In this Python Tutorial we will discuss the use of a “Set” in Python, and the various operations associated with it. By the end of this tutorial, you will know exactly what kind of operations there are available, and how to use them.
In programming, we would define sets as a unordered collection of items. No duplicates are allowed in this collection, meaning all values in unique. Furthermore, the elements in this collection are immutable, meaning that they cannot be modified after they have been added. The set itself is mutable, meaning values can be added and removed to and from it.
Creating a Set in Python
The creation of a Set is a very similar to that of a List, except with curly brackets instead of square brackets.
A Set may have any number of elements in it, any these elements can be of any type, such as integers or strings. However, sets cannot have mutable containers such as Lists or Dictionaries inside of it, as that would violate it’s properties. Let’s take a look at some examples.
Here we have a set of integers.
myset = { 1, 2, 3 }
Here we have a set of strings.
myset = { "a", "b", "c" }
And here we have a set with both strings and integers.
myset = { 1, "a", 2, "b", 3, "c" }
And since a tuple is a immutable container, it is allowed inside a set.
myset = { (1, 2, 3), ("a", "b", "c") }
To be clear, we cannot access elements from a set, as they are unordered. As their order is not defined, indexing doesn’t work either.
Python Set Operations
Now let’s move onto the various Set Operations that we can perform in Python.
Set Addition
There are two ways we can add elements to a set. The first method is with the add()
function. This function takes a single parameter which it then adds to the set.
myset = { 1, 2, 3 }
myset.add(4)
print(myset)
myset.add(5)
print(myset)
# Adding in a value that already exists
# Duplicate values will automatically be removed
myset.add(3)
print(myset)
{1, 2, 3, 4}
{1, 2, 3, 4, 5}
{1, 2, 3, 4, 5}
As you can see from the output, there is only one 3 in the set, despite us attempting to add a second 3.
The second method is through the update()
function. Unlike the add()
function, update()
function can be used add multiple values at once. It is especially useful for situations where you want to add all the elements of a list to a set.
It is important to note that update()
only takes iterable objects like Lists and Tuples. Attempting to pass a single value in will cause an error.
myset = { 1, 2, 3 }
# A list with only one value is O.K
myset.update([4])
print(myset)
myset.update([5, 6, 7])
print(myset)
# You can have multiple iterables passed at once
myset.update([8, 9], ("a", "b", "c"))
print(myset)
{1, 2, 3, 4}
{1, 2, 3, 4, 5, 6, 7}
{1, 2, 3, 4, 5, 6, 7, 8, 9, 'b', 'c', 'a'}
Sets are unordered, so you might notice that if you run this code several times, the output will be slightly be different.
Set Removal
There are two functions that we can use for the removal of elements in a set.
The first function discard(), attempts to find that element in the set. If it finds it, it will delete it. But if it does not find it in the set, it will leave the set unchanged, and not do anything.
myset = { 1, 2, 3, 4, 5 }
myset.discard(5)
print(myset)
myset.discard(2)
print(myset)
{1, 2, 3, 4}
{1, 3, 4}
The second function remove(), also does the same as discard(), but it will raise an error if the element was not found in the set.
myset = { 1, 2, 3, 4, 5 }
myset.remove(5)
print(myset)
myset.remove(5) # Raises an Error
print(myset)
{1, 2, 3, 4}
Traceback (most recent call last):
File "d:\VSCode_Programs\Python\sets.py", line 6, in <module>
myset.remove(5)
KeyError: 5
Python Set Operations
Set Operations refer to the various special operators like & and ^ that we can apply on sets. Let’s take a look at each of them individually.
Set Union
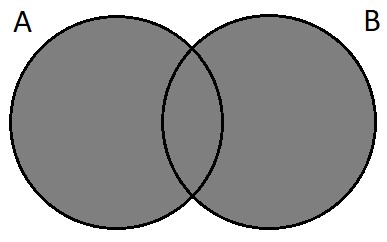
Union of two sets refers to the elements of both Set A and Set B. Basically a Set containing all values from Set A and Set B, but without any duplicates. (Like if Set A and Set B have a common value, it will only appear once in the Union set)
To perform the Union Set Operation, we use the | operator between two Sets. (also known as the pipe operator)
set1 = { 1, 2, 3 }
set2 = { 2, 3, 4, 5, 6 }
set3 = { "a", "b", "c" }
print(set1 | set2)
print(set1 | set3)
{1, 2, 3, 4, 5, 6}
{1, 2, 3, 'c', 'a', 'b'}
Alternatively, the union function can also be used on the sets, which produces the same result.
set1 = { 1, 2, 3 }
set2 = { 2, 3, 4, 5, 6 }
set3 = { "a", "b", "c" }
print(set1.union(set2))
print(set1.union(set3))
{1, 2, 3, 4, 5, 6}
{1, 2, 3, 'a', 'b', 'c'}
Set Intersection
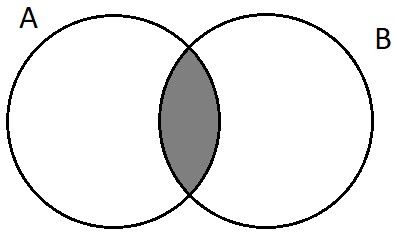
Intersection of two Sets, is the set of common elements between the two sets. To perform this operation, we can use the & operator between two Sets.
set1 = { 1, 2, 3 }
set2 = { 2, 3, 4, 5, 6 }
set3 = { "a", "b", "c" }
print(set1 & set2)
print(set1 & set3)
{2, 3}
set()
The set()
refers to an empty set, and can also be used to initialize an empty set as an alternative to { }
.
Instead of the operator, you can also use the intersection() function, which provides the same functionality.
set1 = { 1, 2, 3 }
set2 = { 2, 3, 4, 5, 6 }
set3 = { "a", "b", "c" }
print(set1.intersection(set2))
print(set1.intersection(set3))
{2, 3}
set()
Set Difference
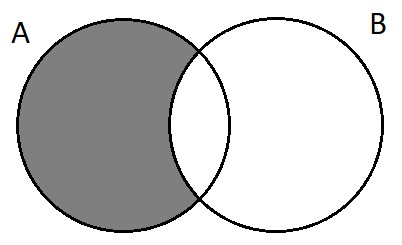
Difference of a Set A and Set B (A – B), refers to the elements that are in A, but not in B. If Set A and Set B are disjoint (have no elements in common), then A – B = A.
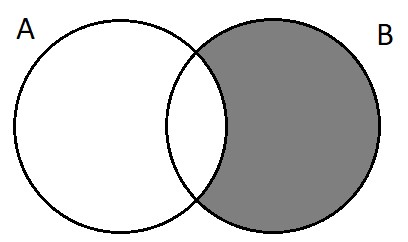
The same is also true for B – A, which produces a set of elements which are in B, but not in A.
We can perform this Set operation in Python through the use of the – (minus) operator.
set1 = { 1, 2, 3 }
set2 = { 2, 3, 4, 5, 6 }
set3 = { "a", "b", "c" }
print(set1 - set2)
print(set2 - set1)
print(set1 - set3)
{1}
{4, 5, 6}
{1, 2, 3}
Once again, as an alternative we can use the difference() function to the same effect.
set1 = { 1, 2, 3 }
set2 = { 2, 3, 4, 5, 6 }
set3 = { "a", "b", "c" }
print(set1.difference(set2))
print(set2.difference(set1))
print(set1.difference(set3))
{1}
{4, 5, 6}
{1, 2, 3}
Set Symmetric Difference
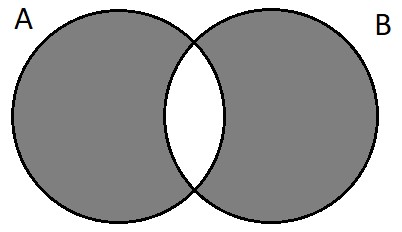
The symmetric difference is the inverse of it’s intersection, giving all the elements except those common between two Sets. We can perform the symmetric difference operation in Python using the ^ operator.
set1 = { 1, 2, 3 }
set2 = { 2, 3, 4, 5, 6 }
set3 = { "a", "b", "c" }
print(set1 ^ set2)
print(set1 ^ set3)
{1, 4, 5, 6}
{1, 2, 3, 'b', 'c', 'a'}
If there are no common elements between them, then symmetric difference of two sets will be the same as union operation.
As an alternative, the symmetric_difference() function can also be used to produce the same result.
set1 = { 1, 2, 3 }
set2 = { 2, 3, 4, 5, 6 }
set3 = { "a", "b", "c" }
print(set1.symmetric_difference(set2))
print(set1.symmetric_difference(set3))
{1, 4, 5, 6}
{1, 2, 3, 'b', 'c', 'a'}
How to find Subsets of Sets
Amongst the various functions that can be used on Sets, are the subset functions. These allow us to identify whether a set is a subset of a perfect subset of another.
The issubset() function is used to determine whether a set is a subset of another. If all elements from Set A are in Set B, then Set A is said to be a subset of Set B.
A.issubset(B)
If A is a subset of B, the above function will return True.
set1 = { 1, 2, 3 }
set2 = { 3, 4, 5 }
set3 = { 1, 2, 3, 4, 5 }
set4 = { 1, 2, 3 }
print(set1.issubset(set3))
print(set1.issubset(set4))
print(set1.issubset(set2))
print(set2.issubset(set3))
True
True
False
True
The issuperset() function is used to determine whether a set is a superset of another. It is essentially the reverse of a subset, where if all elements from Set A are in Set B, then Set B is the superset, while Set A is the subset.
A.issuperset(B)
If A is a superset of B, the above function will return True.
set1 = { 1, 2, 3 }
set2 = { 4, 5, 6 }
set3 = { 1, 2, 3, 4, 5 }
set4 = { 1, 2, 3 }
print(set3.issuperset(set1))
print(set3.issuperset(set2))
print(set1.issuperset(set4))
True
False
True
Disjoint Sets
Another useful function that we can use is the isdisjoint()
function. This can be used to determine whether two sets are disjointed or not. Two Sets are disjoint if they have no common elements between them.
set1 = { 1, 2, 3 }
set2 = { 4, 5, 6 }
set3 = { 1, 2, 3, 4, 5 }
print(set1.isdisjoint(set2))
print(set1.isdisjoint(set3))
True
False
This marks the end of the Python Set Operations Tutorial. Any suggestions or contributions for CodersLegacy are more than welcome. Questions the tutorial content can be asked in the comments section below.