This tutorial covers the JFreeChart Bar Chart in Java.
The Bar Chart has always been a very standard way of representing data, as well as comparing two or more data sets. You can also change the orientation of the bar chart between either vertical or horizontal.
The Java library JFreeChart brings in support for the creation and manipulation of Bar Charts. In this tutorial we will explain how to use JFreeChart to create high quality bar charts.
In this tutorial we’ll be explaining how to create Bar Charts with the Java JFreeChart Library. We’ll be including two different versions here, one with a JavaFX integration and the other with a more standard image output. Benefits of both are described in their respective sections.
JFreeChart Bar Chart Example
We’ll start off by creating a simple JFreeChart Bar Chart and explaining the example code step by step.
The very first thing we did was to create a bunch of different String variables to act as labels and names for our JFreeChart BarChart.
Next we created a dataset object and began inserting data into it using the addValue()
method. The addValue()
method takes 3 parameters, the first is the actual “value” to be plotted. The second is the “series” name which all data of a specific type should share. The third is the name of the bar that will appear for that value. Look at the output image for more understanding.
import org.jfree.chart.ChartFactory;
import org.jfree.chart.ChartUtils;
import org.jfree.chart.JFreeChart;
import org.jfree.chart.plot.PlotOrientation;
import org.jfree.data.category.DefaultCategoryDataset;
import java.io.File;
import java.io.IOException;
public class Tutorial {
public static void main(String[] args) throws IOException {
String P1 = "Player 1";
String P2 = "Player 2";
String Attk = "Attack";
String Def = "Defense";
String Speed = "Speed";
String Stealth = "Stealth";
DefaultCategoryDataset dataset = new DefaultCategoryDataset( );
// Player 1
dataset.addValue(10, P1, Attk);
dataset.addValue(7, P1, Def);
dataset.addValue(6, P1, Speed);
dataset.addValue(6, P1, Stealth);
// Player 2
dataset.addValue(7, P2, Attk);
dataset.addValue(9, P2, Def);
dataset.addValue(8, P2, Speed);
dataset.addValue(8, P2, Stealth);
JFreeChart barChart = ChartFactory.createBarChart("JFreeChart BarChart", "Players", "Points",
dataset, PlotOrientation.VERTICAL, true, true, false);
ChartUtils.saveChartAsPNG(new File("C://Users/CodersLegacy/Desktop/histogram.png"), barChart, 650, 400);
}
}
Once the dataset object is created you just have pass it into the createBarChart
function along with the names for labels of the X and Y axis. Lastly, you have to use the saveChartAsPNG
to create the image with a file path (where the image will be saved), the bar chart object and the width and height dimensions.
Below is the output from the above code.
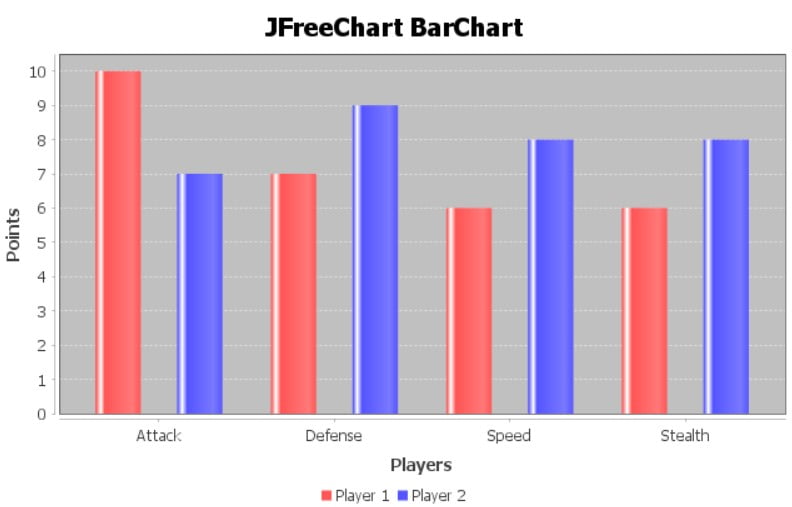
You can also change the orientation of the bar chart by changing the Orientation.VERTICAL
setting to Orientation.HORIZONTAL
.
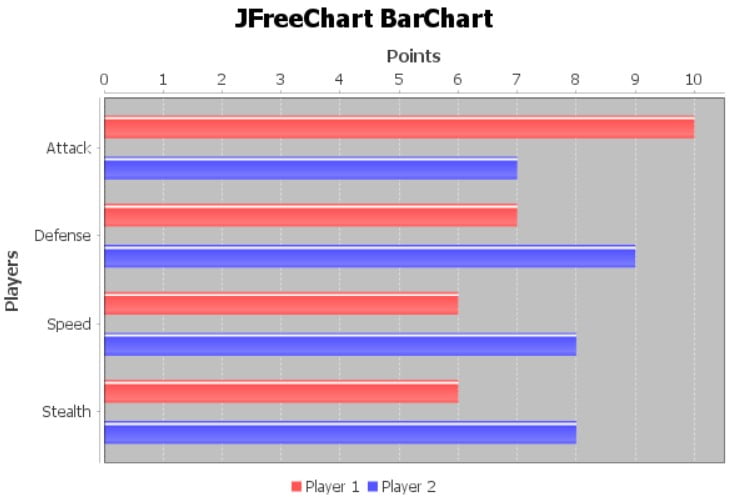
JFreeChart BarChart with JavaFX
JavaFX is one of the many GUI libraries in Java. Because it’s the latest and most well used we’re using this to demonstrate how to insert a JFreeChart Bar Chart into a GUI window instead of having an image file as the output.
There are a few pre-requisites required to use this JavaFX version, and as you can see, there are some new imports included as well. Read this tutorial (if you don’t already have JavaFX setup) which explains the process of bringing in JavaFX support (it’s just a single step using Maven) into JFreeChart.
You need to add 2 extra methods to our class, one for the launching the JavaFX application and the second which contains the JavaFX code. One of our functions will remain mostly unchanged, (the middle one) which creates the barChart from the data and returns it into the JavaFX function.
By using the ChartViewer class, we can directly add the BarChart into the JavaFX Scene.
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.stage.Stage;
import org.jfree.chart.ChartFactory;
import org.jfree.chart.ChartUtils;
import org.jfree.chart.JFreeChart;
import org.jfree.data.statistics.HistogramDataset;
import org.jfree.chart.plot.PlotOrientation;
import org.jfree.data.category.DefaultCategoryDataset;
import org.jfree.chart.fx.ChartViewer;
public class Tutorial extends Application{
public static void main(String[] args) {
launch(args);
}
public static JFreeChart createChart() {
String P1 = "Player 1";
String P2 = "Player 2";
String Attk = "Attack";
String Def = "Defense";
String Speed = "Speed";
String Stealth = "Stealth";
DefaultCategoryDataset dataset = new DefaultCategoryDataset( );
// Player 1
dataset.addValue(10, P1, Attk);
dataset.addValue(7, P1, Def);
dataset.addValue(6, P1, Speed);
dataset.addValue(6, P1, Stealth);
// Player 2
dataset.addValue(7, P2, Attk);
dataset.addValue(9, P2, Def);
dataset.addValue(8, P2, Speed);
dataset.addValue(8, P2, Stealth);
JFreeChart barChart = ChartFactory.createBarChart("JFreeChart BarChart", "Players", "Points",
dataset, PlotOrientation.VERTICAL, true, true, false);
return barChart;
}
@Override
public void start(Stage stage) throws Exception {
ChartViewer viewer = new ChartViewer(createChart());
stage.setScene(new Scene(viewer));
stage.setTitle("JFreeChart: BarChart");
stage.setWidth(600);
stage.setHeight(400);
stage.show();
}
}
A screenshot of the output. You can see JavaFX GUI window around the JFreeChart Bar Chart. The benefit of using JavaFX is that this chart generates immediately at the runtime and by adding extra features like buttons you can make it more interactive (like changing values and updating the chart).
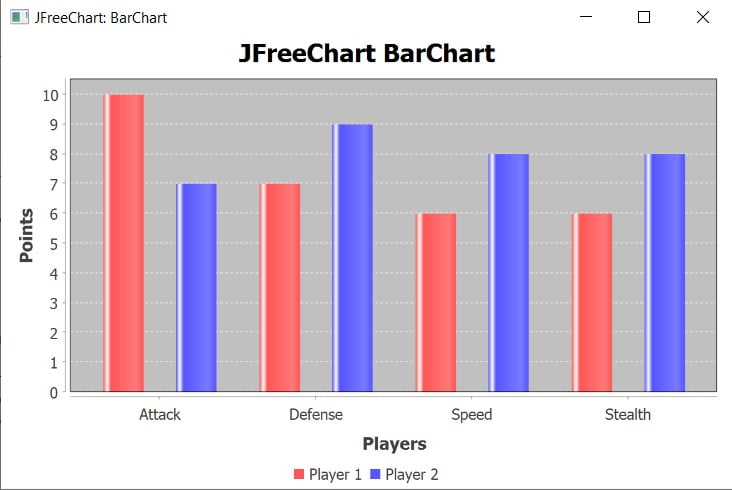
This marks the end of the JFreeChart Bar Chart tutorial. Any suggestions or contributions for CodersLegacy are more than welcome. Questions regarding the article content can be asked in the comments section below.