The Python Tkinter filedialog module offers you a set of unique dialogs to be used when dealing with files. Tkinter has a wide variety of different dialogs, but the ones in filedialog are specifically designed for file selection. And as expected of dialog boxes, these are done in a very user friendly manner.
Below is a list of all the different Dialog options available. Make sure to import filedialog from tkinter as we have shown below. If you want to use tkinter as well as filedialog (which you most definitely will) you will have to import tkinter separately.
from tkinter import filedialog
filedialog.asksaveasfilename()
filedialog.asksaveasfile()
filedialog.askopenfilename()
filedialog.askopenfile()
filedialog.askdirectory()
filedialog.askopenfilenames()
filedialog.askopenfiles()
FileDialog Options
Discussing each function individually would get very repetitive and redundant since they all share the same options (one or two exceptions). Hence we’ll listed these options down below and simply provided examples of several of the above functions. Most of it is pretty self explanatory.
- parent – The Window on to which the dialog is to be placed
- title – The name that appears on the dialog
- initialdir – The directory the dialog first opens up in.
- initialfile – The file that the dialog has selected when it is opened.
- filetypes – Determines that type of the files to be loaded/saved in the dialogs. Passed as a tuple in a (Label, Filetype) format. You may also use the * wild card which applies to all filetypes. You can pass multiple tuples as well for multiple options.
- defaultextension – Default extension to be applied to files when appending to them. (Only applies to save dialogs)
- multiple – When true, it allows the selection of multiple items.
Alot of these options are actually optional. At most you’ll find yourself using 3 – 4 per function.
Returning a File Path
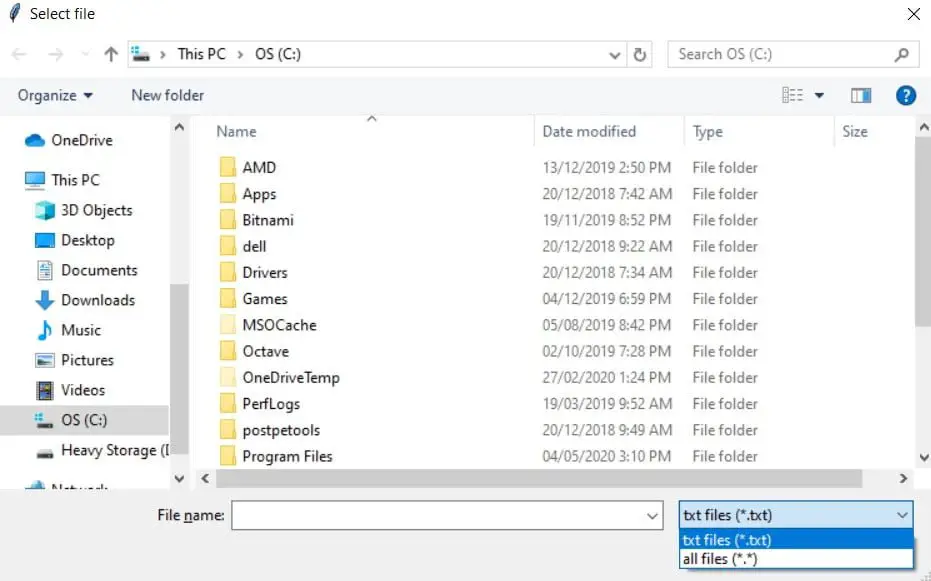
Pay attention to the File types option mentioned here. Notice how the option for filetypes above has effected the options in the GUI.
import tkinter as tk
from tkinter import filedialog
path = filedialog.askopenfilename(initialdir="/", title="Select file",
filetypes=(("txt files", "*.txt"),("all files", "*.*")))
Once you select a file, it’s file path will be returned back into your python program for you to use. Remember, you can only select files with this, not folders.
Use the askopenfilenames function if you want to select multiple files.
Selecting a File
The difference between the askopenfile function and askopenfilename(s) is that one returns the actual File object, and one simply returns the file path. In the example below, this is apparent. Where we select a random text file and see it’s output on screen after calling the read function.
import tkinter as tk
from tkinter import filedialog
root = tk.Tk()
path = filedialog.askopenfile(initialdir="/", title="Select file",
filetypes=(("txt files", "*.txt"),("all files", "*.*")))
print(path.read())
root.mainloop()
Below is the text that was written in the file we selected.
This is Python Tkinter FileDialog. Amazing right?
The look of the GUI is the exact same as the one for the askopenfilename function. And just like it, askopenfiles can be used to open several files.
Saving a File
The asksaveasfile function is used to save a file in a specified location. You get to select this location through the same selection GUI shown in the examples above.
import tkinter as tk
from tkinter import filedialog
root = tk.Tk()
path = filedialog.asksaveasfile(initialdir="/", title="Save file",
filetypes=(("txt files", "*.txt"),("all files", "*.*")))
root.mainloop()
For instance, you may navigate to the Desktop and save a file there with the name of your choice. Be sure to mention the extension when saving the files, otherwise use the defaultextension option to assign an extension to your files.
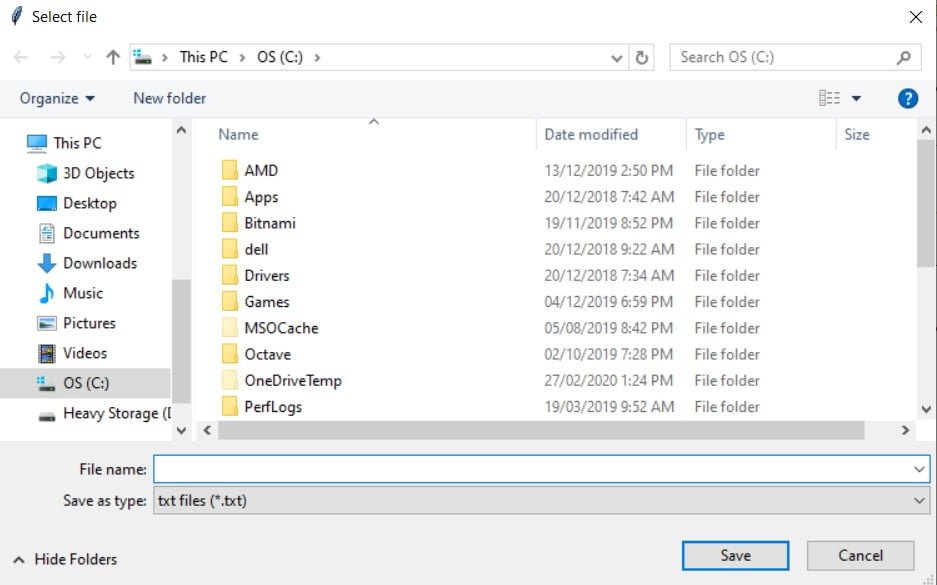
The above image shows the GUI for the asksaveasfile function. The biggest difference is the Save button instead of the Open button.
Selecting a Directory
This function is just like the askopenfilename function, except that it’s not used to select files, rather it selects directories (folders) and return their file path.
import tkinter as tk
from tkinter import filedialog
root = tk.Tk()
path = filedialog.askdirectory(initialdir="/", title="Select file")
print(path)
root.mainloop()
We selected the Users folder in C drive, and the following file path was returned.
C:/Users
Be sure not to include the filetypes option here. Folders don’t have filetypes after all.
This marks the end of the Python Tkinter filedialog article. Suggestions and Contributions for CodersLegacy are more than welcome. Any questions regarding the above material can be asked in the comments section below.
Head back to the main Tkinter article to learn about other great widgets!