This article explains how to use and manipulate strings in C++.
Strings are an integral part of any programming language due to their ability to store text. In C++, there are dozens of different methods and functions that can be used to manipulate these strings. We’ll be explaining how to create strings and use these methods on them in this C++ string tutorial.
Creating a String in C++
There are two steps to creating a string in C++. First you must import the string library by including an additional header file with the following line.
#include <string>
Next you actually have to declare the string. You can choose to simply declare a string variable, or declare it with an initial value as well. Both ways are shown below.
Without a value:
string name;
With a value:
string name = "Coder";
Accessing Strings
Just like other programming languages, indexing in C++ starts from zero. What this means is that the “index” or “position number” assigned to to first character in a string is not 1, it is 0.
Below is a simple diagram we came up with to demonstrate this effect.
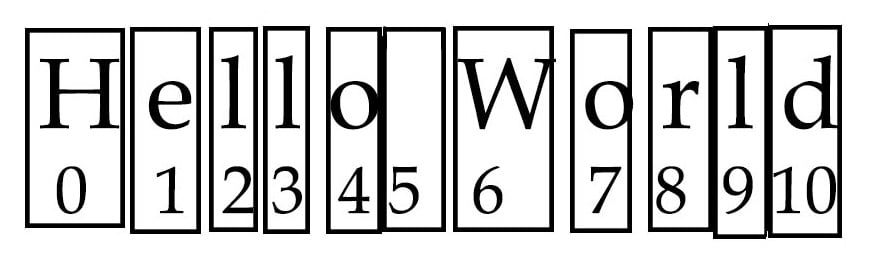
Learning how to access strings correctly has a large number of benefits. Indexing plays a huge role in retrieving individual characters from strings, locating substrings, iterating over strings etc.
Accessing individual characters
#include <iostream>
#include <string>
using namespace std;
int main () {
string x = "Hello World";
cout << x[0];
}
H
cout << x[6];
W
cout << x[8];
r
Iterating over a String
Using a for loop, you can easily iterate over a string. You may not see the use of this right now, but later it will help in fields like data analysis (like locating substrings) and such.
There’s more than one way of doing this, but we’ve chosen the following method for it’s simplicity and small size.
First we create a string called str
with some random text. Next we create a for loop and declare a variable of datatype of char called c
. The string we declared earlier is separated from the char data type by :
.
With this format, every iteration of the for loop will load a new character from the string (str
) into the variable c
. Adding a simple cout
line that prints the value of c each iteration shows us this perfectly.
#include <iostream>
#include <string>
using namespace std;
int main() {
string str = "Hello";
for(char& c : str) {
cout << c;
cout << "\n";
}
}
H
e
l
l
o
String Concatenation
String Concatenation refers to the process where two or more strings are combined together into one. There are two ways of doing so in C++ that we’ll discuss below.
The simpler way of doing so is just to use the +
between two strings (or more) to combine them.
#include <iostream>
#include <string>
using namespace std;
int main () {
string x, y;
cout << "Enter string x: ";
cin >> x;
cout << "Enter string y: ";
cin >> y;
cout << x + y;
}
Enter string x: hello
Enter string y: world
helloworld
The other way is to use the append()
method which is the more “proper” way of concatenating strings in C++.
int main () {
string x, y;
cout << "Enter string x: ";
cin >> x;
cout << "Enter string y: ";
cin >> y;
cout << x.append(y);
}
Enter string x: C++
Enter string y: strings
C++strings
Converting Strings (and Vice versa)
In this section we’ll explain how to convert strings to other data types, as well as converting other data types to strings. Converting strings to numbers is the most common, so we’ll cover the conversion to both integer and decimal values.
Using string streams from the sstream library we make these conversions possible. First step is to create a stream object from the string you wish to convert. Next you just have “stream” it to the variable x by using the >>
operator.
#include <iostream>
#include <sstream>
using namespace std;
int main()
{
string text = "123";
stringstream stream(text);
int x;
stream >> x;
cout << "Value of x : " << x;
}
123
The value is just the same, but of a different type. You can use method to convert to integer, double, float and other number related data types.
Other methods
A few other useful things you can do with strings in C++ using methods.
substr(n1, n2)
The substr()
method is meant to locate sub strings within a string. It takes two arguments as input, a start index and a stop index. Everything in between (including the start index) is returned as a sub string (excluding the stop index).
int main () {
string x = "Hello World";
cout << x.substr(0,5);
}
Hello
size method
A simple but important function that returns the size or “length” of the string.
int main () {
string x = "Hello World";
cout << x.size();
}
11
This marks the end of the Strings in C++ tutorial. Any suggestions or contributions for CodersLegacy are more than welcome. Questions regarding the article content can be asked in the comments section below.