In this Tkinter Tutorial we will discuss how to create and use the ScrolledText Widget. This widget is used to display large amounts of text, where the area occupied by the text is greater than the actual size of the widget. We can then access the rest of the text using the ScrolledText Widget’s “scrolling” features.
This widget is actually just a “convenience” widget just is a combination of the Text widget and Scrollbar widget. It’s an easier and faster way to setup this combination.
Creating ScrolledText in Tkinter
Let’s start off with a simple example.
The scrolledtext widget is not stored where the other widgets are, and must be imported a little differently. There is a scrolledtext module in Tkinter, which contains a Class called ScrolledText. We will import this Class to create our widget.
import tkinter as tk
from tkinter.scrolledtext import ScrolledText
We can define certain attributes about our ScrolledText widget, such as its width and height (in characters). Any of the three layout managers can be used on this widget (pack, grid and place). Regardless of the Layout Manager you use, it’s almost always a good idea to use the fill=tk.BOTH
and expand=True
to allow the Widget to expand and fill any available space.
import tkinter as tk
from tkinter.scrolledtext import ScrolledText
root = tk.Tk()
root.title("ScrolledText Widget")
scrolltext = ScrolledText(root, width=50, height=10)
scrolltext.pack(fill=tk.BOTH, side=tk.LEFT, expand=True)
root.mainloop()
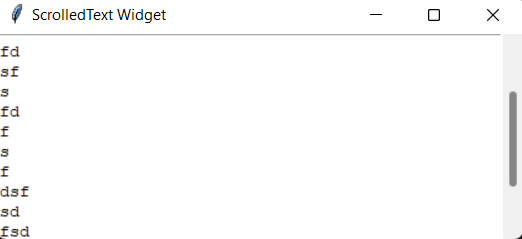
The image above shows the output after we type in some random characters and scroll down a bit using the scrollbar.
We can insert data into the ScrolledText widget through code. This can be done using the insert()
method.
The first parameter is an index, like tk.INSERT
(index of the current location of insert cursor) or tk.END
(insert at the end). Or you can just directly pass in the index, like ‘1.3’, which means “line 1, character 3”.
scrolltext.insert(tk.INSERT,
"""\
Lorem ipsum dolor sit amet, consectetur adipiscing elit.
Mauris nisi odio, volutpat quis consequat ut, suscipit et tellus.
Mauris vel enim mattis, facilisis ante ut, pretium lectus.
In fringilla ante nec elit congue, mattis vehicula tellus tempor.
Duis id massa volutpat, bibendum erat non, dictum libero.
Maecenas justo sem, lacinia sed convallis at, elementum eget velit.
Duis ullamcorper dolor felis, ut dictum lectus aliquam vitae.
Fusce et sapien in urna lacinia faucibus.
""")
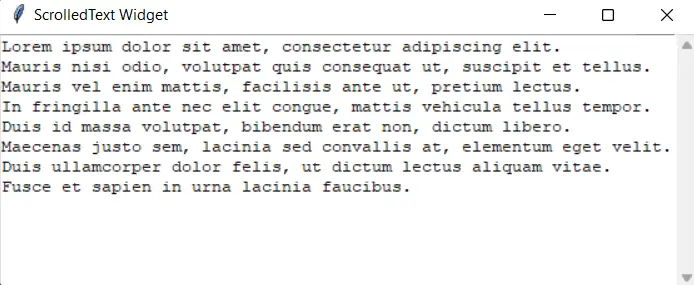
Other methods
Another cool thing we can do is disable the widget, preventing people from writing to it manually, making it read-only.
scrolltext.configure(state ='disabled')
You can also “delete” text in the same manner that you add it.
scrolltext.delete('1.5', '1.10')
Removes the text between the fifth and tenth characters in the first line.
Tip: If you are having trouble finding help about the ScrolledText widget online, you should know this Class inherits from the Text widget. This means all methods that work on the Text widget, will work here. This Class also has the scrollbar as a variable called “vbar” that you can access. (Like object.vbar
)
This marks the end of the Tkinter ScrolledText Widget Tutorial. Any suggestions or contributions for CodersLegacy are more than welcome. Questions regarding the tutorial contents can be asked in the comments section below.