In this Tkinter tutorial we will explore how to use the pack() layout function to align two Frames side by side. Layout in Tkinter can be a little tricky, especially when you have a lot of widgets in your Window. But don’t worry, in this tutorial we will explain everything and show you multiple ways of packing Frames side by side in Tkinter.
How to Align Frames in Tkinter
The procedure for this is actually pretty simple. We need to have a “parent” container into which we first put the two frames. We can either choose to use the “root” window, or create a new parent frame to hold both frames. (
Here is our implementation. All we have done is created two Frames, each with a single label widget to display text. These label widgets have been placed in the center of the frames. Each frame has the ability to expand in size (because of the expand and fill parameters).
from tkinter import *
class Window:
def __init__(self, master):
subframe = Frame(master, background="blue")
subject = Label(subframe, text = "Subject")
subject.place(relx=0.5, rely=0.5,anchor=CENTER)
subframe.pack(expand = True, fill = BOTH)
subframe2 = Frame(master, background="red")
message = Label(subframe2, text= "Message")
message.place(relx=0.5, rely=0.5,anchor=CENTER)
subframe2.pack(expand=True, fill=BOTH)
root = Tk()
root.geometry('300x200')
window = Window(root)
root.mainloop()
It is actually the default nature of Tkinter to stack frames vertically on top of each other.
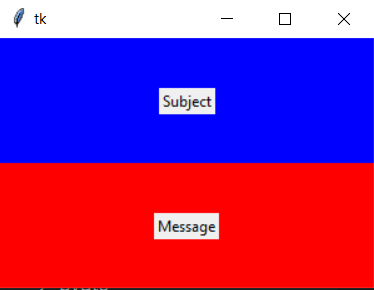
Let us now take a look at how to place them horizontally.
All we have to do, is use the “side” option that is available to the pack()
layout manager. We can pass 4 different alignment settings to this, TOP
, BOTTOM
, RIGHT
, LEFT
.
from tkinter import *
class Window:
def __init__(self, master):
subframe = Frame(master, background="blue")
subject = Label(subframe, text = "Subject")
subject.place(relx=0.5, rely=0.5,anchor=CENTER)
subframe.pack(expand = True, fill = BOTH, side=LEFT)
subframe2 = Frame(master, background="red")
message = Label(subframe2, text= "Message")
message.place(relx=0.5, rely=0.5,anchor=CENTER)
subframe2.pack(expand=True, fill=BOTH, side=LEFT)
root = Tk()
root.geometry('300x200')
window = Window(root)
root.mainloop()
And we now have our frames packed side by side!
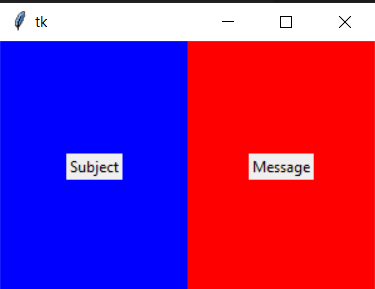
Bonus: Run the code yourself, and try passing in different parameters to the side
option to see how the alignment changes.
You expand your code to fit in even more frames, or even have a combination of both vertically and horizontally stacked frames. Here is another example.
from tkinter import *
class Window:
def __init__(self, master):
title = Label(master, text= "Title").pack()
subframe = Frame(master, background="blue")
subject = Label(subframe, text = "Subject")
subject.place(relx=0.5, rely=0.5,anchor=CENTER)
subframe.pack(expand = True, fill = BOTH, side=LEFT)
subframe2 = Frame(master, background="red")
message = Label(subframe2, text= "Message")
message.place(relx=0.5, rely=0.5,anchor=CENTER)
subframe2.pack(expand=True, fill=BOTH, side=LEFT)
subframe3 = Frame(master, background="green")
email = Label(subframe3, text= "Email")
email.place(relx=0.5, rely=0.5,anchor=CENTER)
subframe3.pack(expand=True, fill=BOTH, side=LEFT)
root = Tk()
root.geometry('300x200')
window = Window(root)
root.mainloop()
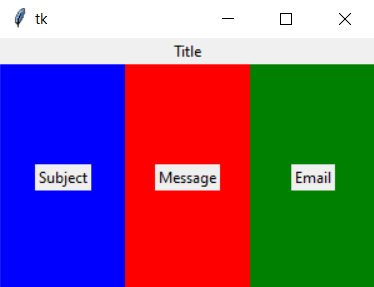
This marks the end of the How to pack two Frames side by side in Tkinter Tutorial. Any suggestions or contributions for CodersLegacy are more than welcome. Questions regarding the tutorial content can be asked in the comments section below.