In this tutorial we will explore how to create a Table using the Treeview Widget in Tkinter. The Treeview widget is a versatile widget used to display hierarchical data in a tabular format. It allows for the creation of columns with headings and supports features such as sorting, selection, and editing of data entries. This makes it the perfect candidate for constructing a Table.
Setting up the Table Widget
We will start by writing out the boilerplate code for our application. The following code features:
- A class called “Table” which stores the data passed to it, as
self.data
. - A list of random product data.
- Tkinter imports + application setup.
import tkinter as tk
from tkinter import ttk
class Table(tk.Tk):
def __init__(self, data, **kwargs):
super().__init__(**kwargs)
self.data = data
products = [("1001", "Product A", "Category 1", "$10.99"),
("1002", "Product B", "Category 2", "$15.49"),
("1003", "Product C", "Category 1", "$8.95"),
("1004", "Product D", "Category 3", "$12.99"),
("1005", "Product E", "Category 2", "$9.99"),
("1006", "Product F", "Category 1", "$7.50"),
("1007", "Product G", "Category 3", "$11.25"),
("1008", "Product H", "Category 2", "$14.99"),
("1009", "Product I", "Category 1", "$6.99"),
("1010", "Product J", "Category 3", "$10.00")]
app = ProductTable(products)
app.mainloop()
Now we will focus on writing the initialization logic for Treeview widget in the __init__
method. First, we will create a Treeview object, using the Treeview class. We will also pass the list of column names into this widget.
self.tree = ttk.Treeview(self, columns=["ID", "Name", "Category", "Price"], show="headings")
Next, we define the Table headings using the heading
method.
“Headings” is not the same as “Columns” in the Treeview widget. You can think of column names as internal values used for identifying columns, whereas the heading name is the visual representation of the column name, that appears as text at the top of the table when the Treeview widget is created. Each heading must correspond to a column name (which is the first parameter) The text
parameter in the heading
method controls what text is displayed in the Treeview widget.
self.tree.heading("ID", text="ID")
self.tree.heading("Name", text="Name")
self.tree.heading("Category", text="Category")
self.tree.heading("Price", text="Price")
Now to insert the product data into the Treeview widget (insert
method).
for row in self.data:
self.tree.insert("", tk.END, values=row)
Don’t forget to pack the widget in.
self.tree.pack(fill="both", expand=True)
This gives us the following table.
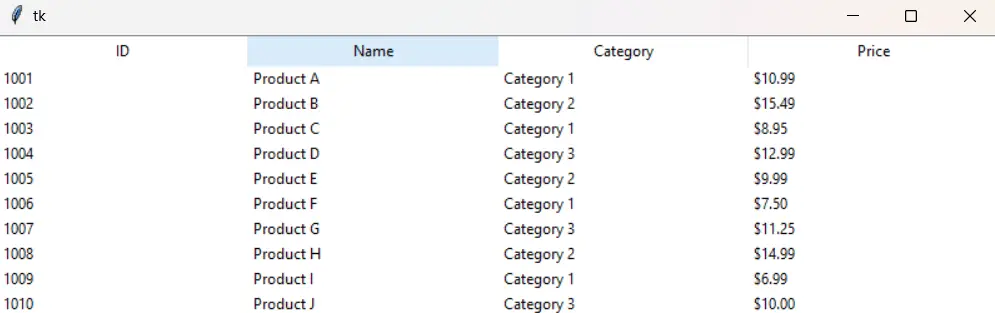
Adding Helper Functions
We will now create two “Helper” functions which add on to the functionality of our table, and make it more convenient to use.
The get_column
method helps us retrieve all the values from a specific column in our Treeview widget. For instance, if we want to get all the product names from the “Name” column, we can use this method.
def get_column(self, column_name):
column_values = []
for id in self.tree.get_children():
column_values.append(self.tree.item(id, "values")[self.tree["columns"].index(column_name)])
return column_values
- First, we create an empty list called
column_values
. This list will hold all the values from the specified column. - We then loop through each item (or row) in our Treeview, using the list of ids returned by the
get_children
method. - For each item, we use
self.tree.item(id, "values")
to get all the values associated with that item. - Now comes a bit of a tricky part. We need to find the index of our desired column in the list of columns defined for our Treeview. We do this using
self.tree["columns"].index(column_name)
. Once we have the index, we can use it to access the value from the corresponding column for the current item. - We append this value to our
column_values
list. - After looping through all items, we return
column_values
, which now contains all the values from the specified column.
The get_row
method is for retrieving all the values from a specific row in our Treeview widget. For example, if we want to get all the details of the third product listed in our table, we can use this method.
def get_row(self, row_index):
print("get_children return value: ", self.tree.get_children())
return self.tree.item(self.tree.get_children()[row_index], "values")
- We first use
self.tree.get_children()
to get all the IDs of the rows in our Treeview. - To access a specific row, we use
self.tree.get_children()[row_index]
and pass in the index of the row we want. - We then use
self.tree.item(..., "values")
to get all the values associated with that row. The...
here represents the ID of the row we obtained earlier. - We return these values, which are now in the form of a tuple, representing all the details of the specified row.
This marks the end of the Tkinter Table Widget using Treeview Tutorial. Any suggestions or contributions for CodersLegacy are more than welcome. Questions regarding the tutorial content can be asked in the comments section below.
1 thought on “Tkinter Table Widget using Treeview (Python)”