In this wxPython Tutorial, we will demonstrate how to use the RadioBox Widget, alongside it’s various styles, features and functions. A complete list of options will be included here, alongside several code examples for your convenience.
wxPython RadioBox Syntax
The Syntax required to a create a RadioBox widget in wxPython.
RadioBox(parent, id, label, pos,
size, choices, majorDimension, style,
validator, name)
parent
: This is the Widget to which it is parented, such as a Panel.id
: Widget ID. Default value is ID_ANY, which gives it the next available ID.label:
The Text which you want to display on the Widget.pos:
A tuple containing the coordinates of where the topleft corner of the StaticText Widget should begin from.size:
A tuple which defines the dimensions of the area occupied by the Text.choices:
A list of strings containing the labels for the RadioButtons. Under default circumstances, the number of strings will correspond to the number of RadioButtons created.majorDimension:
Takes an int value that specifies the maximum number of rows or columns. (Based on which style was selected)style:
Used for styling the Text (such as Alignment)
wxPython RadioBox Styles
A list of available styles for the wxPython RadioButton.
Style | Description |
---|---|
wx.RA_SPECIFY_ROWS | When this style is selected, the int passed to majorDimension refers to max rows. |
wx.RA_SPECIFY_COLS | When this style is selected, the int passed to majorDimension refers to max cols. (Default style) |
wxPython RadioBox Methods
A list of useful methods for the wxPython RadioButton.
Method | Description |
---|---|
EnableItem(int , bool ) | Enables/Disables the RadioButton at the index passed into the function. |
FindString(string ) | Searches for a RadioButton with the given string, returning the index position if found. |
GetCount() | Returns the number of items in the RadioBox |
GetColumnCount() | Returns the number of columns in the RadioBox |
GetItemLabel(int) | Gets the Label of the item at the specified index position. |
GetRowCount() | Returns the number of rows in the RadioBox |
GetSelection() | Returns the index of the currently selected item. |
GetString(int ) | Gets the String of the RadioButton at the specified index position. |
GetStringSelection() | Returns the Label of the currently selected RadioButton. |
SetSelection(int ) | Sets the current Selection to the RadioButton at the specified index. |
SetString(int , string ) | Sets the Label of the RadioButton at the given index, to the given String. |
Example Code
In this example code, we setup a RadioBox which contains 4 RadioButtons.
The first thing we do is define a list of values which will act as the labels for the RadioButtons. We have defined 4 labels in the array values
. We will then pass this array into the RadioBox()
constructor (choices
parameter).
Next we make the RadioBox widget and bind it to the wx.EVT_RADIOBOX
event and the function we made, RadioBoxEvent()
. This function uses GetStringSelection()
on the RadioBox, which returns the label of the currently selected RadioButton.
import wx
class Window(wx.Frame):
def __init__(self, title):
super().__init__(parent = None, title = title)
self.panel = wx.Panel(self)
values = ["Bread", "Butter", "Milk", "Eggs"]
self.rbox = wx.RadioBox(self.panel, label = "Radio Box", pos = (50,50),
choices = values, style = wx.RA_SPECIFY_ROWS)
self.rbox.Bind(wx.EVT_RADIOBOX, self.RadioBoxEvent)
self.label = wx.StaticText(self.panel, label = "You have selected: ", pos = (150,100))
self.Centre()
self.Show()
def RadioBoxEvent(self, event):
rboxSelection = self.rbox.GetStringSelection()
self.label.SetLabelText("You have selected: " + rboxSelection)
app = wx.App()
window = Window("WxPython Tutorial")
app.MainLoop()
This is what the RadioBox looks like with the wx.RA_SPECIFY_ROWS style.
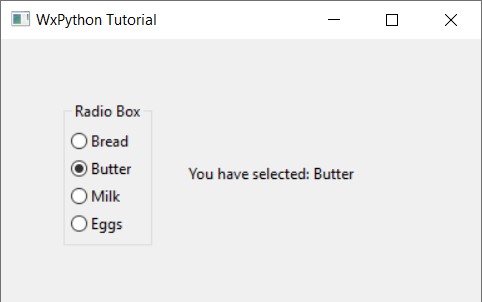
If we change this to the wx.RA_SPECIFY_COLS style….
self.rbox = wx.RadioBox(self.panel, label = "Radio Box", pos = (50,50),
choices = values, style = wx.RA_SPECIFY_COLS)
It will look like this.
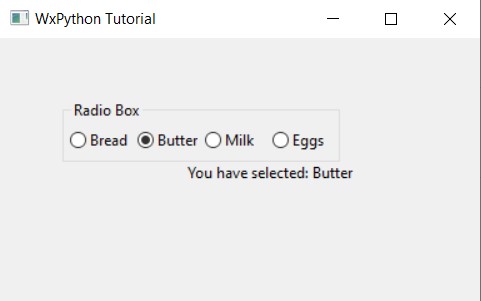
Creating a 2 Dimensional RadioBox
We can also make 2D RadioBoxes by keeping the value of the MajorDimension
lower than the number of values. If there are 4 values, and the major dimension is specified as 2 with the style wx.RA_SPECIFY_ROWS, then only two rows will be created.
Since there 4 values, they will be split equally between both rows.
self.rbox = wx.RadioBox(self.panel, label = "Radio Box", pos = (50,50),
choices = values, style = wx.RA_SPECIFY_ROWS,
majorDimension = 2)
Changing the previous code in the above manner will result in the below output.
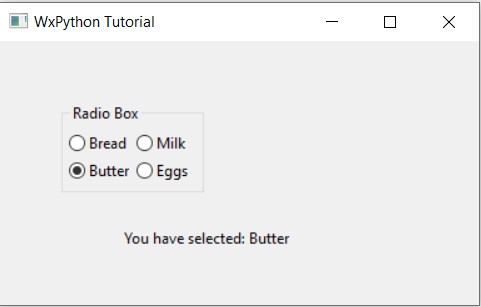
This marks the end of the wxPython RadioBox Widget Tutorial. Any suggestions or contributions for CodersLegacy are more than welcome. Questions regarding the tutorial content can be asked in the comments section below.
Frinedly doccument for wxpython, thanks.