This tutorial covers the Font class in JavaFX.
By default, the Text Class in JavaFX which is responsible for creating and displaying text does not have many options to change the appearance of the text itself. However, the JavaFX Font class comes equipped with the necessary options required to modify various things regarding the text, like it’s size, font-family, positioning etc.
The JavaFX Font Class is represented by javafx.scene.text.Font
.
Be sure to read up on the JavaFX Text Class first, else you’ll have trouble.
JavaFX Font Syntax
The JavaFX Font class has four parameters which control the appearance of the text. We’ll discuss each one individually below in the order that they appear.
text.setFont(Font.font("Verdana", FontWeight.BOLD, FontPosture.REGULAR, 20));
It’s not compulsory to have all of these parameters at once, you only need to include the ones that you want.
1# Font Family
Font Families refer to the style and type of font. A small list of a few popular font-families that we tried and tested.
- Verdana
- Helvetica
- Times New Roman
- Comic Sans MS
- Impact
- Lucida Sans Unicode
2# Font Weight
Determines the “weight” of the Font. The lowest setting leaves a very light imprint, while the highest setting makes it extra bold (dark).
- FontWieght.BLACK
- FontWieght.BOLD
- FontWieght.EXTRA_BOLD
- FontWieght.EXTRA_LIGHT
- FontWieght.LIGHT
- FontWieght.MEDIUM
- FontWieght.NORMAL
- FontWieght.SEMI_BOLD
- FontWieght.THIN
3# Font Posture
Determines whether the font will be of regular type or italic. Pass FontPosture.REGULAR for regular font and FontPosture.ITALIC for italic.
4# Font Size
The simplest parameter which determines the size of the displayed text. The font sizes for normal text range between 14 – 18. 20 and above are used for headings. You can also pass decimal values as this parameter is of type double.
JavaFX Font Example
Using all the parameters that we discussed above, as well as several from the Text class, we created the following example.
package application;
import javafx.application.Application;
import javafx.stage.Stage;
import javafx.scene.Scene;
import javafx.scene.layout.Pane;
import javafx.scene.text.Font;
import javafx.scene.text.FontPosture;
import javafx.scene.text.Text;
public class Tutorial extends Application {
public static void main(String args[]){
launch(args);
}
@Override
public void start(Stage primaryStage) throws Exception {
Text text = new Text(" Hello World.\n JavaFX Font Example.");
text.setY(100);
text.setX(20);
text.setFill(Color.RED);
text.setFont(Font.font("Lucida Sans Unicode", FontPosture.ITALIC, 20));
Pane layout = new Pane(text);
Scene scene = new Scene(layout, 250, 200);
primaryStage.setTitle("CodersLegacy");
primaryStage.setScene(scene);
primaryStage.show();
}
}
The GUI output of the above example:
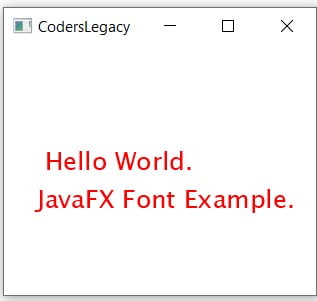
Font Methods
A list of commonly used methods for the JavaFX Font objects.
Method | Description |
---|---|
getFontNames() | Returns a list of installed fonts on the system it’s being run on. |
getDefault() | Returns the default font being used on the system it’s run on. |
getFamilies() | Returns a list of installed font families on the system it’s being run on. |
getFamily() | Returns the family name of the font it’s used on. |
loadFont() | Used to manually insert a font. Takes a filepath as input parameter which represents the location of the font. |
This marks the end of the JavaFX Font tutorial. Any suggestions or contributions for CodersLegacy are more than welcome. Questions regarding the article content can be asked in the comments section below.