This article covers the QInputDialog in PyQt5.
Alot of GUI’s come with prompts in one or the other. One common use of such prompts or “dialogs” are for taking input from the User. PyQt5 has it’s QInputDialog widget which allows us to create a variety of different input dialogs to take input in many different ways.
Here you’ll find a list of all options and methods complete with pictures and examples.
QInputDialog – getText
We’ll start off with the most common Input Dialog, Text input. It offers a simple entry field and two buttons, OK and Cancel.
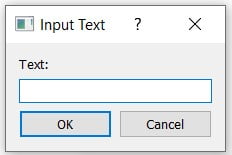
The getText()
function is a bit complicated, so we’ll examine it first. The getText()
function returns two values, hence we set up two variables to receive them. The first variable, input
receives the input of the user, the second variable, pressed
receives a True of False value stating whether the OK button was clicked or not.
input, pressed = QInputDialog.getText(parent, Title, Label, EchoMode)
Parameters for getText()
:
parent:
The main window that you created upon which this dialog is supposed to be displayed.Title:
The Heading that appears on the title bar of the dialogLabel:
The Text that appears above the entry field.EchoMode
: The echo mode that the entry field will use when receiving input.
Finally, the full code for our PyQt5 QInputDialog example is shown below.
from PyQt5 import QtWidgets
from PyQt5.QtWidgets import QApplication, QWidget, QInputDialog, QLineEdit, QPushButton, QLabel
import sys
def display():
text , pressed = QInputDialog.getText(window, "Input Text", "Text: ",
QLineEdit.Normal, "")
if pressed:
label.setText(text)
label.adjustSize()
app = QApplication(sys.argv)
window = QWidget()
window.setGeometry(400,400,250,250)
window.setWindowTitle("CodersLegacy")
label = QLabel(window)
label.setText("Empty Text")
label.move(60,80)
button = QPushButton(window)
button.setText("Press me")
button.clicked.connect(display)
button.move(60,120)
window.show()
sys.exit(app.exec_())
Unlike how we did in our previous tutorials, we’re displaying the output directly onto the GUI itself, rather than console output. We’re doing this by updating the label text with the returned text from the dialog.
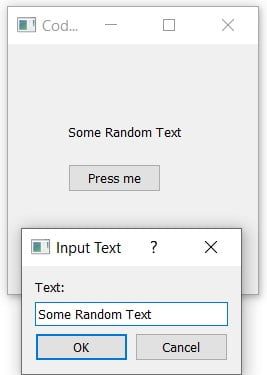
In the next examples we won’t be repeating the certain code blocks to save space and inefficiency. We’ll only post the part of the code that’s actually changing, which is the function that’s being called.
QInputDialog – getInt
Below is the getInt dialog window. You can either use the arrows to change the integer value or directly type in a value yourself.
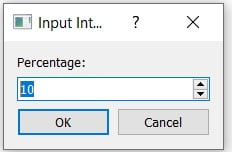
QInputDialog.getInt(parent, Title, Label, Default, Min, Max, Step)
Explanation of the Parameters in order:
parent:
The main window that you created upon which this dialog is supposed to be displayed.Title:
The Heading that appears on the title bar of the dialogLabel:
The Text that appears above the entry field.Default:
The Default value that the dialog has when it is initialized.Min:
The minimum possible value that can be selected.Max:
The maximum possible value that can be selected.Step:
The increment between values when you click the arrows.
def display():
integer , pressed = QInputDialog.getInt(window, "Input Integer","Number:",
10, 0, 100, 1)
if pressed:
label.setText(str(integer))
label.adjustSize()
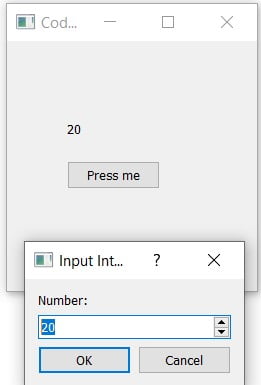
QInputDialog – getItem
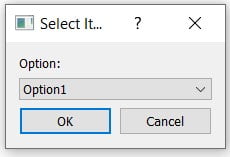
option, pressed = QInputDialog.getItem(parent, Title, Label, Options,
current, editable)
Explanation of Parameters in order:
parent:
The main window that you created upon which this dialog is supposed to be displayed.Title:
The Heading that appears on the title bar of the dialogLabel:
The Text that appears above the entry field.Options:
A List/Tuple of strings to be displayed as options.current:
The currently selected item. Takes integer values to represent index.editable:
Takes a True or False value to determine whether you can edit the options to add your own custom option.
def display():
options = ("Option1", "Option2", "Option3")
option , pressed = QInputDialog.getItem(window, "Select Item","Option:",
options, 0, False)
if pressed:
label.setText(option)
label.adjustSize()
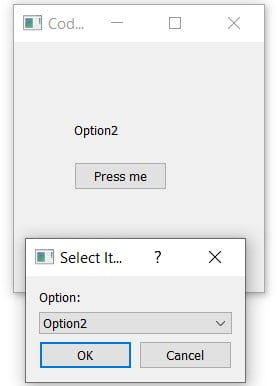
QInputDialog – getDouble
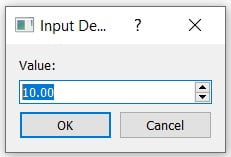
decimal, pressed = QInputDialog.getDouble(parent, Title, Label, Default, Min, Max, Step)
parent:
The main window that you created upon which this dialog is supposed to be displayed.Title:
The Heading that appears on the title bar of the dialogLabel:
The Text that appears above the entry field.Default:
The Default value that the dialog has when it is initialized.Min:
The minimum possible value that can be selected.Max:
The maximum possible value that can be selected.Step:
The increment between values when you click the arrows.
def display():
options = ("Option1", "Option2", "Option3")
decimal , pressed = QInputDialog.getDouble(window, "Input Decimal","Value:",
10.00, 0, 100, 2)
if pressed:
label.setText(str(decimal))
label.adjustSize()
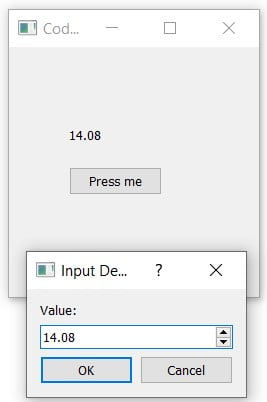
Head over to the main PyQt5 tutorial page to learn about there other great widgets and tricks.
This marks the end of the PyQt5 QInputDialog article. Any suggestions or contributions for CodersLegacy are more than welcome. Questions regarding the article content can be asked in the comments section below.