In this Matplotlib tutorial, we will discuss how to save our Matplotlib Graphs as “SVGs”. Matplotlib luckily has built-in-functionality for this that allows us to easily save graphs as an SVG, or any other type of file format (like PNGs, PDFs and JPGs).
How to create Matplotlib graphs as SVG
To convert a Matplotlib graph to an SVG, all we need to do is call the plt.savefig()
function. You need to pass the filename + its extension to this function as an argument, and it will automatically create a the SVG file in the same directory as your Python script.
plt.savefig("mySVG.svg")
Here is a complete matplotlib program which generates a graph with some text annotations and saves it as an SVG. Try it out!
import matplotlib.pyplot as plt
import numpy as np
fig, ax = plt.subplots()
x = np.arange(0.0, 4.0, 0.01)
y = np.cos(np.pi * x) * np.sin(x)
arrow = ax.annotate('Local Max',
xy = (2, 1),
xytext = (2.4, 1.4),
arrowprops = dict(arrowstyle="fancy"))
ax.set_ylim(-2, 2)
plt.plot(x, y)
plt.savefig("mySVG.svg")
plt.show()
I could not upload the SVG here, as it was not a supported filetype, so I uploaded a PNG instead to show you what the graph looks like.
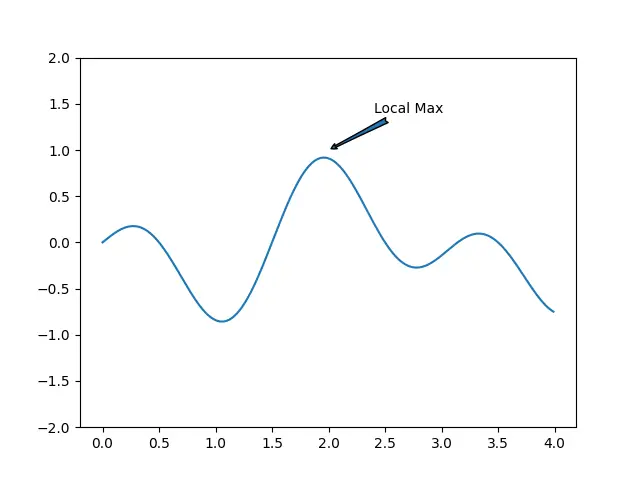
Note: The savefig() can be used on any figure as a method. e.g: fig.savefig()
. This is important if you have multiple plots open. Because plt.savefig()
is a global function that will apply the operation on the currently selected graph.
Tip: If you call plt.show()
before savefig()
, this will cause an empty white graph to be saved. This is because plt.show()
clears the graph after it is done executing.
Tip: Don’t forget that plt.show()
is a blocking function, which means that the SVG won’t be saved until you close the Window (This only happens if you put savefig() after show()).
This marks the end of the How to save Matplotlib graphs as SVG Tutorial. Any suggestions or contributions for CodersLegacy are more than welcome. Questions regarding the tutorial content can be asked in the comments section below.