This article covers the PyQt QRadioButton widget (PyQt6)
Radio Buttons are a common occurrence in GUI applications when we need to present the user with a list of options. Only one Radio Button option can be selected by the user at any given point. Another similar widget is the PyQt6 CheckBox Widget that allows the User to select multiple options (or even none at all).
To create Radio Buttons in PyQt6, we will be using the QRadioButton Class from the QtWidgets Module. You can find a complete list of methods and options for the QRadioButton Class at the bottom of this page.
Creating a QRadioButton
Let’s start off by making just one RadioButton. We will pass some text to the QRadioButton Class, which will appear as a Label on the Radio Button. (Alternatively you can use the setText()
method to set the text after the object has been created)
from PyQt6.QtWidgets import QApplication, QWidget, QRadioButton
import sys
class Window(QWidget):
def __init__(self):
super().__init__()
self.resize(300, 250)
self.setWindowTitle("CodersLegacy")
radio = QRadioButton("Option 1", self)
radio.move(100, 100)
app = QApplication(sys.argv)
window = Window()
window.show()
sys.exit(app.exec())
The output to the above code: (Don’t forget to pass self into QRadioButton Class, otherwise the RadioButton will not be acknowledged as a child of the Window and won’t display)
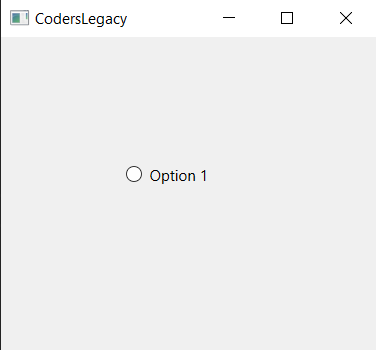
Retrieving QRadioButton values
There are two things worth retrieving from a RadioButton. The first is the text being displayed on it, and second is whether it’s current selected or not.
You can retrieve QRadioButton values using the text()
method, which will return the text value you assigned to the Radio Button. For checking it’s state you can use the isChecked()
method. This returns an integer number that represents whether the Radiobutton is selected or not.
Normally we might use these methods in a Function that is executed whenever a Button is pressed. But there is an alternative way of doing things, using PyQt6 Signals. Every time a RadioButton is pressed, it emits a “Signal Event”. We can easily bind a Function to this signal, so whenever the signal is emitted, the function will be executed. (In fact, the QPushButton uses signals as well)
We are making use of the toggled
signal, which triggers whenever the RadioButton is clicked. We will bind the showDetails()
we made to the toggled
signal using connect
. This causes the showDetails()
function to be called whenever the state of the Radio Button has been changed.
from PyQt6.QtWidgets import QApplication, QWidget, QRadioButton
import sys
class Window(QWidget):
def __init__(self):
super().__init__()
self.resize(300, 250)
self.setWindowTitle("CodersLegacy")
self.radio = QRadioButton("Option 1", self)
self.radio.toggled.connect(self.showDetails)
self.radio.move(100, 100)
def showDetails(self):
print("Selected: ", self.sender().isChecked(),
" Name: ", self.sender().text())
app = QApplication(sys.argv)
window = Window()
window.show()
sys.exit(app.exec())
The sender() function in the above code returns a reference to the object that triggered the event. Very useful when we have multiple Radio Buttons using the same function.
Below is the output of the above code in the console after we clicked the RadioButton twice, selecting and deselecting it.
Selected: True Name: Option 1
Selected: False Name: Option 1
P.S: Whatever you do, never create a method called show
, or anything else with the same name as one of the QWidget’s methods. show
is the name of a method belonging to our window, and will cause ambiguity problems.
RadioButton Groups
Radio Buttons are meant to be used in groups, not standalone. A single radio button is not very practical, as it offers just one option to the User. To create a RadioButton, we must use a grouping layout such as QGridLayout
.
In a group of radio buttons, only one radio button at a time can be checked. If the user selects another button, the previously selected radio button will be switched off.
from PyQt6.QtWidgets import QApplication, QWidget, QRadioButton, QGridLayout
import sys
class Window(QWidget):
def __init__(self):
super().__init__()
self.resize(300, 300)
self.setWindowTitle("CodersLegacy")
layout = QGridLayout()
layout.setContentsMargins(50, 80, 50, 80)
self.setLayout(layout)
self.radio = QRadioButton("Option 1", self)
self.radio.toggled.connect(self.showDetails)
layout.addWidget(self.radio)
self.radio = QRadioButton("Option 2", self)
self.radio.toggled.connect(self.showDetails)
layout.addWidget(self.radio)
def showDetails(self):
print("Selected: ", self.sender().isChecked(),
" Name: ", self.sender().text())
app = QApplication(sys.argv)
window = Window()
window.show()
sys.exit(app.exec())
Below is a GIF of the above output. It shows clearly the fact that you cannot have two of these selected at the same time.
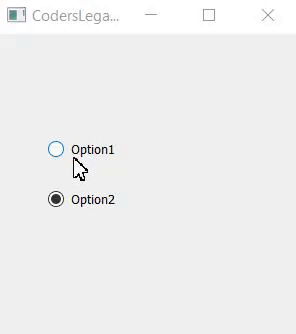
Remember to include that extra import, QGridLayout
. You can also use other Layout Managers of course, depending on what type of layout you want for your GUI Applications.
Methods Compilation
Below is a compilation of the methods available for the QRadioButton widget.
Method | Description |
---|---|
setChecked() | Changes the state of the Checkbox to checked. |
setText() | Sets the text associated with the checkbox. |
text() | Retrieves the text value of the CheckBox. |
isChecked() | Checks to see if the checkbox is selected. Returns True is selected, else False. |
This marks the end of the PyQt6 QRadioButton article. Any suggestions or contributions for CodersLegacy are more than welcome. Questions regarding the article content can be asked in the comments section below.