In this wxPython Tutorial, we will demonstrate how to use the StatusBar Widget, alongside it’s various styles, features and functions. A complete list of options will be included here, alongside several code examples for your convenience.
wxPython StatusBar Styles
A list of available Styles for the wxPython StatusBar widget.
Style | Description |
---|---|
wx.STB_SIZEGRIP | Displays a gripper at the right side of the status bar used to resize the parent window. |
wx.STB_SHOW_TIPS | Displays tooltips for those panes whose status text has been ellipsized/truncated because the status text doesn’t fit the pane width. (Only available for GTK) |
wx.STB_ELLIPSIZE_START | If the Text exceeds the size of the widget, this style replaces the beginning of the Text with Ellipsis (…). |
wx.STB_ELLIPSIZE_MIDDLE | If the Text exceeds the size of the widget, this style replaces the middle of the Text with Ellipsis (…). |
wx.STB_ELLIPSIZE_END | If the Text exceeds the size of the widget, this style replaces the end of the Text with Ellipsis (…). |
wx.STB_DEFAULT_STYLE | The default style, which includes STB_SIZEGRIP| wxSTB_SHOW_TIPS| wxSTB_ELLIPSIZE_END | wxFULL_REPAINT_ON_RESIZE |
wxPython StatusBar Methods
A list of useful methods for the wxPython StatusBar widget.
Methods | Description |
---|---|
GetStatusText() | Returns the Text currently being display on the StatusBar. |
SetStatusText(string) | Sets the Text to be display on the StatusBar. |
SetMinHeight(int) | Sets the minimum height for the StatusBar (actual size may vary due to font size) |
Example Code
In this Example code, we’ll demonstrate how to create a StatusBar.
A fairly popular example/usage for the StatusBar is to display information for the widgets in the Window when a certain action is performed, such as hovering over it. In this example, we will make the wxPython StatusBar display the name of the Widget the mouse is currently hovering over.
First we created a bunch of standard widgets like the StaticText and Button, then bind them to the wx.EVT_ENTER_WINDOW
event and the onHover()
function. The wx.EVT_ENTER_WINDOW
event triggers when you enter the space occupied by that specific widget.
The onHover()
function, retrieves the Class Name of the widget that triggered the event and sets it as the text for the StatusBar.
import wx
class Window(wx.Frame):
def __init__(self, title):
super().__init__(parent = None, title = title)
self.panel = wx.Panel(self)
self.status = self.CreateStatusBar()
text = wx.StaticText(self.panel, label= "Hello World", pos=(50, 50))
button = wx.Button(self.panel, label = "Press Me", pos=(200, 50))
rb = wx.RadioButton(self.panel, label = "Pizza", pos=(50, 120))
cb = wx.ComboBox(self.panel, value = "Kittens", pos=(200, 120))
self.panel.Bind(wx.EVT_ENTER_WINDOW, self.onHover)
text.Bind(wx.EVT_ENTER_WINDOW, self.onHover)
button.Bind(wx.EVT_ENTER_WINDOW, self.onHover)
rb.Bind(wx.EVT_ENTER_WINDOW, self.onHover)
cb.Bind(wx.EVT_ENTER_WINDOW, self.onHover)
self.Centre()
self.Show()
def onHover(self, e):
name = e.GetEventObject().GetClassName()
self.status.SetStatusText(name + " Widget")
app = wx.App()
window = Window("WxPython Tutorial")
app.MainLoop()
The output of the above code, while hovering over the Button.
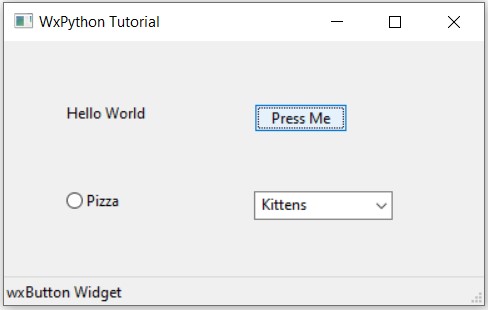
self.CreateStatusBar()
is a Frame method, used as an alternative way of creating the StatusBar. You can create it using the normal way as well, using wx.StatusBar()
.
This marks the end of the wxPython StatusBar Widget Tutorial. Any suggestions or contributions for CodersLegacy are more than welcome. Questions regarding the tutorial content can be asked in the comments section below.