In this tutorial, we will delve into the powerful combination of Pandas and Tkinter through the PandasTable library. PandasTable provides a convenient way to display and interact with pandas DataFrames in a Tkinter GUI.
Prerequisites:
- Basic knowledge of Python and Tkinter.
- Some Familiarity with Pandas and DataFrames.
You will also need to install the pandas and pandastable libraries. Run the following commands to do so:
pip install pandas
pip install pandastable
Creating Interactive Data Tables with PandasTable in Tkinter
Let’s start by importing the required libraries for our tutorial.
import tkinter as tk
from pandastable import Table, TableModel
import pandas as pd
Create a class for your application and initialize the main Tkinter window.
class PandasTableApp:
def __init__(self, root):
self.frame = tk.Frame(root)
self.frame.pack(padx=10, pady=10)
Next, we need to define a DataFrame with some sample data, which we will feature in our PandasTable.
# Create a sample DataFrame
data = {'Name': ['John', 'Alice', 'Bob'],
'Age': [28, 24, 22],
'City': ['New York', 'San Francisco', 'Los Angeles']}
df = pd.DataFrame(data)
Now, create a PandasTable widget and display the DataFrame within the Tkinter window. Remember to call the show function, as it is responsible for visually rendering (or updating) the PandasTable.
# Create a PandasTable
self.table = Table(self.frame, dataframe=df, showtoolbar=True, showstatusbar=True)
self.table.show()
Tip: Don’t ever put the root window as the PandasTable object’s parent (first parameter). You must use a container type object, such as a frame as the parent.
Complete the script with the Tkinter main loop.
if __name__ == "__main__":
root = tk.Tk()
app = PandasTableApp(root)
root.mainloop()
This gives us the following output:
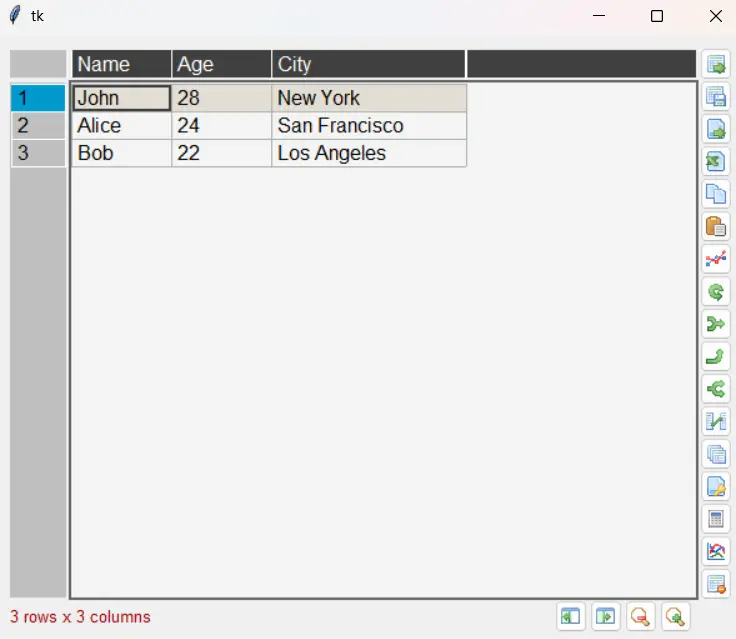
You can see the Toolbar on the right which comes with a whole bunch of cool features, such as saving the table and loading it. Various mutations you can apply, deleting rows, adding rows, zoom features, even some advanced plotting features, you name it!
Modifying the PandasTable directly
Most the functions are fairly intuitive, so there is little need to explain how to use them. Instead, there is another important aspect we will focus on explaining.
As a programmer, you need to know how to modify this table internally (through code). In the previous example, we initialized the table with a dataframe, but what if we want to make modifications (e.g. load another dataframe, or add a new record).
To do so, you can access the dataframe using the table.model.df
attributes. The following code defines a function which modifies the table by adding a new row:
import tkinter as tk
from pandastable import Table, TableModel
import pandas as pd
class PandasTableApp:
def __init__(self, root):
self.frame = tk.Frame(root)
self.frame.pack(padx=10, pady=10)
# Create a sample DataFrame
data = {'Name': ['John', 'Alice', 'Bob'],
'Age': [28, 24, 22],
'City': ['New York', 'San Francisco', 'Los Angeles']}
self.df = pd.DataFrame(data)
# Create a PandasTable
self.table = Table(self.frame, dataframe=self.df, showtoolbar=True, showstatusbar=True)
self.table.show()
self.modify_table()
def modify_table(self):
new_row = {'Name': 'Eve', 'Age': 25, 'City': 'Paris'}
self.df = self.df._append(new_row, ignore_index=True)
self.table.model.df = self.df
self.table.redraw()
if __name__ == "__main__":
root = tk.Tk()
app = PandasTableApp(root)
root.mainloop()
Note that we used the redraw
function after making the changes. This function is required for the changes to visually update.
As you can see, our new row has been added:
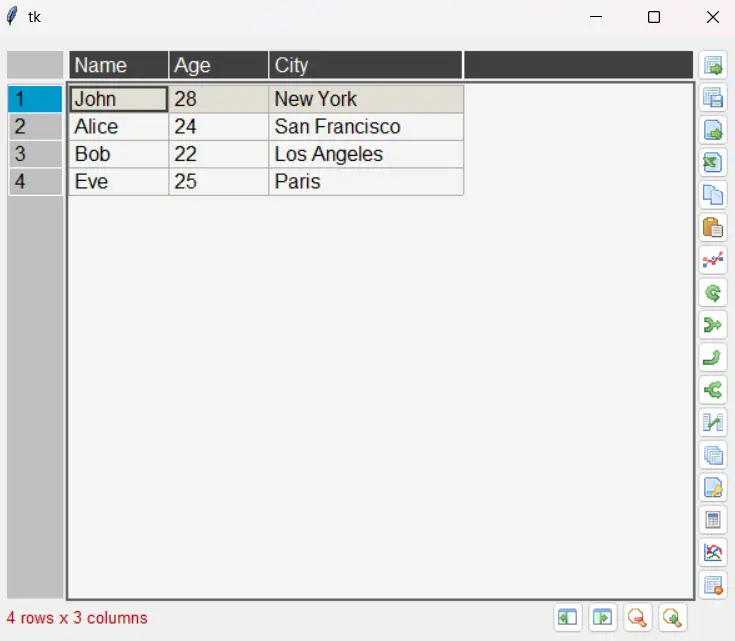
This marks the end of the Tkinter with PandasTable tutorial. Any suggestions or contributions for CodersLegacy are more than welcome. Questions regarding the tutorial content can be asked in the comments section below.