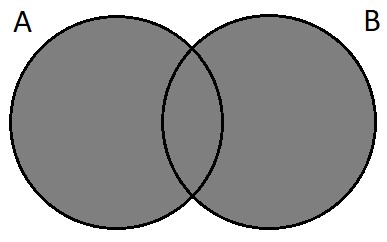
Union of Two Sets
Union of two sets refers to the elements of both Set A and Set B. Basically a Set containing all values from Set A and Set B, but without any duplicates. (Like if Set A and Set B have a common value, it will only appear once in the Union set)
Union Operator
To perform the Union Set Operation, we can use the | operator between two Sets. (also known as the pipe operator)
set1 = { 1, 2, 3 }
set2 = { 2, 3, 4, 5, 6 }
set3 = { "a", "b", "c" }
print(set1 | set2)
print(set1 | set3)
{1, 2, 3, 4, 5, 6}
{1, 2, 3, 'c', 'a', 'b'}
Union Function
Alternatively, the union function can also be used on the sets, which produces the same result.
set1 = { 1, 2, 3 }
set2 = { 2, 3, 4, 5, 6 }
set3 = { "a", "b", "c" }
print(set1.union(set2))
print(set1.union(set3))
{1, 2, 3, 4, 5, 6}
{1, 2, 3, 'a', 'b', 'c'}
Union on multiple Sets
Of course, there is nothing stopping us from performing Union on more than just 2 sets. There is no real limit to how many Sets we can perform Union on at the same time.
Let’s take a look at a short example illustrating this.
set1 = { 1, 2, 3 }
set2 = { 4, 5, 6 }
set3 = set() # Creates an empty set
set4 = { 'a', 'b', 'c '}
print(set1 | set2 | set3 | set4)
{1, 2, 3, 4, 5, 6, 'c ', 'b', 'a'}
This marks the end of the Union of Sets in Python Tutorial. Any suggestions or contributions for CodersLegacy are more than welcome. Questions regarding the tutorial content can be asked in the comments section below.