The article is about the File Handling in Java.
Before we begin, know that there are many different classes in Java related to File Handling. They have a lot in common aside from a few differences so the concept remains roughly the same. Some of these Classes are File, FileResource, FileReader, FileWriter, FileOutputStream, FileInputStream, BufferedWriter and BufferedReader.
Typically anything with Input or Reader is used to read data from files, whereas anything with Output or Writer is used to write data to files. In this article, we’ll cover File
, FileWriter
and FileReader
.
FileWriter
The FileWriter class is used to write data in the form of text to text files. It writes a stream of characters to the file, as compared to the stream of bytes that the FileOutputStream class writes.
Before we use any of the methods in the FileWriter class, we must declare an object for it.
FileWriter obj = new FileWriter(file_path)
- obj is the name of the object which we creating from the FileReader Class.
- The new keyword is used to create the object from the FileReader Class.
- The file_path must be a string and a valid path to a text file on your computer.
In the code below we are going to write to a file called “Data.txt”. If this file does not already exist, the FileWriter Class is create it for you.
Remember to import the two following classes into your code as they are not included normally. Secondly, remember to include the throws IOException
statement. It’s part of Java’s behavior to throw an IO exception when reading or writing. Finally, it’s the write()
function that we use to insert text into the file.
import java.io.FileWriter;
import java.io.IOException;
public class example {
public static void main(String[] args) throws IOException {
FileWriter file = new FileWriter("C://CodersLegacy/Data.txt");
String data;
data = "File Handling in Java - CodersLegacy";
file.write(data);
file.close();
}
}
Below is a screenshot of the text file created by the above code. If a file with this name had already existed, any data in it would have been wiped and overwritten with the below text.
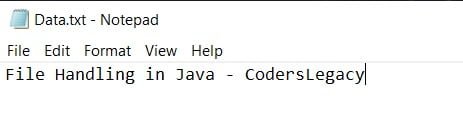
Remember to call the close() function on the object you created to free up memory resources for the program to use elsewhere.
FileReader
The FileReader class is used to read text data from files. It reads a stream of characters from the file, as compared to the stream of characters that the FileInputStream class reads.
Before we use any of the methods in the FileReader class, we must declare an object for it. The syntax for this is the same as the FileWriter code if you swap out FileWriter for FileReader.
FileReader obj = new FileReader(file_path)
In this example we’ll use the same file we created in the FileWriter
section. We’ll be using the read()
method belonging to the FileReader
Class. It is used to return a character’s ASCII integer value and returns -1 at the end of file.
import java.io.FileReader;
import java.io.IOException;
public class example {
public static void main(String[] args) throws IOException {
FileReader file = new FileReader("C://CodersLegacy/Data.txt");
int data;
while ((data = file.read()) != -1) {
System.out.print((char)data);
}
file.close();
}
}
The read()
function only returns one character at a time, so we have to employ the use of a while loop. Each iteration of the while loop moves the file to the next character. We convert the output to char
type else an integer ASCII value would be displayed.
If you want to display the text in the below format, use the print()
function, not println()
. Else one character would be displayed per line.
File Handling in Java - CodersLegacy
Once again, remember to call the close() function on the object you created.
File
The File class has many useful methods that prove useful during File Handling in Java. We’ll discuss the most useful ones here briefly. All the below functions take a file path as their input argument.
First up, is the exists()
function which is used to check if a file at the specified file path exists. It’s a good idea to use the exists()
function before attempting to read or write from a specified file path. This helps prevent any nasty surprises down the road.
The delete()
function has the unique ability to delete a file at a specific file path. It’s advised to be careful while using this. You might end up permanently deleting a valuable file from your PC.
The length()
Function returns the length of a file in bytes.
The mkdir()
function takes as input a file path, and creates a directory at that location. You can think of it as creating a folder.
The list()
function takes a directory path as input, as returns the names of all the files in it, in Array format.
Lastly, the createNewFile()
is another way to create Files in Java. All you have to do is pass an appropriate path into it’s parameters.
All these functions are methods of the File class, so you will have to call them in the following format, File.methodname
.
This marks the end of the Java File Handling section. Any suggestions or contributions for CodersLegacy are more than welcome. You can ask any relevant questions in the comments section below.