This article is a tutorial on JavaFX FileChooser dialogs.
File Dialogs or “File Choosers” are an important part of any software that involves a GUI, JavaFX or not. File Dialogs have the important ability of allowing the user to browse through the computer and select/save a file at the file path of their choice. Even in small GUI program’s, this ability will surely come in handy.
In the tutorial below we’ll be discussing two different types of Dialogs that we can open using the JavaFX FileChooser component.
Creating a FileChooser Dialog
As we mentioned earlier, we can create two different dialogs, the OpenDialog and SaveDialog. The first dialog is used to select files, while the second is used to select directories.
We’ll be creating a GUI program with two buttons, each rigged to one of the above two dialogs.
package application;
import java.io.File;
import javafx.application.Application;
import javafx.geometry.Pos;
import javafx.stage.FileChooser;
import javafx.stage.Stage;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.scene.layout.VBox;
public class Tutorial extends Application {
public static void main(String args[]){
launch(args);
}
@Override
public void start(Stage primaryStage) throws Exception {
Button button1 = new Button("Open File");
Button button2 = new Button("Save File");
button1.setOnAction(e -> {
FileChooser filechooser = new FileChooser();
filechooser.setTitle("Open File");
File selected = filechooser.showOpenDialog(primaryStage);
});
button2.setOnAction(e -> {
FileChooser filechooser = new FileChooser();
filechooser.setTitle("Save File");
File selected = filechooser.showSaveDialog(primaryStage);
});
VBox layout = new VBox(button1, button2);
layout.setAlignment(Pos.CENTER);
layout.setSpacing(25);
Scene scene = new Scene(layout, 200, 200);
primaryStage.setTitle("CodersLegacy");
primaryStage.setScene(scene);
primaryStage.show();
}
}
Pressing the first button opens up the following JavaFX FileChooser dialog used to select files, returning the filepath of the selected file.
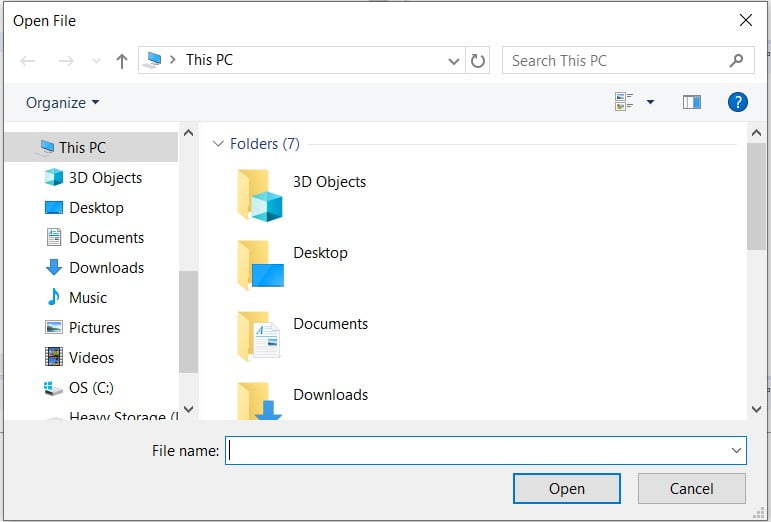
Pressing the first button opens up the following JavaFX FileChooser dialog which allows you select a folder/directory to save a File in. It returns the filepath of the selected directory.
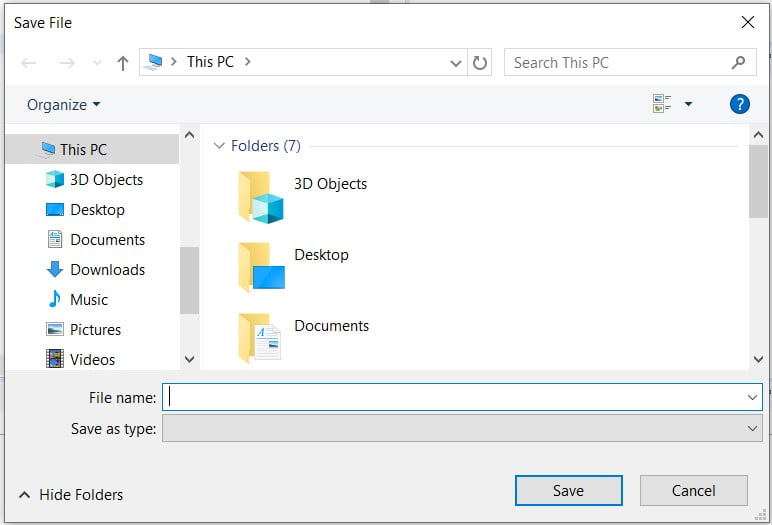
Extra FileChooser Functions
A brief look through some of the other useful functions available for the JavaFX FileChooser component.
File Filters
In Folders with a large number of files, file filters can come in handy if you only what to see a certain type of file. Commonly used file filters are for text or (MS) word files.
filechooser.getExtensionFilters().addAll(
new FileChooser.ExtensionFilter("Text Files", "*.txt")
,new FileChooser.ExtensionFilter("Python Files", "*.py")
);
These are what file filters look like in a JavaFX FileChooser dialog.
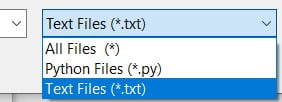
Initial File Name
Sets a Default name when the JavaFX FileChooser dialog opens. Might be useful when you’re using the SaveDialog feature as a default save name.
file.setInitialFileName("myfile.txt");

Initial File Directory
By changing the initial File directory you can have your file dialog open in the directory of your choosing. Can help save your user some time if you do it correctly.
file.setInitialDirectory(new File("D://"));
The line above will cause the JavaFX OpenDialog to start up in D drive.
This marks the end of the JavaFX FileChooser tutorial. Any suggestions or contributions for CodersLegacy are more than welcome. Questions regarding the article content can be asked in the comments section below.