This tutorial is about various Data types in Java and how to use them
If you want to master Java, or even grasp it’s core concepts well, you need to understand the foundation. You need to be aware of the limitations, sizes and quirks of each data type. Incorrect handling of Data types may go unnoticed for a while, but will cause problems later on.
Data types in Java are split into two categories, Primitive and Non-Primitive. Primitive data types include Boolean, Byte, int, char, long, double, float and short. Non-primitive data types include String, Arrays and Classes.
Due to the complexity of classes, strings and Arrays as data types, they are discussed separately in the Strings, Arrays and classes sections respectively.
Primitive Data Types:
A list of all primitive data types with their descriptions.
Type | Meaning |
---|---|
Boolean | Holds True and False values |
byte | stores integers of size 8 bit |
char | Stores a character |
double | Double precision floating point |
float | Single precision floating point |
int | Stores a integer |
long | Stores long integer |
short | Stores a short integer |
Boolean
The Boolean data type, has only two possible values. true, or false. Used often in Logic gates or logical operations.
boolean a;
a = true;
System.out.println(a);
a = false;
System.out.println(a);
char
The char data type is a 16 bit type having a range of 0 to 65,535. Java uses Unicode, hence the large range. Since ASCII is a subset of Unicode, Java char data type and ASCII are compatible. (ASCII range is from 0 to 127). The purpose of using Unicode is to ensure portability across all systems.
float
There are two kinds of data types to represent decimals values in Java. The only difference between them is the range of values they support. The first is float, which is a 32 bit data type, and the second is double, which is a 64 bit data type. double is more often used type both by programmers and Java’s functions itself.
Integers
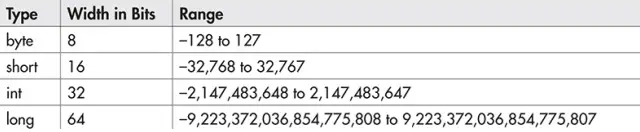
In the above image you can all the different data types used to store numerical values in Java.
With int being the most commonly used, long is used in situations where very large numerical values need to be stored. Similarly, the short integer is used where only small values are required.
The larger the data type, the more memory it consumes. Hence pick carefully according to the situation at hand. Below are some situations laid out for three integer types.
short a;
int b;
long c;
a = 40 * 10
b = 40 * 40 * 40
c = 40 ^ 10
Java Type Promotion in Expressions
When an expression is being evaluated, you are bound to find several different data types interacting with each other. This can cause issues due to each data type having different limitations imposed on it. Hence, Java has a system called type promotion which it uses to “promote” all the data types to one same data type. The expression is then evaluated.
Keep in mind, promotion only works on compatible data types. for instance, Strings and int will never be compatible. On the other hand, short and long are compatible since they both hold numerical values.
Java’s type promotion rules.
First, all char, byte, and short values are promoted to int. Then, if one operand is a long, the whole expression is promoted to long. If one operand is a float operand, the entire expression is promoted to float. If any of the operands is double, the result is double.
It is important to understand that type promotions apply only to the values operated upon when an expression is evaluated. For example, if the value of a byte variable is promoted to int inside an expression, outside the expression, the variable is still a byte. Type promotion only affects the evaluation of an expression.
Due to their close relationship, you should also check out the Java Operators Article.
This marks the end of our Java Data Types article. Any suggestions or contributions for CodersLegacy are more than welcome. Any questions can be asked below in the comments.