In this Python Tutorial, we will learn how to create draggable annotations in Matplotlib. Annotations are useful for adding textual or graphical information to your plots. By making annotations draggable, you can interactively reposition them within the plot, which can be particularly useful for exploring data or highlighting specific points of interest.
Creating a Matplotlib Plot
First, we will create a basic matplotlib plot which shows some basic data.
import matplotlib.pyplot as plt
fig, ax = plt.subplots()
x = [1, 2, 3, 4, 5]
y = [10, 8, 6, 4, 2]
ax.scatter(x, y)
plt.show()
Here is our current matplotlib plot, which does not yet have any annotations on it.
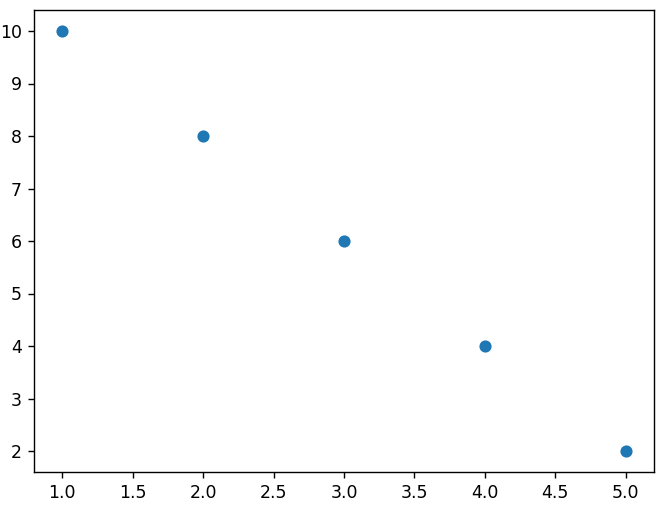
Adding Annotations to our Matplotlib Plot
The next step is to add some annotations to our matplotlib plot. By default, these annotations will be non-draggable. We will create a new function where we write our annotation code, so that we can easily re-use this function to create as many annotations as we want.
def create_draggable_annotation(text, x, y):
bbox_args = dict(boxstyle="round", facecolor="wheat")
annotation = ax.annotate(text, xy=(x, y), bbox=bbox_args)
ax.add_artist(annotation)
return annotation
We will now call this function twice, to create two annotations.
annotation1 = create_draggable_annotation("Annotation 1", 3, 5)
annotation2 = create_draggable_annotation("Annotation 2", 1, 3)
This gives us the following result:
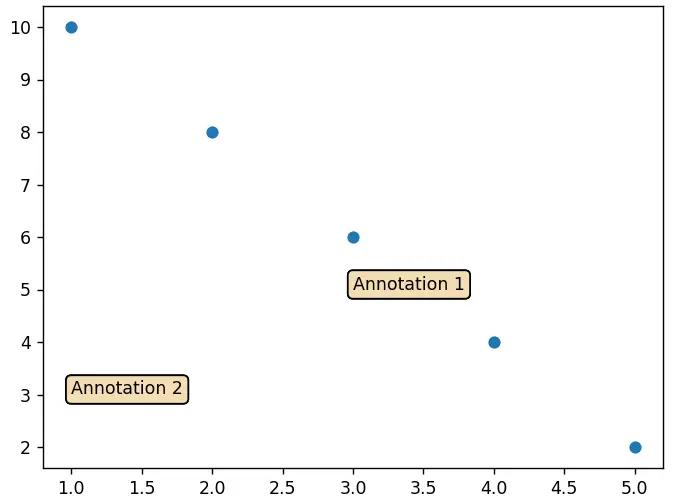
If you wish to learn more about the different types of annotations, and how you can customize them, follow the link.
Making our Matplotlib Annotations Draggable
These annotations that we have created are not draggable yet. To make these annotations interactive, all we have to do is call the draggable()
method on an annotation object. We will update our function accordingly to now create draggable annotations.
Here is the complete code (along with the modifications for making the annotations draggable):
import matplotlib.pyplot as plt
def create_draggable_annotation(text, x, y):
bbox_args = dict(boxstyle="round", facecolor="wheat")
annotation = ax.annotate(text, xy=(x, y), bbox=bbox_args)
annotation.draggable()
ax.add_artist(annotation)
return annotation
fig, ax = plt.subplots()
x = [1, 2, 3, 4, 5]
y = [10, 8, 6, 4, 2]
ax.scatter(x, y)
annotation1 = create_draggable_annotation("Annotation 1", 3, 5)
annotation2 = create_draggable_annotation("Annotation 2", 1, 3)
plt.show()
Try running this code for yourself, and have an interactive session with the annotations.
This marks the end of the Tutorial on Draggable Annotations in Matplotlib. Any suggestions or contributions for CodersLegacy are more than welcome. Questions regarding the tutorial content can be asked in the comments section below.