In this tutorial we will discuss the wxPython FlexGridSizer Layout Sizer.
The wxPython FlexGridSizer is one of the five sizers in wxPython designed to help with the layout management of widgets in the window. The FlexGridSizer inherits from GridSizer, so it’s mostly the same. The difference is with the ability to modify rows and columns into “Growable” rows and columns which can change their size.
wxPython FlexGridSizer Example#1
In this example we’ll build a simple Form using the wxPython FlexGridSizer.
wrapper = wx.BoxSizer(wx.VERTICAL)
First we created a BoxSizer to act as a wrapper around the FlexGridSizer. The idea is to use the BoxSizer to create some padding between the widgets in the FlexGridSizer and the edges of the window. (Try it both ways, with and without the wrapper).
sizer = wx.FlexGridSizer(3, 2, 5, 5)
Next we create a FlexGridSizer, by passing in 4 parameters. The first two are for the number of rows and columns respectively. The last two parameters are for the vertical and horizontal gap (padding) between the rows and columns.
sizer.AddMany([ (wx.StaticText(panel, label = "Username")),
(wx.TextCtrl(panel), 0, wx.EXPAND),
(wx.StaticText(panel, label = "Password")),
(wx.TextCtrl(panel), 0, wx.EXPAND),
(wx.StaticText(panel, label = "Address")),
(wx.TextCtrl(panel, style=wx.TE_MULTILINE | wx.TE_NO_VSCROLL), 0, wx.EXPAND) ])
It’s now time to add widgets. We’ll use the AddMany()
to quickly add a bunch of widgets. For the TextCtrl widgets, we pass in the wx.EXPAND parameter so that it expands
sizer.AddGrowableRow(2, 1)
sizer.AddGrowableCol(1, 1)
It’s also essential (in this case), we specify that the Third row (second index), and Second column (first index) should be growable. This allows them to expand beyond their default size. The second parameter is the proportion with which they grow.
Passing in 1 into both allows them both to grow equally. Passing in 1 and 2, would cause one to grow twice as much as the other.
wrapper.Add(sizer, 1, flag = wx.ALL | wx.EXPAND, border = 15)
panel.SetSizer(wrapper)
Finally we add the FlexGridSizer object we made into the BoxSizer, along with some borders for padding purposes. Lastly, we set the BoxSizer to the Panel.
The complete code:
import wx
class Window(wx.Frame):
def __init__(self, title):
super().__init__(parent = None, title = title)
panel = wx.Panel(self)
wrapper = wx.BoxSizer(wx.VERTICAL)
sizer = wx.FlexGridSizer(3, 2, 5, 5)
sizer.AddMany([ (wx.StaticText(panel, label = "Username")),
(wx.TextCtrl(panel), 0, wx.EXPAND),
(wx.StaticText(panel, label = "Password")),
(wx.TextCtrl(panel), 0, wx.EXPAND),
(wx.StaticText(panel, label = "Address")),
(wx.TextCtrl(panel, style=wx.TE_MULTILINE | wx.TE_NO_VSCROLL), 0, wx.EXPAND) ])
sizer.AddGrowableRow(2, 1)
sizer.AddGrowableCol(1, 1)
wrapper.Add(sizer, 1, flag = wx.ALL | wx.EXPAND, border = 15)
panel.SetSizer(wrapper)
self.Centre()
self.Show()
app = wx.App()
window = Window("WxPython Tutorial")
app.MainLoop()
The output:
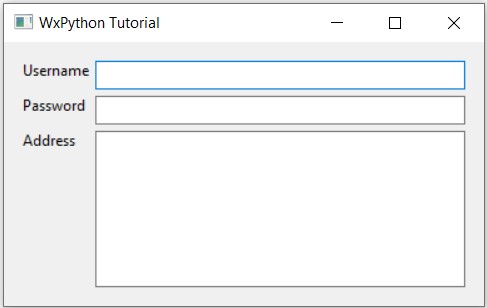
Other Sizers in wxPython
There are many other Sizers in wxPython that you can use instead of FlexGridSizer. In fact, the best layouts are often the result of combining together several different types of Sizers together. This can be done by simply nesting them into each other. There is no limitation to this ability.
- BoxSizer
- StaticBoxSizer (A variant of BoxSizer)
- GridSizer
- GridBagSizer
This marks the end of the wxPython FlexGridSizer Tutorial. Any suggestions or contributions for CodersLegacy are more than welcome. Questions regarding the tutorial content can be asked in the comments section below.