In this tutorial we will explore how to create a simple toolbar in wxPython. A toolbar is handy little menu that appears on your Window with a bunch of icons which act as buttons. These icons are linked to functions, such as a Save Icon triggering a Save function.
Overall the ToolBar is an important widget, which will add alot of flavor and user-friendliness into your wxPython Window.
wxPython ToolBar Syntax
There are two ways of creating a ToolBar in wxPython. You can either use the CreateToolBar()
method on the frame, or create the ToolBar using conventional methods.
ToolBar(parent, id, pos, size, style, name)
The first method is more recommended however, as it helps shorten your code and looks simpler. We’ll be using this method later in our examples.
wxPython ToolBar Styles
A list of available styles for the wxPython Toolbar.
Style | Description |
---|---|
wx.TB_FLAT | Makes the ToolBar look Flat |
wx.TB_HORIZONTAL | Makes the layout horizontal (default) |
wx.TB_VERTICAL | Makes the layout Vertical |
wx.TB_TEXT | Shows Text on the Toolbar (only icons shown by default) |
wx.TB_NOICONS | Disables Icons on the ToolBar |
wx.TB_HORZ_LAYOUT | Puts Icons and Text side by side, instead of vertically. |
wx.TB_HORZ_TEXT | A combination of the TB_HORZ_LAYOUT and TB_TEXT styles. |
wx.TB_NO_TOOLTIPS | Disables Tooltips which appear on mouse hover. |
wx.TB_BOTTOM | Aligns ToolBar at the bottom. |
wx.TB_RIGHT | Aligns ToolBar to the Right. |
wxPython ToolBar Methods
A list of useful methods for the wxPython ToolBar.
Method | Description |
---|---|
AddTool(toolid, label, Bitmap) | Adds a Tool to the Toolbar. |
AddCheckTool(toolid, label, Bitmap) | Adds a Check Tool to the ToolBar. |
AddControl(widget) | Adds as a Tool a widget which inherits from wx.Control (e.g: ComboBox) |
AddRadioTool(toolid, label, Bitmap) | Adds a Radio Tool to the ToolBar. |
AddSeparator() | Adds a Separator to the ToolBar (for grouping of tools) |
ClearTools() | Deletes all Tools in the ToolBar. |
DeleteTool(toolid) | Deletes the Tool completely. |
EnableTool(toolid, bool) | Used to Enable/Disable the Tool. |
InsertTool(pos, toolid, label, Bitmap) | Inserts a tool at the specified index position in the Toolbar. |
InsertControl(pos, toolid, label, Bitmap) | Inserts a Control tool at the specified index position in the Toolbar. |
InsertSeparator(pos) | Inserts a separator at the specified index position in the Toolbar. |
Realize() | Must be called after you have added tools into the Toolbar. |
RemoveTool() | Removes the Tool from the ToolBar, but doesn’t delete it. (can be re-used) |
ToggleTool(toolid, bool) | Toggles the Tool On/Off. |
Example Code
A small example showing how to create a ToolBar, add in some Icons and an image showing what it all looks like.
We’ve used the CreateToolBar()
method to create a Toolbar, to which we add a few “Tools”. We’ve added two simple tools using the AddTool()
method.
import wx
class Window(wx.Frame):
def __init__(self, title):
super().__init__(parent = None, title = title)
self.panel = wx.Panel(self)
toolbar = self.CreateToolBar()
# Tool 1
toolbar.AddTool(wx.ID_ANY, "Open",
wx.Bitmap("Open.bmp"))
# Tool 2
toolbar.AddTool(wx.ID_ANY, "Save",
wx.Bitmap("Save.bmp"))
toolbar.Realize()
self.Centre()
self.Show()
app = wx.App()
window = Window("WxPython Tutorial")
app.MainLoop()
The output of the above code:
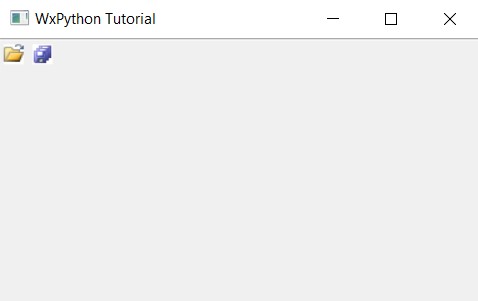
Adding Check Tools
In this example we will add a Check tool in our ToolBar.
The CheckTool()
method is used the same way as AddTool()
, but it adds some extra functionality. The Tool that is created adds like a CheckButton. When you click it once, it will be highlighted with a blue color and turn “on”. Clicking it again will turn it off.
Remember to call the Realize()
method in the end, otherwise the tools will not show up on the ToolBar.
import wx
class Window(wx.Frame):
def __init__(self, title):
super().__init__(parent = None, title = title)
self.panel = wx.Panel(self)
toolbar = self.CreateToolBar()
# Tool 1
toolbar.AddTool(wx.ID_ANY, "Open",
wx.Bitmap("Open.bmp"))
# Tool 2
toolbar.AddTool(wx.ID_ANY, "Save",
wx.Bitmap("Save.bmp"))
# Tool 3
toolbar.AddCheckTool(wx.ID_ANY, "Highlight",
wx.Bitmap("Highlight.bmp"))
toolbar.Realize()
self.Centre()
self.Show()
app = wx.App()
window = Window("WxPython Tutorial")
app.MainLoop()
The output, where we have selected the Highlight tool option.
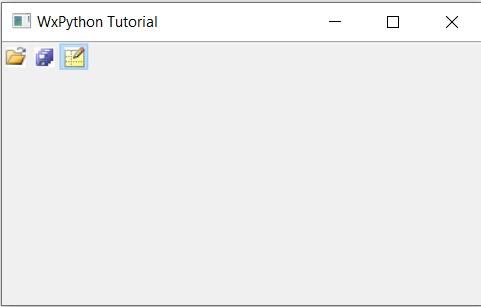
Adding Control Tools
In this example we will add a Control tool in our ToolBar.
The control widget must first be created (such as a ComboBox), and parented to the Toolbar. It can then be added to the ToolBar using the AddControl()
method.
import wx
class Window(wx.Frame):
def __init__(self, title):
super().__init__(parent = None, title = title)
self.panel = wx.Panel(self)
toolbar = self.CreateToolBar()
# Tool 1
toolbar.AddTool(wx.ID_ANY, "Open",
wx.Bitmap("Open.bmp"))
# Tool 2
toolbar.AddTool(wx.ID_ANY, "Save",
wx.Bitmap("Save.bmp"))
# Tool 3
toolbar.AddCheckTool(wx.ID_ANY, "Highlight",
wx.Bitmap("Highlight.bmp"))
# Tool 4
combo = wx.ComboBox(toolbar, choices=["Option1","Option2"])
toolbar.AddControl(combo)
toolbar.Realize()
self.Centre()
self.Show()
app = wx.App()
window = Window("WxPython Tutorial")
app.MainLoop()
The output of the above code:
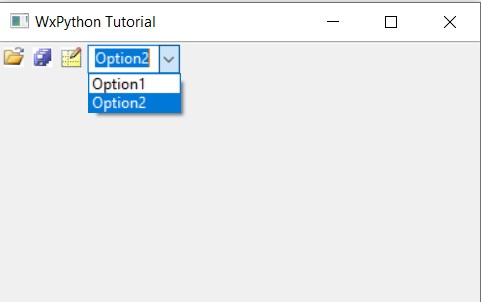
This marks the end of the wxPython Making a ToolBar Tutorial. Any suggestions or contributions for CodersLegacy are more than welcome. Questions regarding the tutorial content can be asked in the comments section below.
hello, Coderlegacy, I am watching your Chanels on YouTuBe, and I have a question, How to set an action on a tool of toolbar that shows the function of the tool when the mouse enter on the icon of the tool. I known that there is a function SetToolTip for a button, but how to set it for a tool? thank you very much!