In this tutorial, we’re going to be improving the performance of our Pygame RPG by making various optimizations. In order to properly measure the increase in performance, we removed the FPS limit.
Now obviously our game is pretty small and simple (comparatively), so we shouldn’t be experiencing performance issues (unless we mess up horribly). There is however, no harm in making obvious optimizations so that it can work at full speed on even the most weakest of systems. It’s good practice anyway, so we should always leave some time out at the end to optimize the game.
For reference’s sake, this game was developed on a laptop with low-mid ranged specs.
Upgrade to Pygame 2.0
This game was initially developed using Pygame’s older version, which was not yet released at the time. At the end of 2020, Pygame’s long awaited version 2 was released which brought about many improvements in terms of features, fixes and performance.
pip install pygame --upgrade
The first thing I did was to update Pygame to the latest version, which immediately gave us a significant boost in FPS. An improvement of about 10 – 20% was observed.
You just need to run the above command in the command prompt and watch the magic happen.
Using Convert on Images
The real hero, and main reason for the performance jump we received was properly handling the images in Pygame.
When drawing Surfaces to the screen in Pygame, they are converted to the same pixel format as your final display. This conversion takes place every time you draw a surface, and due to being a “per-pixel” operation, it takes alot of time. To resolve this issue, when loading the images in Pygame, you should convert them using the convert()
function.
This removes the need for the conversion before drawing, which drastically improves performance. Since our game is has so many different images for every animation, we benefit greatly.
Before we were getting around 120 – 150 FPS on average (with the Pygame 2.0 update). After conversion, we were getting around 500 – 600 FPS. In short, almost 5 times the performance was observed.
As an example, below is some of the code required for importing and converting images for the running animation of the Player.
# Run animation for the RIGHT
run_ani_R = [pygame.image.load("Player_Sprite_R.png").convert_alpha(), pygame.image.load("Player_Sprite2_R.png").convert_alpha(),
pygame.image.load("Player_Sprite3_R.png").convert_alpha(),pygame.image.load("Player_Sprite4_R.png").convert_alpha(),
pygame.image.load("Player_Sprite5_R.png").convert_alpha(),pygame.image.load("Player_Sprite6_R.png").convert_alpha(),
pygame.image.load("Player_Sprite_R.png").convert_alpha()]
The reason we used convert_alpha() in the image above, is because convert() does not account for transparency. Hence, any transparent part will show up as white. You’ll find that you will almost always require convert_alpha()
.
Using 1 instead of True
A small optimization trick, that has no noticeable change (due to fluctuation in frame rate), but is an optimization nonetheless.
Instead of using the boolean True, we should use 1, which is something the computer understands natively. The boolean True, needs to be converted before the computer can understand it. Removing this in-between step can save you a few valuable operations.
while 1:
...
...
Below is an actual comparison carried out by someone, which we have attached here.
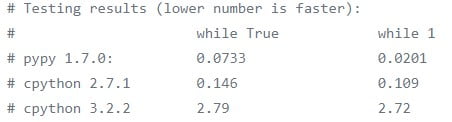
While the difference was more significant in the past, there still remains a noticeable difference between the two variants in Python 3 (the latest version).
Double buffering
An easy, one line trick that can give you a little boost in FPS, are the double buffering and Full screen flags. Simply add (or modify existing code) the following lines of code into your program.
from pygame.locals import *
flags = FULLSCREEN | DOUBLEBUF
screen = pygame.display.set_mode(resolution, flags, 16)
This will open your game in a special Full screen window where your performance will be improved. The third parameter is “bits-per-pixel” or “bpp”. Common bit-per-pixel numbers are 8, 16 and 24.
This may not suit everyone, which is why we have not included this optimization in the final game. You may turn this on if you wish however, if performance is lower than you want, or perhaps you want a full screen mode.
Further Reading
If you’re interested in learning more about how you can improve performance in Pygame, read our article on it. It has alot more tips and advice that you can use to 10 – 20x the speed of your game.
End of Series
This marks the end of our Pygame RPG Series, here on CodersLegacy. There will be another code review out where you will find the updated code from all the tutorials. There is a new and updated series we are beginning on our YouTube channel that is more up-to-date, better structured and has more features than the website version.
If you are interested, then follow that series for a better and more complete game.
This marks the end of the Pygame RPG “Improving Performance” Tutorial. Any suggestions or contributions for CodersLegacy are more than welcome. Questions regarding the tutorial content can be asked in the comments section below.