This article covers the ComboBox widget in JavaFX.
The ComboBox widget is a popular choice for space-constrained GUI’s in JavaFX. Since it has a drop-down menu that’s retractable (folds into the widget), it doesn’t take up much space. On this drop-down menu are list of options that the User may pick from you.
You will also find a complete list of methods for the ComboBox available at the bottom of this page.
Another space-saving component is the MenuButton!
JavaFX ComboBox Example
Creating a ComboBox object is pretty straightforward. Next up you actually have to begin adding the options you want to present the user. We’ll do this by first calling the list of items the widget has by using the getItems()
method.
Next we’ll add an item to this list using the add()
method. This directly adds the new option to the list.
If you aren’t satisfied with this method of adding options, we’ll be discussing an alternate method near the end of this page.
package application;
import javafx.application.Application;
import javafx.stage.Stage;
import javafx.scene.Scene;
import javafx.scene.control.ComboBox;
import javafx.scene.layout.VBox;
public class Tutorial extends Application {
public static void main(String args[]){
launch(args);
}
@Override
public void start(Stage primaryStage) throws Exception {
VBox layout = new VBox();
Scene scene = new Scene(layout, 300, 300);
ComboBox combo = new ComboBox();
combo.getItems().add("Option 1");
combo.getItems().add("Option 2");
combo.getItems().add("Option 3");
combo.getItems().add("Option 4");
combo.getItems().add("Option 5");
layout.getChildren().addAll(combo);
primaryStage.setTitle("CodersLegacy");
primaryStage.setScene(scene);
primaryStage.show();
}
}
Below is the GUI output for the above code.
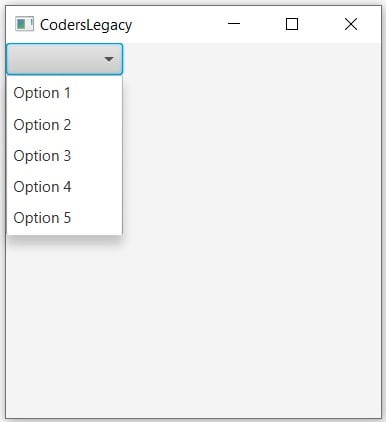
Retrieving ComboBox values
If you’ve been following our JavaFX tutorial series uptil now, you’ll notice that the method described below is the same for many other input widgets, using a value return function (getValue()
) and a button widget.
package application;
import javafx.application.Application;
import javafx.stage.Stage;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.scene.control.ComboBox;
import javafx.scene.layout.VBox;
public class Tutorial extends Application {
public static void main(String args[]){
launch(args);
}
@Override
public void start(Stage primaryStage) throws Exception {
VBox layout = new VBox();
Scene scene = new Scene(layout, 300, 300);
ComboBox combo = new ComboBox();
combo.getItems().add("Option 1");
combo.getItems().add("Option 2");
combo.getItems().add("Option 3");
combo.getItems().add("Option 4");
combo.getItems().add("Option 5");
Button button = new Button("Click me");
button.setOnAction(e -> {
System.out.println(combo.getValue());
});
layout.getChildren().addAll(combo, button);
primaryStage.setTitle("CodersLegacy");
primaryStage.setScene(scene);
primaryStage.show();
}
}
The output:
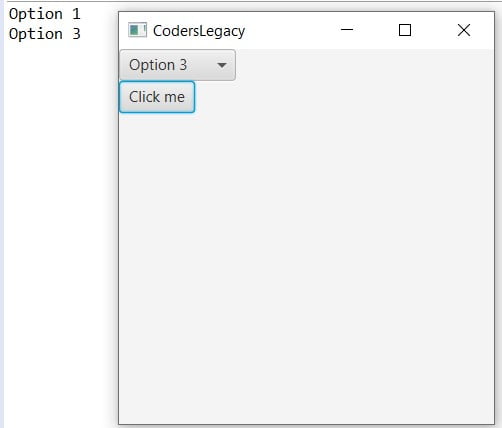
An Alternate Method
Instead of adding each option individually, there is a way to add them all at once.
With the use of an Array containing a list of all our options and the use of the FXCollections
class, we can achieve our goal within 2 lines.The output for below code will exactly match it’s previous equivalent.
package application;
import javafx.application.Application;
import javafx.collections.FXCollections;
import javafx.stage.Stage;
import javafx.scene.Scene;
import javafx.scene.control.ComboBox;
import javafx.scene.layout.VBox;
public class Tutorial extends Application {
public static void main(String args[]){
launch(args);
}
@Override
public void start(Stage primaryStage) throws Exception {
VBox layout = new VBox();
Scene scene = new Scene(layout, 300, 300);
String options[] = { "Option 1", "Option 2", "Option 3",
"Option 4", "Option 5" };
ComboBox combo = new ComboBox(FXCollections.observableArrayList(options));
layout.getChildren().addAll(combo, button);
primaryStage.setTitle("CodersLegacy");
primaryStage.setScene(scene);
primaryStage.show();
}
}
Don’t forget to include that extra import for FXcollections!
ComboBox Methods
A compilation of many useful methods for the ComboBox widget.
Method | Description |
---|---|
setEditable(bool) | Default value is False. Setting it two True will allow to user to enter his own custom choice. |
getValue() | Returns the currently selected value in the Combobox. |
getItems() | Returns a list of the options in the ComboBox. |
setVisibleRowCount(n) | Sets the number of rows that will appear in the menu at a given time. |
getVisibleRowCount() | Returns the value of the number of rows to be displayed at a given time. |
This marks the end of the JavaFX ComboBox article. Any suggestions or contributions for CodersLegacy are more than welcome. Questions regarding the article content can be asked in the comments section below.