In this tutorial we will discuss a particular issue that Matplotlib users sometimes face. Often when creating multiple figures in matplotlib, we run into a particular error which warns us about having too many figures currently in memory. This is an issue we can solving using the concept of “Reference Counting” with Matplotlib Figures.
Normally we create figures using the plt
module. Shown below is a very common format for creating a Figure and Axis in Matplotlib.
fig, ax = plt.subplots()
The problem here, is that the figure object created is not “reference counted”. To put it basically, this means that Matplotlib does not keep track of whether this figure object is still being used or not. So for example, even if we close the Matplotlib window, the figure will still remain inside the memory until the Python program itself is closed. Eventually you will get a warning (if your IDE supports warnings) about high memory consumption.
This issue shows up commonly in places where Matplotlib Windows are opened and closed often. Such as a GUI software which displays graphs on a separate window.
Now how do we solve this? Luckily, there is a way of creating Matplotlib figures which are reference counted.
Creating Reference Counted Figures in Matplotlib
The solution to this problem is actually very simple, and requires very little change to your existing code. Basically we just need to create figure
object in a way that does not involve pyplot. We will make a new import to the matplotlib.figure
module, and import the Figure
class from there.
from matplotlib.figure import Figure
Now wherever we have used plt.subplots()
to create the figure and axis object, we will replace that part with the following two lines.
fig = Figure()
ax = fig.add_subplot(111)
The first line creates the Figure object. You can also pass in several parameters in here, which you may have also used in the plt.subplots()
function, such as figsize
.
The second line of code adds a new axis object to our figure. We now have both our new figure and axis objects. They are no different than the ones created using plt.subplots()
, except they won’t suffer from the memory issue we discussed earlier.
Have fun with your matplotlib windows!
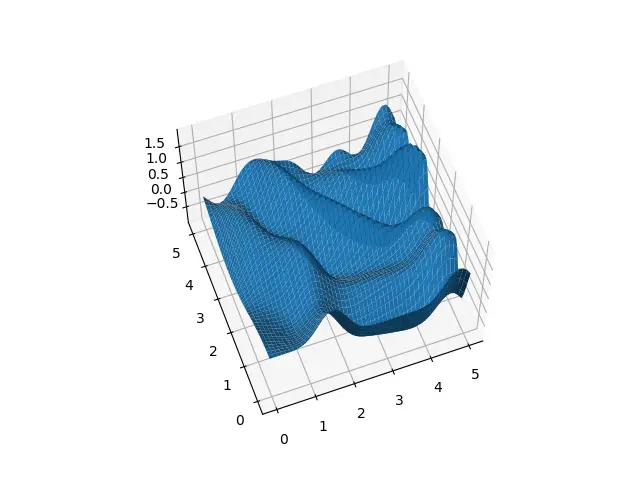
Curious as to how we made the above graph? Click the image to find out.
Note: There is another recommended approach for handling this problem, which is to explicitly close the figures created using plt(). Follow the link for more information on how this is done.
This marks the end of the Matplotlib Reference Counting for Figures Tutorial. Any suggestions or contributions for CodersLegacy are more than welcome. Questions regarding the tutorial content can be asked in the comments section below.