Tooltips are a useful feature in PyQt6 applications that provide users with additional information about certain widgets or actions. In this article, we will explore how to create a tooltip(s) in PyQt6, including how to customize them to better suit your application’s needs.
What is a Tooltip?
A tooltip is a small popup box that appears when the user hovers the cursor over a widget. The tooltip usually contains a brief description of the widget or the action that can be performed. Tooltips can provide users with additional information and improve the user experience.
Adding a Tooltip to a PyQt6 Widget
Adding a tooltip to a PyQt6 widget is pretty straightforward.
Most widgets in PyQt6, support a method called setToolTip()
, that takes a string parameter, which is the message that you want displayed in the tooltip. This method is supported on widgets which are meant to show tooltips, such as “buttons”, “input boxes”, “labels”. Widgets like the “menu” and “toolbar” to do not support tool-tips.
Here is an example of adding a tooltip to a QPushButton
widget:
from PyQt6.QtWidgets import QApplication, QPushButton, QWidget, QToolTip
import sys
class MyWindow(QWidget):
def __init__(self):
super().__init__()
self.btn = QPushButton('Click me!', self)
self.btn.setToolTip('This is a tooltip')
app = QApplication(sys.argv)
window = MyWindow()
window.show()
sys.exit(app.exec())
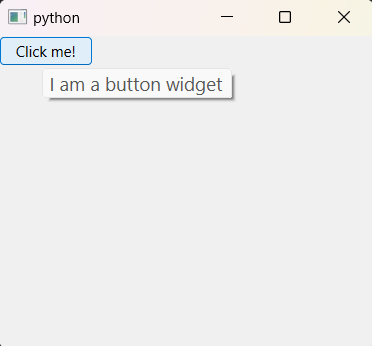
Let’s now explore some possible customization options for tooltips in PyQt6.
Customizing Tooltips in PyQt6
PyQt6 allows you to customize the appearance and behavior of tooltips. To make these changes, we must call the “static methods” belonging to the “QToolTip” class. Here are some common customizations:
Tooltip Duration
You can set the duration for which the tooltip will be displayed using the calling the setDuration()
method on any widget that supports it. By default, the duration is 5 seconds. Here is an example, where we change this default duration.
widget.setDuration(10000) # set duration to 10 seconds
Customizing Tooltip Color with CSS Stylesheets
You can change the background and foreground color of tooltips using CSS stylesheets. You can also use them to change other attributes, such as border, font-size, font-family, etc.
self.setStyleSheet("""QToolTip {
background-color: black;
color: white;
border: black solid 1px
}""")
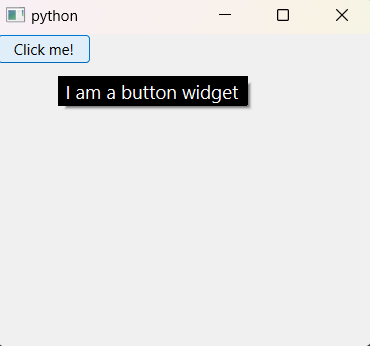
Tooltip Font using QFont()
You can change the font of tooltips using the QToolTip
static method QToolTip.setFont()
. Here is an example:
font = QFont('Arial', 16)
QToolTip.setFont(font)
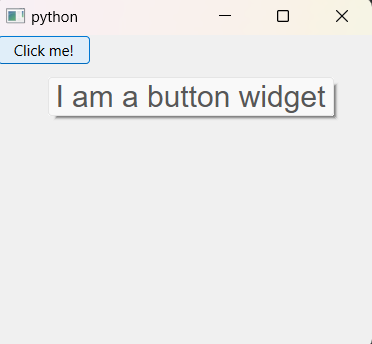
Just remember to call these static methods right in the beginning of your program, before you create any tooltips. Otherwise the new changes will not take effect.
Using HTML
We can also customize our tooltips by passing in HTML into the string. Here is an example where we use HTML to “bold” some text.
self.btn = QPushButton('Click me!', self)
self.btn.setToolTip("I am a <b>button widget</b>")
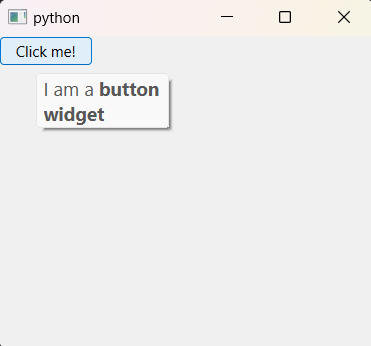
Here an another example where we use “<h>” tags.
self.btn = QPushButton('Click me!', self)
self.btn.setToolTip("<h3>I am a button widget</h3>")
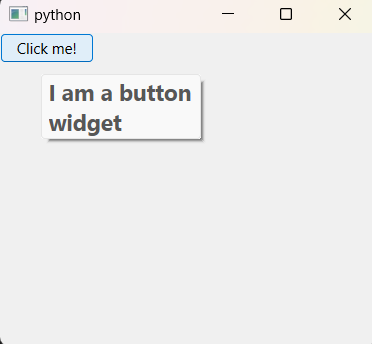
You can even combine together several HTML tags. Give it a try!
This marks the end of the PyQt6 Tooltip on Mouse hover Tutorial. Any suggestions or contributions for CodersLegacy are more than welcome. Questions regarding the tutorial content can be asked in the comments section below.