In this tutorial, we will learn how to create a status bar in Tkinter, the built-in Python GUI framework. A status bar is a small area at the bottom of a window that provides feedback about the current state of the application, such as the progress of a task or the status of an operation.
Tkinter Status Bar Tutorial
Our approach in this tutorial, will be to create an actual Class for the Status Bar, that we can re-use as many times as we wish. Creating it directly in our window would actually be easier (in the short term), but the Class based approach is far better for a proper application.
The StatusBar Class is actually pretty small. We make our StatusBar class inherit the Frame widget, and define a single label inside it.
import tkinter as tk
class StatusBar(tk.Frame):
def __init__(self, master):
tk.Frame.__init__(self, master)
self.label = tk.Label(self)
self.label.pack(side=tk.LEFT)
self.pack(side=tk.BOTTOM, fill=tk.X)
Special attention needs to be given to the options inside the pack() methods. We want our statusbar to be at the very bottom, hence the frame has the option side=tk.BOTTOM
, and it “fills” the entire row thanks to the fill=tk.X
option.
We also want the label widget to show up on the bottom-left of the screen, hence we used side=tk.LEFT
. If you want it to be in the center, do not specify any option. You can also use side=tk.RIGHT
to make it appear on the bottom-right.
Next we will define two methods for our Status bar class, one which updates the label with some new text, and the other which clears the text back to an empty string.
def set(self, newText):
self.label.config(text=newText)
def clear(self):
self.label.config(text="")
Now you can copy paste this StatusBar Class into your own code and begin using it like a regular widget. The below code shows you how to utilize this Class.
import tkinter as tk
class Window:
def __init__(self, master):
self.frame = tk.Frame(master)
b1 = tk.Button(self.frame, text="Button 1", command=self.handleButtonOne)
b1.pack(padx=30, pady=20)
b2 = tk.Button(self.frame, text="Button 2", command=self.handleButtonTwo)
b2.pack(padx=30, pady=20)
self.status = StatusBar(self.frame)
self.frame.pack(expand=True, fill=tk.BOTH)
def handleButtonOne(self):
self.status.set("Button 1 was clicked")
def handleButtonTwo(self):
self.status.set("Button 2 was clicked")
root = tk.Tk()
window = Window(root)
root.mainloop()
Here is the output:
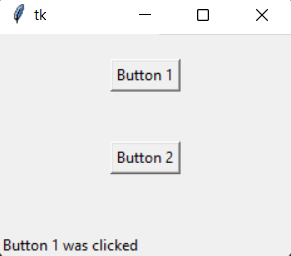
Customizing the StatusBar
Since it is our Class, we can make as many modifications as we want, and allow for extra parameters to customize things like the background or text color, as shown below.
import tkinter as tk
class StatusBar(tk.Frame):
def __init__(self, master, color):
tk.Frame.__init__(self, master, background = color)
self.label = tk.Label(self, text = "", fg = "white", bg = color)
self.label.pack(side = tk.LEFT)
self.pack(fill=tk.X, side = tk.BOTTOM)
def setText(self, newText):
self.label.config(text = newText)
def clearText(self):
self.label.config(text = "")
class Window:
def __init__(self, master):
self.frame = tk.Frame(master)
b1 = tk.Button(self.frame, text="Button 1", command=self.handleButtonOne)
b1.pack(padx=30, pady=20)
b2 = tk.Button(self.frame, text="Button 2", command=self.handleButtonTwo)
b2.pack(padx=30, pady=20)
self.status_bar = StatusBar(self.frame, "blue")
self.frame.pack(expand = True, fill = tk.BOTH)
def handleButtonOne(self):
self.status_bar.setText("Button 1 was Clicked")
def handleButtonTwo(self):
self.status_bar.setText("Button 2 was Clicked")
root = tk.Tk()
window = Window(root)
root.mainloop()
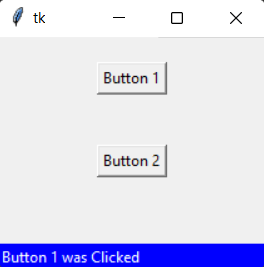
This marks the end of the Tkinter Status Bar Tutorial. Any suggestions or contributions for CodersLegacy are more than welcome. Questions about the tutorial content can be asked in the comments section below.