In this wxPython Tutorial, we will demonstrate how to use the ToggleButton Widget, alongside it’s various styles, features and functions. A complete list of options will be included here, alongside several code examples for your convenience.
wxPython ToggleButton Syntax
The syntax structure required to create a ToggleButton Widget in wxPython.
ToggleButton(parent, id, label, pos,
size, style, val, name)
parent
: This is the Widget to which it is parented, such as a Panel.id
: Widget ID. Default value is ID_ANY, which gives it the next available ID.label:
The Text which you want to display on the Widget.pos:
A tuple containing the coordinates of where the topleft corner of the StaticText Widget should begin from.size:
A tuple which defines the dimensions of the area occupied by the Text.style:
Used for styling the Button.
wxPython ToggleButton Methods
A list of useful methods for the ToggleButton Widget.
Method | Description |
---|---|
GetValue() | Returns a bool (True/False) to reflect the current state of the ToggleButton. |
SetValue(bool) | Sets the ToggleButton to the state mentioned in the parameters. |
SetLabel(string) | Sets the label on the ToggleButton to the given String. |
GetString() | Returns the string value of the Label on the ToggleButton. |
Example Code
A simple example where we create two ToggleButton Widgets, and set one of them to the On state.
import wx
class Window(wx.Frame):
def __init__(self, title):
super().__init__(parent = None, title = title)
self.panel = wx.Panel(self)
toggle1 = wx.ToggleButton(self.panel, label ="Off", pos = (50, 50))
toggle2 = wx.ToggleButton(self.panel, label ="On", pos = (150, 50))
toggle2.SetValue(True)
self.Centre()
self.Show()
app = wx.App()
window = Window("WxPython Tutorial")
app.MainLoop()
The output, where you can see both the two ToggleButton’s in different states.
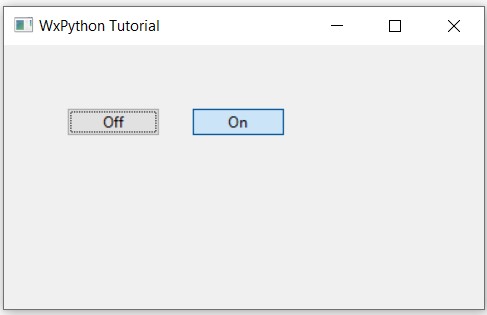
ToggleButton Events and Function Example
In this example we’ll take a look at how to Bind a ToggleButton to a function that triggers when it is pressed.
The Code below binds a ToggleButton to the ToggleButtonEvent()
function. This function changes the text of the toggleButton, depending on what it’s current value is. In short, clicking it while it’s off, will change the text to “On”.
Run the code for yourself to try it out.
import wx
class Window(wx.Frame):
def __init__(self, title):
super().__init__(parent = None, title = title)
self.panel = wx.Panel(self)
toggle = wx.ToggleButton(self.panel, label ="Off", pos = (50, 50))
toggle.Bind(wx.EVT_TOGGLEBUTTON, self.ToggleButtonEvent)
self.Centre()
self.Show()
def ToggleButtonEvent(self, e):
toggleButton = e.GetEventObject()
if (toggleButton.GetValue() == True):
print("turning on")
toggleButton.SetLabel("On")
else:
print("turning off")
toggleButton.SetLabel("Off")
app = wx.App()
window = Window("WxPython Tutorial")
app.MainLoop()
This marks the end of the wxPython ToggleButton Widget Tutorial. Any suggestions or contributions for CodersLegacy are more than welcome. Questions regarding the tutorial content can be asked in the comments section below.