Often the need arises to create a Time delay in your Python program.
This is where the sleep()
function of the Time library comes in handy. Passing a number to this function will pause the execution of the program for a given amount of time. The argument may even be a floating point number to indicate a more precise sleep time.
import time
import datetime
while True:
print("This prints every 5 seconds")
print(datetime.datetime.now())
time.sleep(5) # Delay for 5 seconds.
The above code produces the following output. The output is technically never ending as there is no end clause, so this is just a portion of the output.
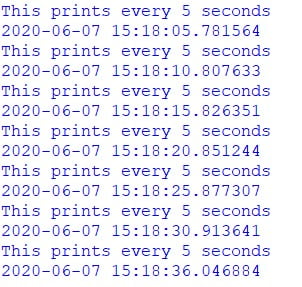
One issue associated with the sleep()
function is that it freezes the thread on which you’re program is executing. It basically puts your entire program to “sleep”. This isn’t really an issue on a smaller program, but in a larger program with many different section running simultaneously, using sleep()
for a single section will pause all the other sections as well.
You can over come this either through the use of the threading library in python, or if you’re using a GUI you can create a time delay with the tkinter after() method.
This marks the end of the Python Time Delay article. Any suggestions or contributions for CodersLegacy are more than welcome. Questions regarding the material in the article can be asked in the comments section below.