This article covers Input Dialogs in JavaFX
There are many ways of taking input in JavaFX using widgets like TextField and TextArea. However, there is another unique way, using dialogs which we call “Input dialogs”. These work through the use of prompts that activate after certain actions (e.g button click) and present the user with an entry field and a set of appropriate buttons.
We’ll be using the JavaFX TextInputDialog Class to create these dialogs.
Be sure to check out our Alert Dialogs with JavaFX article as well!
JavaFX Input Dialog Example
Using the TextInputDialog Class we’ll create the JavaFX Input Dialog, then using the show()
method, we’ll display it on screen. Of course, there are many other methods and functions that we can use.
The setContentText()
method is used to set the text for the main text area in the dialog. Next is the setHeaderText()
method, used to change the Header text that appears on the dialog like a heading. Finally, the text that appears in the textfield in the dialog, is set by passing text into the parameters of the JavaFX TextInputDialog Class.
package application;
import javafx.application.Application;
import javafx.geometry.Insets;
import javafx.stage.Stage;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.scene.control.ButtonType;
import javafx.scene.control.TextField;
import javafx.scene.control.TextInputDialog;
import javafx.scene.layout.VBox;
public class Tutorial extends Application {
public static void main(String args[]){
launch(args);
}
@Override
public void start(Stage primaryStage) throws Exception {
TextInputDialog inputdialog = new TextInputDialog("Enter some Text");
inputdialog.setContentText("Text: ");
inputdialog.setHeaderText("JavaFX Input Dialog Example");
Button button = new Button("JavaFX Input Dialog");
button.setOnAction(e -> {
inputdialog.show();
});
VBox layout = new VBox(button);
layout.setMargin(button, new Insets(20,20,20,20));
Scene scene = new Scene(layout, 300, 200);
primaryStage.setTitle("CodersLegacy");
primaryStage.setScene(scene);
primaryStage.show();
}
}
The GUI output of the above example:
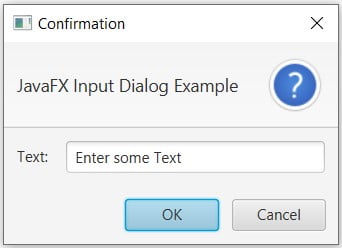
Returning the entered text
Now that you’ve created the input dialog using the JavaFX TextInputDialog Class, it’s time to actually return the text that the user entered in the textfield.
We’ll have to change the show()
method to showAndWait()
. Next we’ll be using the getEditor()
and getText()
functions respectively to retrieve the text stored in the TextField of the JavaFX input dialog. Once we have retrieved the text, we’ll store into the label we just created so that we can see it.
TextInputDialog inputdialog = new TextInputDialog("Enter some Text");
inputdialog.setContentText("Text: ");
inputdialog.setHeaderText("JavaFX Input Dialog Example");
Button button = new Button("JavaFX Input Dialog");
Label label = new Label("");
button.setOnAction(e -> {
inputdialog.showAndWait();
label.setText(inputdialog.getEditor().getText());
});
VBox layout = new VBox(button, label);
layout.setSpacing(20);
layout.setMargin(button, new Insets(20,20,20,20));
Scene scene = new Scene(layout, 300, 200);
The output:
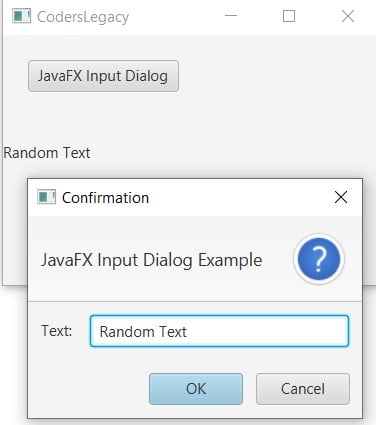
This marks the end of the JavaFX Input Dialogs article. Any suggestions or contributions for CodersLegacy are more than welcome. Questions regarding the article content can be asked in the comments section below.