Often we find ourselves wanting to place some widgets inside the Tkinter Canvas. We can do this easily by just specifying the “canvas” object as the parent. However, it is often a better idea to create a Frame inside the Tkinter Canvas, and then place the widgets inside the Frame.
One benefit to using frames inside a canvas, is that if content of the frame is too much, then the Canvas will automatically make overflowing part “scrollable” (if you have added a scrollbar widget).
Placing a Frame inside Canvas (Example#1)
Let’s go through the process step-by-step.
First we will create our Tkinter application.
import tkinter as tk
root = tk.Tk()
Next we will create a simple Canvas and give it a little color as well. We didn’t add a scrollable region to the Canvas (yet), and just gave it a fixed size for now.
canvas = tk.Canvas(root, height=200, width=300,
background="lightblue")
canvas.pack()
Next we create a frame and give it the color “red” (to differentiate it from the Canvas). Remember to make the frame a child of the Canvas widget.
# Create a frame
frame = tk.Frame(canvas, bg="red")
No need to use any layout manager (like pack) on this Frame. The Canvas will handle that automatically.
To add this newly created frame to our window, we will call the create_window()
method on our Canvas object.
# Add the frame to the canvas
canvas.create_window(50, 50, window=frame, anchor="nw")
The first two parameters are the starting X
and Y
positions of the Frame (its top-left corner). The third parameter is where we pass the frame object we wish to add. The anchor
parameter makes the top-left corner of the frame the “anchor point” (nw
for north west).
Here is the full code.
import tkinter as tk
root = tk.Tk()
# Create a canvas
canvas = tk.Canvas(root, height=200, width=300, background="lightblue")
canvas.pack()
# Create a frame
frame = tk.Frame(root, bg="red")
# Add the frame to the canvas
canvas.create_window(50, 50, window=frame, anchor="nw")
# Add a button to the frame
button = tk.Button(frame, text="Click me!")
button.pack(padx = 20, pady = 20)
root.mainloop()
And here is the output.
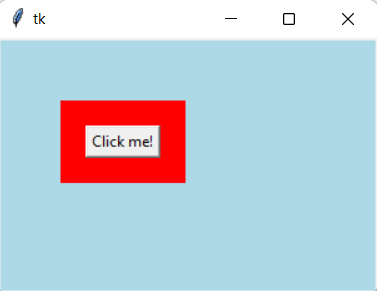
Example# 2
Let’s take a look at another example where we have added a scrolling feature into our Frame. The comments should be fairly self-explanatory. There are no new functions begin used here, so you shouldn’t have any trouble following code.
import tkinter as tk
root = tk.Tk()
# Create a Scrollbar
vbar = tk.Scrollbar(root, orient = tk.VERTICAL)
vbar.pack(side = tk.RIGHT, fill = tk.Y)
# Create a Canvas
canvas = tk.Canvas(root,
height=200,
width=300,
background="lightblue",
scrollregion=(0,0,500,500))
canvas.pack(expand=True, fill=tk.BOTH)
# Configure Canvas and ScrollBar
vbar.config(command = canvas.yview)
canvas.create_window(0, 0, window=frame1, anchor="nw")
canvas.config(yscrollcommand=vbar.set)
# Create wrapper frame
frame1 = tk.Frame(canvas, bg="blue")
# Create Frame 2
frame2 = tk.Frame(frame1, bg="red")
for i in range(5):
button = tk.Button(frame2, text="Click me!")
button.pack(padx = 20, pady = 20)
frame2.pack(padx = 5, pady = 5)
# Create Frame 3
frame3 = tk.Frame(frame1, bg="green")
button = tk.Button(frame3, text="Click me!")
button.pack(padx = 20, pady = 20)
frame3.pack(padx = 5, pady = 5)
root.mainloop()
The above code also shows how you can created nested frames inside a Canvas. Shown below is the output.
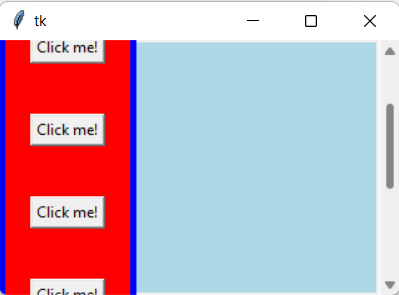
If you are interested in another example with a Frame whose contents can be scrolled, refer to this tutorial on Scrollable Frames. We also explored the concept of Scrollable Frames in greater detail there (and how we can resize them along with the Canvas)
This marks the end of the Create a Frame inside a Canvas in Tkinter Tutorial. Any suggestions or contributions for CodersLegacy are more than welcome. Questions about the tutorial content can be asked in the comments section below.