Tkinter, being the large and expansive GUI library that it is, offers us a wide range of widgets to take input in Python. One of these many widgets is the Tkinter Text Widget, which can be used to take multiline input. It also has a ton of amazing features that allow us to create complex GUI applications such as Notepad’s and Syntax Highlighters.
Another very popular alternative is the Tkinter Entry widget, used to take single line input. For most cases, it will be your preferred choice of input.
Creating the Text Widget
We’ve added in some extra parameters such as Height and Width for a custom size. We have also passed in expand = True
and fill = tk.BOTH
parameters into the frame and text widget, so that if we resize the window the size of the Text widget will expand in “both” the X and Y direction to adjust accordingly.
import tkinter as tk
class Window:
def __init__(self, master):
self.master = master
self.frame = tk.Frame(self.master)
self.frame.pack(expand = True, fill = tk.BOTH)
self.label = tk.Label(self.frame, text = "My NotePad")
self.label.pack()
self.text = tk.Text(self.frame, undo = True, height = 20, width = 70)
self.text.pack(expand = True, fill = tk.BOTH)
root = tk.Tk()
window = Window(root)
root.mainloop()
Below is the output of the above code:
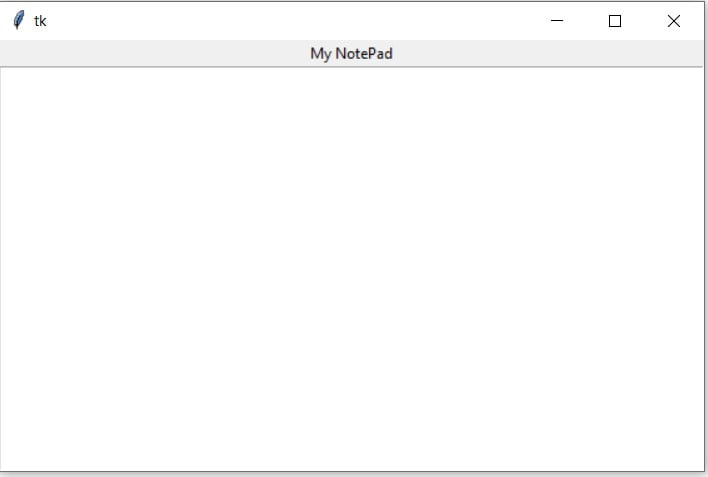
Indexes
The first thing you need to do, is to understand the indexing system in the Text widget. "1.0"
for instance, refers to “Line 1” and “character 1”. So character 1 on line 1. (And yes, remember that indexing starts from zero, but that only applies on the characters, not the lines). Similarly, "3.7"
refers to Line 3, character 8.
There are a bunch of other words we can use, like "end"
which means the last character in the Text widget. There is also linestart
and lineend
, which give us the first and last character respectively of a line. "2.4 linestart"
will return the index of the first character on line 2.
Insert Text
Using the insert()
method on the text widget we created, allows us to directly insert text at a specified index. It takes two parameters, an index and the data to be inserted.
self.text.insert("1.0", "This is some Sample Data \nThis is Line 2 of the Sample Data")
As you can see in the code above, and the image below, you can add newline characters to ensure proper formatting as well.
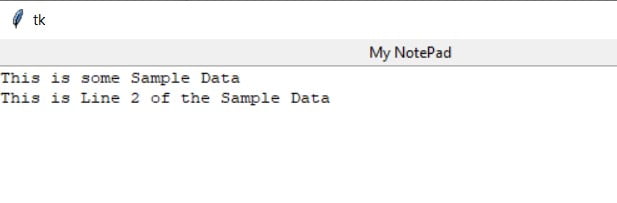
Here is another example of inserting some data. This code will add the text to the end of the Text widget.
self.text.insert("end", "\nThis is some Sample Data \nThis is Line 2 of the Sample Data")
Retrieve Text
Since we can insert text into widgets, it makes sense for us to be able to retrieve them as well. Using the get() function, we can retrieve text by specifying a starting index and a ending index. All the data between those two points will be returned.
self.text.get("1.0", "end - 1 chars")
The code above returns all the text in the tkinter widget, except for the last newline character. Since the last character in the text widget is a newline character, doing end - 1 chars
will give us the position of the character before the newline character. (You don’t have to do this, I just wanted to demonstrate this capability)
self.text.get("1.0", "end - 1 lines")
Similarly, the above code returns all of the text in the text widget, with the exception of the last line.
self.text.get("1.0", "1.0 lineend")
The above code returns the first line of text in the text widget.
self.text.get("1.0", "1.0 + 3 lines")
The above code returns the first 3 lines of code in the text widget.
Delete Text
If you’ve been paying attention, you’ll realize immediately that the below code uses the delete()
function to remove all the text in the text widget.
self.text.delete("1.0", "end")
Here is another interesting way of using indexes in Text widget. Imagine that you have the index of the first character of a word, and you want to delete the whole word. Now, you don’t know the full size of the word, and thus can’t specify the end range.
This is where the wordstart
and wordend
features comes in. Just like end
, it will automatically find the start and end of the word.
self.text.delete("1.3 wordstart", "1.3 wordend")
The above code will delete the whole word, situated at the index 1.3 (line 1, character 3).
Adding Images
With a combination of the Tkinter PhotoImage and image_create()
functions, we can load, create and insert an image into the Tkinter Text widget.
self.img = tk.PhotoImage( file = "crown.png")
self.text.image_create("end", image = self.img)
In the code above, we load up the "crown.png"
, and insert it at the end of the text widget.
self.img = tk.PhotoImage( file = "crown.png")
self.text.image_create(tk.INSERT, image = self.img)
Here is another very interesting piece of code which uses the tk.INSERT
index. The unique thing about this is that it returns the index of the “Insert” cursor. (You can see it in the image below, near the top). Wherever this cursor of yours is, it will insert the image there.
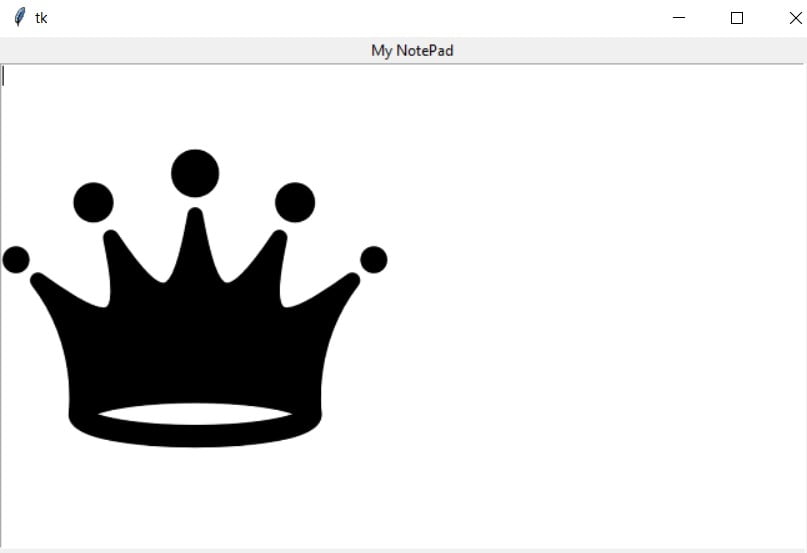
Adding Scrolling
Another handy thing we can do is add a scrollbar to the Text widget. This is actually part of the Tkinter ScrollBar tutorial, but I decided to include this here too.
class Window:
def __init__(self, master):
self.master = master
self.frame = tk.Frame(self.master)
self.frame.pack(expand = True, fill = tk.BOTH)
self.label = tk.Label(self.frame, text = "My NotePad")
self.label.pack()
# Creating ScrollBars
self.scrolly = tk.Scrollbar(self.frame)
self.scrolly.pack(side = tk.RIGHT, fill = tk.Y)
self.scrollx = tk.Scrollbar(self.frame, orient = tk.HORIZONTAL)
self.scrollx.pack(side = tk.BOTTOM, fill = tk.X)
# Creating Text Widget
self.text = tk.Text(self.frame, wrap = tk.NONE, undo = True, yscrollcommand = self.scrolly.set,
xscrollcommand = self.scrollx.set)
self.text.pack(expand = True, fill = tk.BOTH)
# Config the Scrollbars
self.scrolly.config(command = self.text.yview)
self.scrollx.config(command = self.text.xview)
If you want an explanation for the above code, just refer to the Scrollbar tutorial, otherwise the output is shown below.
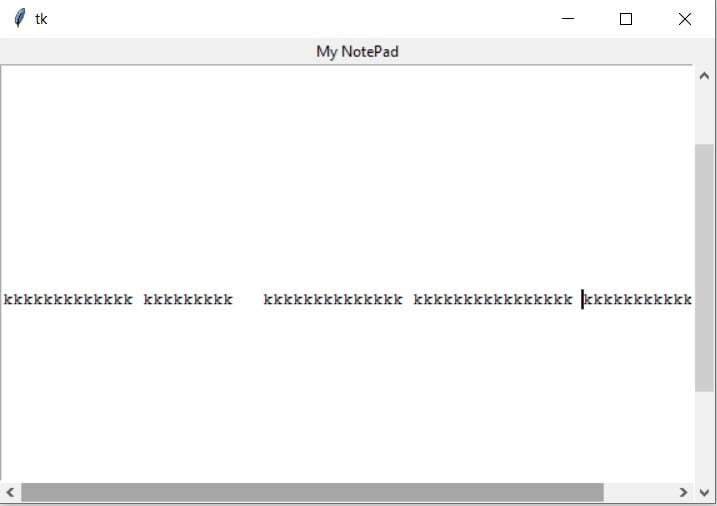
Video
Here, we have a short video series on the Tkinter Text Widget. It’s great if you want more depth and demonstration on the various options and code that we discussed in this tutorial. It’s also great for understanding how exactly we can use the Tkinter Text widget in real life applications.
Be sure to check out the last video, where we create an actual Syntax Highlighter using the Tkinter Text Widget.
List of Options
A complete list of options availible for the Tkinter Text Widget.
No. | Option | Description |
---|---|---|
1. | bg | Background Color of the Widget |
2. | bd | Border size of the Widget in pixels. Default is 2. |
3. | cursor | The type of cursor that will appear when hovering over the widget. |
4. | exportselection | Boolean value. If set, the widget selection is linked to the Window Manager selection |
5. | font | Used to specify what font the text is to be displayed in. |
6. | fg | Foreground color. In this case, the color of the text. |
7. | height | Height of the Text Widget in terms of “lines”. (not pixels) |
8. | highlightbackground | The color of the focus highlight when the text widget does not have focus. |
9. | highlightcolor | The color of the focus highlight when the text widget has the focus. |
10. | highlightthickness | The thickness of the focus highlight. Default is 1. |
11. | padx | padding to the left and right of the text. |
12. | pady | padding above and below the text. |
13. | relief | It specifies the type of the border. Default is Flat, other options include RAISED and SUNKEN . |
14. | spacing1 | Used to set amount of vertical space above a line of text. Default is 0. |
15. | spacing2 | Used to set amount of vertical space between two warped lines. Default is 0. |
16. | spacing3 | Used to set amount of vertical space below a line of text. Default is 0. |
17. | state | One of “NORMAL, “READONLY”, or “DISABLED”. Normal is the default state. Readonly prevents the user from editing any values. disabled shuts down the widgets and greys it out. |
18. | width | Width of the Text Widgets in terms of characters. |
19. | wrap | Use WORD, CHAR or NONE to enable different kinds of wrapping modes. WORD breaks the line around the word, whereas CHAR breaks it around characters. |
20. | yscrollcommand | Allows the user to scroll vertically. Assign to this, the set method of a vertical Tkinter scrollbar. |
21. | xscrollcommand | Allows the user to scroll horizontally. Assign to this, the set method of a horizontal Tkinter scrollbar. |
Text Methods
Being a larger than usual widget, the Tkinter Text has many functions that you can use on it.
No. | Method | Description |
---|---|---|
1. | delete( startindex, endindex) | Deletes all text in between the two indexes in the parameters. |
2. | get(startindex, endindex) | Retrieves all text in between the two indexes in the parameters. |
3. | index( index ) | Returns the absolute value of an index. |
4. | insert(index, string) | Inserts a string at the specified index. |
5. | see(index) | Used to check if the text at the given index, is visible or not. |
Tag related methods
Tags are in important part of the Text Widget, and have their own sets of methods dedicated to them.
No. | Method | Description |
---|---|---|
1. | tag_add(taganme, startindex, [endindex]....) | Adds a tag at either the startindex given, or between the two index (starting and ending). |
2. | tag_config() | You can use this to configure the tags, and change basic properties such as background, foreground, justify etc. |
3. | tag_delete(tagname) | Takes a regular string as input, and deletes the corresponding tag. |
4. | tag_remove(taganme, startindex, [endindex]....) | This removes the effect of the tag from either the given startindex, or the range between the start and end index. (It does not delete the actual tag). |
This marks the end of the Tkinter Text Widget Tutorial. Any suggestions or contributions for CodersLegacy are more than welcome. Questions regarding the tutorial content can be asked in the comments section below.