The CTkMessagebox module is a third-party module that provides custom messagebox functionality for Customtkinter applications. This module allows you to easily create informative, warning, error, and question message boxes with customizable icons and options.
The customtkinter module itself does not have the “custom tkinter” version of the messagebox widget available in the standard tkinter module. Hence, this is why we must either create our own, or use pre-built solutions from other community users such s the CTkMessagebox library.
Installation
Before using the CTkMessagebox module, you need to install it. Open your terminal or command prompt and enter the following command:
pip install CTkMessagebox
CTkMessagebox Examples
Example 1: Information Message Box
The information message box is a simple way to provide users with non-critical information.
from CTkMessagebox import CTkMessagebox
from customtkinter import CTkButton, CTk
def show_information():
CTkMessagebox(title="Information",
message="This is a basic information message.")
app = CTk()
bttn = CTkButton(app, text="Show Information", command=show_information)
bttn.pack(pady=50, padx=50)
app.mainloop()
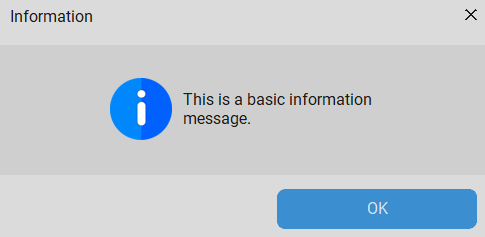
Example 2: Success Message Box with Checkmark
The success message box with a checkmark icon is suitable for conveying successful operations.
from CTkMessagebox import CTkMessagebox
from customtkinter import CTkButton, CTk
def show_success():
CTkMessagebox(message="Operation successful!",
icon="check",
option_1="OK")
app = CTk()
bttn = CTkButton(app, text="Show Success Message", command=show_success)
bttn.pack(pady=50, padx=50)
app.mainloop()
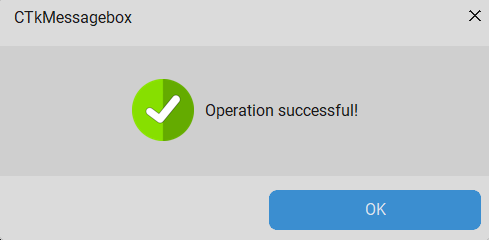
Example 3: Error Message Box with Retry Option
The error message box with a retry option is useful for handling and recovering from errors.
from CTkMessagebox import CTkMessagebox
from customtkinter import CTkButton, CTk
def show_error_retry():
msg = CTkMessagebox(title="Error",
message="Something went wrong!",
icon="cancel",
option_1="Retry",
option_2="Cancel")
if msg.get() == "Retry":
print("Retrying the operation...")
else:
print("Operation canceled.")
app = CTk()
bttn = CTkButton(app, text="Show Error with Retry", command=show_error_retry)
bttn.pack(pady=50, padx=50)
app.mainloop()
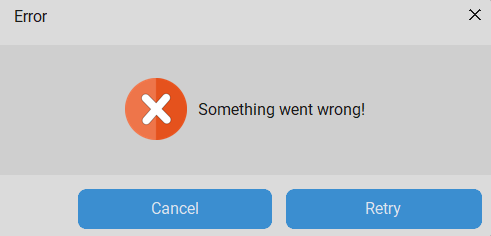
Example 4: Warning Message Box with Cancel/Retry Options
The warning message box with cancel and retry options is suitable for warning users about potential issues.
from CTkMessagebox import CTkMessagebox
from customtkinter import CTkButton, CTk
def show_warning():
msg = CTkMessagebox(title="Warning",
message="Connection lost!",
icon="warning",
option_1="Cancel",
option_2="Retry")
if msg.get() == "Retry":
print("Retrying the connection...")
else:
print("Connection canceled.")
app = CTk()
bttn = CTkButton(app, text="Show Warning with Options", command=show_warning)
bttn.pack(pady=50, padx=50)
app.mainloop()
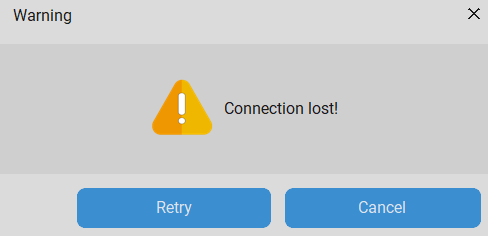
Example 5: Question Message Box with Yes/No/Cancel Options
The question message box with yes, no, and cancel options is useful for seeking user confirmation.
from CTkMessagebox import CTkMessagebox
from customtkinter import CTkButton, CTk
def ask_question():
msg = CTkMessagebox(title="Question",
message="Do you want to save changes?",
icon="question",
option_1="Cancel",
option_2="No",
option_3="Yes")
response = msg.get()
if response == "Yes":
print("Changes saved.")
elif response == "No":
print("Changes discarded.")
else:
print("Operation canceled.")
app = CTk()
bttn = CTkButton(app, text="Ask Yes/No/Cancel Question", command=ask_question)
bttn.pack(pady=50, padx=50)
app.mainloop()
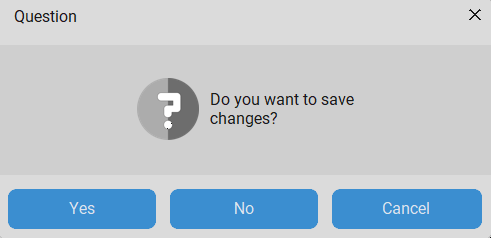
Feel free to customize these examples to fit your specific application needs, providing a user-friendly experience with informative and interactive messages. You can use of other styling options such as those from the ttk module to enhance your application’s UX and UI.
Custom Icons
You can also add your own custom icons, to match your custom messages in these message boxes.
Normally, when using these message boxes, we are using the default icons available as part of the library, simply by passing the appropriates string to the icon
parameter. To use custom icons, instead of a simple string, we will pass a file path of an .ico
file.
The below example features us setting a custom “bulb” icon.
from CTkMessagebox import CTkMessagebox
from customtkinter import CTkButton, CTk
def show_information():
CTkMessagebox(title="Information",
message="This is a basic information message.",
icon="bulb.ico")
app = CTk()
bttn = CTkButton(app, text="Show Information", command=show_information)
bttn.pack(pady=50, padx=50)
app.mainloop()
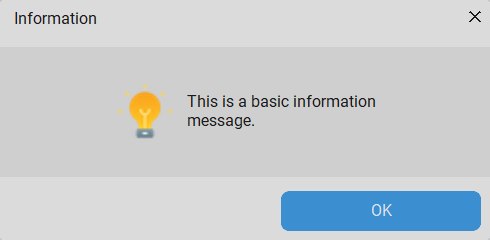
Here are a bunch of sample icons for you to download and use freely in your programs.
Download “Sample Icons” icons.zip – Downloaded 1386 times – 11.71 KBThis marks the end of the Customtkinter MessageBox using CTkMessagebox Tutorial. Any suggestions or contributions for CodersLegacy are more than welcome. Questions regarding the tutorial content can be asked in the comments section below.
Hello, I’d like to use CTkMessageBox not in a command, but calling it like a function, Is it possible
I mean:
show_info(message):
CTkMessagebox(title="Information",
message=message)
show_info(“some Information”)
Yeah
Sometimes when im using the icon, the icon makes the whole box not load and produces an error in python.
what error does it give, if you use icons that are not pre installed it will throw no directory found error..
so if you are using custom icon try to put full directoryy