The CustomTkinter library (customtkinter) introduces several enhanced widgets to the standard Tkinter toolkit in Python. In this tutorial, we will focus on the CustomTkinter Button widget (CTkButton) and explore its features, differences from the standard Tkinter button widget, and how to utilize images with CTkButtons in your GUI applications.
CustomTkinter CTkButton vs. Tkinter Button:
The CTkButton widget provides additional functionality and customization options compared to the standard Tkinter Button widget. While both widgets serve the purpose of creating clickable buttons in GUIs, CTkButton offers extended parameters and capabilities. Here are some notable differences:
- Renamed Parameters: There are some parameters which have renamed. Below is a list of them (may not be a complete list) in the following format CTk-parameter -> Tk-parameter.
fg_color
->bg
text_color
->fg
- Additional Parameters:
hover_color
: Specifies the color of the button when the mouse pointer hovers over it.
text_color
: Defines the color of the button’s text.text_color_disabled
: Defines the color of the button’s text when it is disabled.
corner_radius
: Controls the roundedness of the button’s corners.border_spacing:
Internal spacing between text and borders.
- Customizable Styles: CTkButton provides a range of pre-defined styles, such as “raised,” “flat,” “outline,” and more. These styles give you the flexibility to create buttons with different visual appearances, allowing for better integration with your application’s overall design.
- Enhanced Interactivity: CTkButton supports additional events, such as the
on_enter
andon_leave
events triggered when the mouse pointer enters or exits the button. These events enable you to add dynamic behavior to your buttons, such as changing colors or displaying tooltips.
Example 1: Basic CTkButton Usage
In this example, we will create a simple CTkButton that triggers an action when clicked.
import tkinter as tk
from customtkinter import CTkButton
def handle_button_click():
print("Button clicked!")
# Create the main window
root = tk.Tk()
# Create a CTkButton
button = CTkButton(root, text="Click Me",
command=handle_button_click)
# Display the button
button.pack()
# Start the Tkinter event loop
root.mainloop()
This produces the following output (resized for visibility)
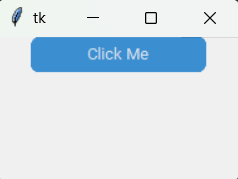
Explanation:
- We import the necessary modules, including
tkinter
for the main window andCTkButton
from thecustomtkinter
library. - Next, we define a function
handle_button_click()
that will be executed when the button is clicked. In this example, it simply prints a message. - We create the main window using
tk.Tk()
. - Then, we create a CTkButton widget called
button
with the specified text and command. - Finally, we use the
pack()
method to display the button within the main window, and we start the Tkinter event loop usingroot.mainloop()
.
Example 2: Customized CTkButton Styling
In this example, we will create a CTkButton with a customized style and additional parameters.
import tkinter as tk
from customtkinter import CTkButton
def handle_button_click():
button.configure(text="Clicked!",
fg_color="white",
text_color="black")
# Create the main window
root = tk.Tk()
# Create a CTkButton with customized parameters
button = CTkButton(root, text="Click Me",
fg_color="black",
hover_color="grey",
text_color="white",
corner_radius=5, font=("Arial", 12))
# Configure the button's command
button.configure(command=handle_button_click)
# Display the button
button.pack()
# Start the Tkinter event loop
root.mainloop()
Explanation:
- Similar to the previous example, we import the necessary modules and create the main window.
- We define a function
handle_button_click()
that changes the button’s text, background color, and foreground color when clicked. - We create a CTkButton called
button
with customized parameters, including background color (bg
), foreground color (fg
), hover color (hover_color
), active color (active_color
), border radius (border_radius
), and text font (text_font
). - Next, we configure the button’s command to execute
handle_button_click()
when clicked. - Finally, we display the button using
pack()
and start the Tkinter event loop.
Using Images with CTkButtons:
CTkButtons make it simple to incorporate images into your buttons. Follow these steps to utilize images with CTkButtons:
- Prepare the Image: Before using an image with a CTkButton, ensure that you have the image file (in a supported format like PNG or JPEG) available on your system.
- Import the Necessary Modules: Begin by importing the required modules in your Python script:
import tkinter as tk
from customtkinter import CTkButton
from PIL import ImageTk, Image
- Load and Resize the Image: Use the PIL (Python Imaging Library) module to load and resize the image:
# Load the image
image = Image.open("path_to_image_file")
# Resize the image as needed
resized_image = image.resize((width, height))
- Convert Image to Tkinter-Compatible Format: Convert the resized image into a Tkinter-compatible format using the
ImageTk
module:
# Convert the image to a Tkinter-compatible format
tk_image = ImageTk.PhotoImage(resized_image)
- Create the CTkButton and Assign the Image: Instantiate a CTkButton and assign the image to the
image
parameter:
# Create a CTkButton with an image
button = CTkButton(root, image=tk_image, text="Click Me")
- Display the Button: Add the button to your GUI by using the appropriate layout managers and geometry managers provided by Tkinter.
- Handle Button Clicks: To handle button clicks, bind a function to the button’s
command
parameter, just like you would with a standard Tkinter button.
Example Code:
Here’s an example that demonstrates the usage of CTkButton with an image:
import tkinter as tk
from customtkinter import CTkButton
from PIL import ImageTk, Image
def handle_button_click():
# Perform desired actions on button click
pass
# Create the main window
root = tk.Tk()
# Load and resize the image
image = Image.open("./zoom_in.png")
resized_image = image.resize((40, 40))
# Convert the image to a Tkinter-compatible format
tk_image = ImageTk.PhotoImage(resized_image)
# Create a CTkButton with an image
button = CTkButton(root,
image=tk_image,
text="Click Me",
command=handle_button_click)
# Display the button
button.pack()
# Start the Tkinter event loop
root.mainloop()
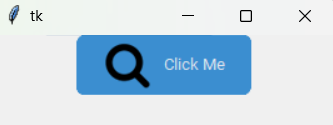
This marks the end of the CustomTkinter Button tutorial. Any suggestions or contributions for CodersLegacy are more than welcome. Questions regarding the tutorial content can be asked in the comments section below.