In this Tkinter tutorial we will explore how to change the default window Icon. Often when building custom software, you need to change the default icon to something more meaningful, like a logo for your company or software. (Also because the default one makes your software look like it was a low-effort job)
Let’s get started!
Creating a Tkinter Window
To change the icon of a Tkinter window, you need to first create a Tk
object and a Tkinter window:
import tkinter as tk
# Create a Tk object
root = tk.Tk()
# Set the window title
root.title("My Window")
# Start the Tkinter event loop
root.mainloop()
If we run this code we get the following output.
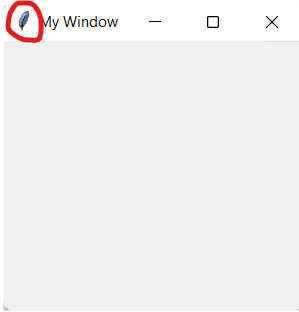
We have circled the default icon in the above image. Our goal is to now change this. (This code was run on the Window 11 OS. The look of the window/icon might be a bit different based on the OS you are using).
Changing the Icon of Tkinter Window
Once you have created a Tkinter window, you can use the iconbitmap
method to change its icon. The iconbitmap
method takes the path to an icon file (.ico
) as its argument and sets the icon of the window to the image in the file.
Here is an example of how you can use the iconbitmap
method to change the icon of a Tkinter window:
import tkinter as tk
root = tk.Tk()
# Set the window title
root.title("My Window")
# Set the window icon
root.iconbitmap("my_icon.ico")
root.mainloop()
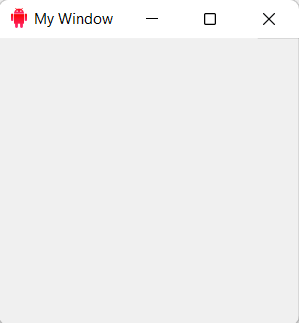
We have included a few .ico
files as a download here for you to try out.
Notes
Here are a few important notes to keep in mind when using the iconbitmap
method:
- The
iconbitmap
method only works with certain image formats, such as.ico
on Windows and.png
on macOS. - The image file must be in a specific size and format for the icon to display correctly. For more information, you can check the documentation for the
iconbitmap
method on the Tkinter website. - If the
iconbitmap
method is called with an invalid file path or an unsupported image format, the icon of the window will not be changed.
Using PNG as Icons on Windows
If you use the iconbitmap()
function with a png file, it most likely won’t work. In that case, there is another method that you can use, called iconphoto()
.
Here is a small example showing you how to use it.
import tkinter as tk
root = tk.Tk()
# Set the window title
root.title("My Window")
# Set the window icon
root.iconphoto(False, tk.PhotoImage(file="closeIcon.png"))
root.mainloop()
The first parameter is a boolean value, which controls whether or whether not the Icon should also be applied to any TopLevel windows created. The second parameter is a Tkinter PhotoImage object, which is created using a file name or file path of our icon file.
This marks the end of the How to change Tkinter Window Icon using iconbitmap)? Tutorial. Any suggestions or contributions for CodersLegacy are more than welcome. Questions about the tutorial content can be asked in the comments section below.