What is a Python Tkinter Radio Button?
A radio button is a Python Tkinter GUI widget that allows the user to choose only one of a predefined set of mutually exclusive options. This is in contrast to the Check Button which allows you to pick more than one.
Syntax
Rbttn = Radiobutton(master, option..........)
Below is a compilation of all the main options available for Radio Buttons.
No. | Option | Description |
---|---|---|
1 | activebackground | Color of the background when widget is under focus. |
2 | activeforeground | Color of the foreground when widget in under focus. |
3 | bg | Background color for the area around the widget. |
4 | bd | Size of the border around the widget. Default value is 2 pixels. |
5 | borderwidth | |
6 | command | If the state of this widget is changed, this procedure is called. |
7 | cursor | When the mouse is hovering over this widget, it can be changed to a special cursor type like an arrow or dot. |
8 | font | The type of font to be used for this widget. |
9 | fg | The color for the text. |
10 | highlightcolor | The text color when the widget is under focus. |
11 | image | Used to display an image |
12 | justify | Specifies how the text is aligned within the widget. One of “left”, “center”, or “right”. |
13 | padx | Amount of padding in terms of pixels |
14 | pady | Amount of padding in terms of pixels for the area above and below the widget. |
15 | relief | It specifies the type of the border. Default is Flat, other options include RAISED and SUNKEN . |
16 | State | Default is NORMAL. DISABLED causes the Widget to gray out and go inactive. ACTIVE is the state of the Widget when mouse is over it. |
17 | text | The text displayed next to the Checkbutton. |
18 | variable | The variable that stores the value of the radiobutton. This variable is shared with the other radio buttons in it’s group. |
Creating a Tkinter Radio Button
Here we’ll discuss how to create a standard Tkinter Radio Button. If you’ve done Check-buttons, the syntax is almost identical. The only difference is in their control variables.
You can create a control variable by using the IntVar()
function. You can also use StringVar()
if you wish to return a string value.
It’s important for Radio Buttons to share the same control variable. This is because only one radio button should be selected at a time (Because the control variable can only hold one value at a time). If each radio button had a unique control variable, it would be no different from a Check Button. However, to differentiate between them, they must have different values.
from tkinter import *
root = Tk()
root.geometry("200x150")
frame = Frame(root)
frame.pack()
Var1 = StringVar()
RBttn = Radiobutton(frame, text = "Option1", variable = Var1,
value = 1)
RBttn.pack(padx = 5, pady = 5)
RBttn2 = Radiobutton(frame, text = "Option2", variable = Var1,
value = 2)
RBttn2.pack(padx = 5, pady = 5)
root.mainloop()
While they have the same control variable, make sure they have different values
. You’ll realize this once you reach the next section.
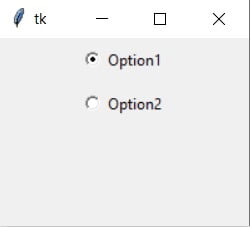
Retrieving Radio Button Values
A Radio Button returns the value assigned to it while creating it.
There are two ways to retrieve Radio Button values. The first is User-triggered. Meaning, unless the user triggers the appropriate command the values will not be returned. In this case, the User has to press the Submit button for the values to be returned.
Pressing the submit button will call a function that uses the get()
method on the control variable that the Radio Buttons share. The get method returns the value that we assigned to the option value
when creating the Radio Button.
from tkinter import *
def retrieve():
print(Var1.get())
root = Tk()
root.geometry("200x150")
frame = Frame(root)
frame.pack()
Var1 = IntVar()
RBttn = Radiobutton(frame, text = "Burger", variable = Var1,
value = 1)
RBttn.pack(padx = 5, pady = 5)
RBttn2 = Radiobutton(frame, text = "Pizza", variable = Var1,
value = 2)
RBttn2.pack(padx = 5, pady = 5)
Button = Button(frame, text = "Submit", command = retrieve)
Button.pack()
root.mainloop()
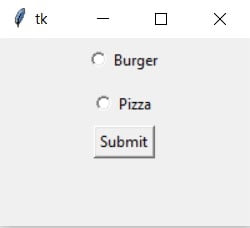
The second method involves the use of the inbuilt option within the Radio Button called command
. It functions very similar to the example above. In this case, no button is required. Every time the user changes the state of the Radio button, this command will call the appropriate Function.
from tkinter import *
def retrieve():
print(Var1.get())
root = Tk()
root.geometry("200x150")
frame = Frame(root)
frame.pack()
Var1 = IntVar()
RBttn = Radiobutton(frame, text = "Burger", variable = Var1,
value = 1, command = retrieve)
RBttn.pack(padx = 5, pady = 5)
RBttn2 = Radiobutton(frame, text = "Pizza", variable = Var1,
value = 2, command = retrieve)
RBttn2.pack(padx = 5, pady = 5)
root.mainloop()
Video Code
The Code from our Video on Tkinter RadioButtons on our YouTube Channel for CodersLegacy.
import tkinter as tk
class Window:
def __init__(self, master):
self.master = master
# Frame
self.frame = tk.Frame(self.master, width = 200, height = 200)
self.frame.pack()
# Radiobutton
self.var1 = tk.IntVar()
self.radio = tk.Radiobutton(self.frame, variable = self.var1, value = 1, text = "Option 1", command = self.submit)
self.radio.place(x = 30, y = 30)
self.radio2 = tk.Radiobutton(self.frame, variable = self.var1, value = 2, text = "Option 2", command = self.submit)
self.radio2.place(x = 30, y = 60)
def submit(self):
if self.var1.get() == 1:
print("Option 1 was selected")
elif self.var1.get() == 2:
print("Option 2 was selected")
root = tk.Tk()
root.title("Tkinter")
window = Window(root)
root.mainloop()
You can head back to the main Tkinter article using this link. Any questions about the tutorial content can be asked in the comments section below.