This article explains File Handling in C++.
File Handling in C++ refers to the process of creating, reading from, writing to and modifying files. In this tutorial we’ll cover each and every one of these major aspects of file handling.
Streams and Modes
All output and input in C++ is handled through the use of “streams” like the iostream
(input output stream) class which provides us with the cin
and cout
methods. Similarly, there is the fstream
(file stream) class which is used to handle writing to and from files.
There are three different streams that you may use.
Stream | Description |
---|---|
ofstream | Represents the output file stream, used to create and write data to files. |
ifstream | Represents the input file stream, used to read data from files. |
fstream | It represents the file stream itself. Can be used to read and write data from files. |
Below are the list of different input modes. These define how the files are opened and for what purpose (read/write/append).
Mode | Description |
---|---|
ios::in | The mode used for reading files |
ios::out | The mode used for writing files |
ios::app | Opens file in append mode. New data is written or “added” to the end of the file. |
ios::trunc | If the file (of the same name) exists, the data is truncated before opening it. |
ios::binary | Opens file in binary mode. |
Most of the time you will not have to do anything about this. The streams we discussed earlier use one or two modes by default so you don’t have to enter them manually. These default modes are shown below.
- ifstream
- ios::in
- ofstream
- ios::out
- fstream
- ios::in
- ios::out
Example
A short piece of code to demonstrate what we’ve learnt so far.
ofstream file;
file.open("file.txt", ios::out | ios::trunc );
First we created a stream object using the ofstream class (we want to write to a file). Next we used the open()
method which takes two parameters, a name and file mode. You can enter multiple file modes by separating them with a |
character.
With the below configuration, you can open a file for both input and output. (By using fstream, we don’t actually have to specify the input modes, we can leave it to the default value)
fstream file;
file.open("file.txt", ios::out | ios::in );
C++ File Handling Examples
We will now explain (with examples) how to read and write data to and from text files. We will start off by writing some data to a file, saving it and then reading the same data from it and printing it out.
Writing to a File
Remember to include the fstream
import.
int main()
{
// Create output file stream and data variable
ofstream stream;
string data;
// Open stream (default output mode)
stream.open("C://Users/Shado/Desktop/sample.txt");
// Text to be inserted
data = "This is some random data, \n to be inserted into the file";
// Inserting Data
stream << data;
// Close stream
stream.close();
return 0;
}
A screen shot from the file that we just created.
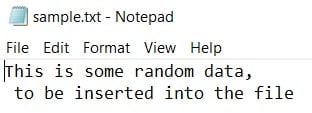
Reading from a file
There are many different ways you can read data from files in C++. We’ll cover two approaches here. In the first the data is read line by line. In the second, it is read word by word.
This is the line by line approach. The getline()
takes two parameters, the stream from which data is to be retrieved, and the variable to insert it into. At the name implies, it retrieves the data “line by line”.
#include <iostream>
#include <string>
#include <fstream>
using namespace std;
int main()
{
// Create input stream and data variable.
ifstream stream;
string line;
// Open stream with default input mode
stream.open("C://Users/CodersLegacy/Desktop/sample.txt");
while (stream) {
// Read a line from File
getline(stream, line);
// Print line
cout << line << endl;
}
stream.close();
return 0;
}
This is some random data,
to be inserted into the file
This approach is easier to manage than the word by word approach and requires lesser effort to make it presentable.
This is the word by word approach. It’s necessary to add a space using " "
else all the words would appear combined together.
#include <iostream>
#include <string>
#include <fstream>
using namespace std;
int main()
{
// Create input stream and data variable.
ifstream stream;
string data, x;
// Open stream with default input mode
stream.open("C://Users/CodersLegacy/Desktop/sample.txt");
while (stream >> x) {
data = data + x + " ";
}
cout << data;
stream.close();
return 0;
}
This is some random data, to be inserted into the file
Word by word approach is useful when you want analyse each word in the file individually.
This marks the end of the C++ File handling article. Any suggestions or contributions for CodersLegacy are more than welcome. Questions regarding the article content can be asked in the comments section below.