This is a tutorial on the JavaFX Separator, complete with examples.
The JavaFX Separator is a GUI component that is used as a visual divider between components. We have multiple techniques to avoid components being too close to each other, such as padding and spacing. The JavaFX separator is a “visible” type of spacing method.
The JavaFX Separator is represented by the javafx.scene.control.Separator
class. You’ll find this helpful for when you’re importing the Separator class into your program.
JavaFX Separator Example
When creating the JavaFX separator from the Separator class, be sure to pass an orientation to it. You can choose from either Orientation.HORIZONTAL
or Orientation.VERTICAL
, causing a horizontal and vertical alignment respectively for the separator.
You’ll have to be careful as to how you position the separator in the layout. If you want to have the separator appear between two components, make sure you keep the separator in between both components when adding them to the layout. As reference you can look at the below code.
package application;
import javafx.application.Application;
import javafx.geometry.Orientation;
import javafx.stage.Stage;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.scene.control.Separator;
import javafx.scene.layout.VBox;
public class Tutorial extends Application {
public static void main(String args[]){
launch(args);
}
@Override
public void start(Stage primaryStage) throws Exception {
Button button1 = new Button("Button1");
Button button2 = new Button("Button2");
Separator separator = new Separator(Orientation.HORIZONTAL);
VBox layout = new VBox(button1, separator, button2);
Scene scene = new Scene(layout, 200, 100);
primaryStage.setTitle("CodersLegacy");
primaryStage.setScene(scene);
primaryStage.show();
}
}
The JavaFX GUI output of the above example:
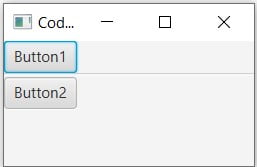
In the event that there is an obstruction or similar error, the separator will not display on screen.
Customizing the Separator
In it’s default state, the separator’s width (or height) is uncontrolled, resulting it in taking up as much space as it can. You may wish for a certain width or a “maximum” width (or height) for the separator. In this section we’ll show you how to do so.
We’ve also added some spacing between the components so the separator stands out a bit more.
Separator separator = new Separator(Orientation.HORIZONTAL);
separator.setMaxWidth(120);
VBox layout = new VBox(button1, separator, button2);
layout.setSpacing(5);
The output of the above example.:
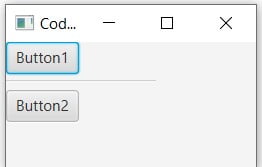
The Separator is an important component, especially in certain cases such as when you’re using something like the JavaFX Toolbar. Read up on the Toolbar as well to see how to use the separator effectively!
If you’re interested in learning more about the Separator, you can read up on the official documentation.
This marks the end of the JavaFX Separator article. Any suggestions or contributions for CodersLegacy are more than welcome. Questions regarding the article content can be asked in the comments section below.