Taking input from the User quickly and accurately is an important part of any game. In this Pygame tutorial, we will explain and demonstrate how to detect Mouse click input as well as many other Mouse related functions.
Setup Code
Here is the basic setup code that we will be using across this tutorial. We have just written this here, so don’t we don’t have to show it each time we demonstrate the use of a new function.
import pygame
from pygame.locals import *
import sys
pygame.init()
display = pygame.display.set_mode((300, 300))
FPS_CLOCK = pygame.time.Clock()
while 1:
for event in pygame.event.get():
if event.type == QUIT:
pygame.quit()
sys.exit()
//...
//...
pygame.display.update()
FPS_CLOCK.tick(30)
In the above code, we create the Pygame window, setup the Game and event loop as well as create an event detection for the Quit event and set up a clock as well.
Mouse Presses (Input)
This piece of code goes directly into the game loop. The get_pressed()
function returns three values, one for each button on the mouse (scroll wheel is the middle). You can either store this into a list, or use the below format to store each value in a separate variable.
left, middle, right = pygame.mouse.get_pressed()
if left:
print("Left Mouse Key is being pressed")
You can then you those values to determine which key is being pressed.
Here is the “list” way of doing it. Same concept as before.
mouse_presses = pygame.mouse.get_pressed()
if mouse_presses[0]:
print("Left Mouse Key is being pressed")
For both of the above pieces of code, as long as the mouse is being held down, it will keep printing. So you can expect several print statements, even if you just click (If you hold it down for 0.1 seconds, three print statements will execute since frame rate is 30).
The following snippet goes into the event for loop. It the same thing, but within the event loop, under the MOUSEBUTTONDOWN
event. This ensures that it only prints out the message once, regardless of how long you hold it down.
if event.type == pygame.MOUSEBUTTONDOWN:
mouse_presses = pygame.mouse.get_pressed()
if mouse_presses[0]:
print("Left Mouse key was clicked")
These are the two techniques used, one for mouse clicking and one for mouse pressing. The method you use, will depend on your requirements.
Mouse Position
Some functions related to the mouse position in Pygame.
The get_pos()
function returns the coordinates of the mouse cursor on the screen. Remember, the top left corner is (0 , 0).
print(pygame.mouse.get_pos())
The get_rel()
function returns the amount of movement in x
and y
since the previous call to this function. In other words, it gives relative movement.
print(pygame.mouse.get_rel())
If your mouse cursor is still for a while, it will return (0, 0). If you move it 5 pixels to the right, in the time between two get_rel()
calls, it will return (5, 0). Try running this code and tweaking it to see it’s effect.
Also remember that the Frame rate will effect the returned values. (lower frame rates leads to increased duration between get_rel()
calls). This is also the reason why I implemented a frame rate limit in the code for this tutorial.
Other Mouse Functions
Here are some other handy mouse related functions.
First up is the set_visible()
function, used to change the visibility of the mouse. In simpler words, you can use it to hide the mouse by passing False into it.
flag = 1
while 1:
for event in pygame.event.get():
if event.type == pygame.KEYDOWN:
if event.key == pygame.K_a:
if flag == 1:
pygame.mouse.set_visible(False)
flag = 0
elif flag == 0:
pygame.mouse.set_visible(True)
flag = 1
The above code uses a toggle system that turns the visibility on and off as you press the “A” key. Try running it out for yourself.
The below code uses a very handy feature that was introduced in Pygame 2.0. For those of you who installed Pygame before 2021, should update pygame.
if event.type == pygame.KEYDOWN:
if event.key == pygame.K_1:
pygame.mouse.set_cursor(pygame.SYSTEM_CURSOR_ARROW)
if event.key == pygame.K_2:
pygame.mouse.set_cursor(pygame.SYSTEM_CURSOR_HAND)
The above code changes the type of cursor that appears on the screen.
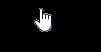
Pictured above is the cursor after pressing the Key 2. (Because pressing key 2 will call the function).
Collision with other Sprites/Rects
This is a really handy way of detecting whether the mouse is touching a sprite or not. Once again, try running the code for the optimal result.
class Player:
def __init__(self):
self.rect = pygame.draw.rect(display, (255, 0, 0), (100, 100, 100, 100))
player = Player()
while 1:
for event in pygame.event.get():
...
...
if event.type == pygame.MOUSEBUTTONDOWN:
if player.rect.collidepoint(pygame.mouse.get_pos()):
print("Mouse clicked on the Player")
if event.type == pygame.MOUSEBUTTONUP:
if player.rect.collidepoint(pygame.mouse.get_pos()):
print("Mouse released on the Player")
The only thing to not here is the collidepoint()
function, which takes a set of coordinates, and determines whether they are colliding with the rect
.
Check out this tutorial’s counterpart, Keyboard input in Pygame!
This marks the end of the Pygame Mouse Click and Events Tutorial. Any suggestions or contributions for CodersLegacy are more than welcome. Questions regarding the tutorial content can be asked in the comments section below.
Hi, I am trying to get a rect/image or sprite to follow the mouse click. How do I do this, i’m quite a novice coder. Thanks
hi you want made collision?
get mouse pos, and draw rect with x, y of mouse_pos