Matplotlib can produce some pretty cool visualizations of data in the form of graphs (e.g Histograms, Bar charts and Pie-charts). Uptil now you have been probably been using screen-shots to share or use these graphs in other areas. In this tutorial we will teach you how to directly save Matplotlib Graphs as JPG Images instead!
This allows for a better image quality and a completely preserved aspect ratio when saving graphs. (Not to mention that if you are building an application, you want to give the user an option to export his graphs).
Converting Matplotlib Graphs to JPG Images
To demonstrate how we can convert a Graph into a JPG image, we will be using the following code. It’s a simple Matplotlib program that generates a Sin graph, and also features some special text annotation.
import matplotlib.pyplot as plt
import numpy as np
fig, ax = plt.subplots()
ax.set_ylim(-2, 2)
x = np.arange(0.0, 5.0, 0.01)
y = np.sin(2*np.pi * x)
ax.annotate('Maximum Point',
xy = (3.3, 1),
xytext = (3.5, 1.8),
arrowprops= dict(facecolor = "red"))
plt.plot(x, y)
plt.show()
Saving this as an image is actually very easy! All we have to do is add a single function call to plt.savefig()
using a filename with the correct jpg extension!
plt.savefig('myJPG.jpg', dpi = 100)
Instead of a filename, you can also choose to pass in a filepath. You can also change the DPI settings (you don’t have to specify this as matplotlib will use the default instead) if you want to change the resolution/size of the image.
Place this function call between the plot()
and show()
functions, and we are good to go!
plt.plot(x, y)
plt.savefig('myJPG.jpg', dpi = 100)
plt.show()
Here is the output image file produced:
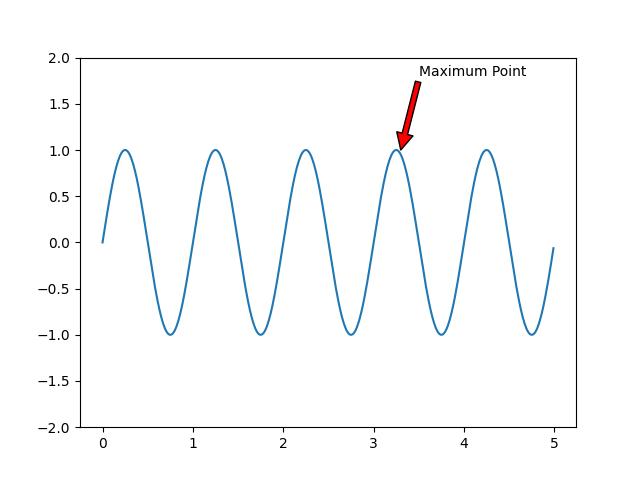
Benefits of using JPG: The main benefit of using JPG over other file types is the space requirements. JPG’s use advanced techniques which reduce the colors and compress the image in such a way that it is not noticeable to the human eye. This can result in a reduction of about 50% (varies on the image being used and the source resolution, could be more, could be less) over a higher quality format like PNG.
Common Mistakes
Placing the plt.show()
function call before savefig()
will cause an empty graph to be saved. plt.show()
is a blocking function call that will only allow savefig()
to execute once the Matplotlib Window is closed. The problem is that closing the Matplotlib window will also clear the graph, which results in an empty image.
TLDR: Place the savefig()
function before plt.show()
.
This marks the end of the How to save Matplotlib graphs as JPG images Tutorial. Any suggestions or contributions for CodersLegacy are more than welcome. Questions regarding the tutorial content can be asked in the comments section below.