In this tutorial we will explore how to create a Shapely Polygon from a Python Pandas Dataframe. To create a Shapely Polygon, we need a coordinate array containing the x
and y
value pairs. In order to extract this data from our Pandas Dataframe, we need to perform a series of operations first.
Creating a Pandas Dataframe
First we need to set up our imports and create a Pandas Dataframe with our coordinates.
from shapely.geometry import Polygon
import pandas as pd
import numpy as np
df = pd.DataFrame({
'x': [10, 50, 50, 30, 10, 10,],
'y': [10, 10, 50, 80, 50, 10,],
})
Pandas Dataframes usually store values in the above format, where there are two columns. One for the X-axis and one for the Y-axis. For shapely we need coordinates pairs (tuples with a x and y value), not two lists as shown in the Dataframe.
How can we accomplish this?
Creating Shapely Polygon with Pandas
To convert the Pandas Dataframe, we will make use of the map()
function. This can be used to map our dataframe to a pair of “n” tuples, where “n” is the number of values in each column. The number of values in each table is equal to the number of columns (two in this case).
result = map(tuple, np.asarray(df))
However, we still need to perform one more operation. The map()
returns a map object. What we need is a list. So we will wrap the whole thing into a list()
function.
The complete code along with the output is shown below.
from shapely.geometry import Polygon
import pandas as pd
import numpy as np
df = pd.DataFrame({
'x': [10, 50, 50, 30, 10, 10,],
'y': [10, 10, 50, 80, 50, 10,],
})
coords = list(map(tuple, np.asarray(df)))
poly = Polygon(coords)
print(poly)
POLYGON ((10 10, 50 10, 50 50, 30 80, 10 50, 10 10))
We can also use Matplotlib to visualize our Shapely Polygon by plotting its coordinates to our Matplotlib window as shown below. This also helps us verify that our Polygon was created successfully.
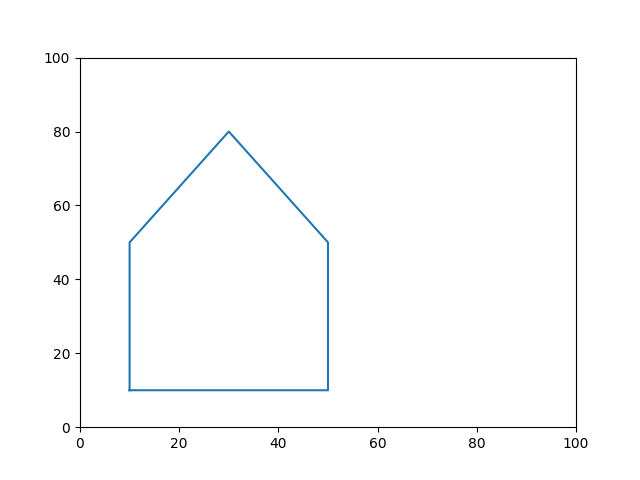
Shapely Polygons are actually quite compatible, and easily plottable and customizable with Matplotlib. Refer to this link for more on this topic.
This marks the end of the Create Shapely polygon from Pandas Dataframe Tutorial. Any suggestions or contributions for CodersLegacy are more than welcome. Questions regarding the tutorial content can be asked in the comments section below.